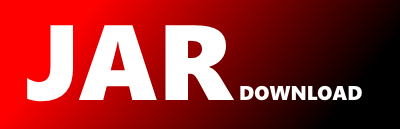
com.ats.tools.report.analytics.TestsResultBarChart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.analytics;
import java.awt.Color;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.text.DecimalFormat;
import java.util.Base64;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.ChartUtils;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.labels.StandardCategoryItemLabelGenerator;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.renderer.category.GroupedStackedBarRenderer;
import org.jfree.chart.renderer.category.StandardBarPainter;
import org.jfree.data.category.CategoryDataset;
import org.jfree.data.category.DefaultCategoryDataset;
public class TestsResultBarChart {
private static final Color FAIL_COLOR = Color.decode("#35535E");
private static final Color PASS_COLOR = Color.decode("#9CBF3F");
private static final Color SKIP_COLOR = Color.decode("#ECF0DF");
private DefaultCategoryDataset dataSet;
public TestsResultBarChart(int pass, int fail, int skip) {
dataSet = new DefaultCategoryDataset();
dataSet.addValue(pass, "passed", "0");
dataSet.addValue(fail, "failed", "0");
dataSet.addValue(skip, "filtered", "0");
}
@SuppressWarnings("serial")
private static StandardCategoryItemLabelGenerator labelGen = new StandardCategoryItemLabelGenerator("{0} ({2})", new DecimalFormat("0"), new DecimalFormat("0%")) {
@Override
public String generateLabel(CategoryDataset dataset, int row, int column) {
if(dataset.getValue(row, column).intValue() == 0) {
return null;
}
return super.generateLabel(dataset, row, column);
}
};
public String getBase64() {
final JFreeChart chart = ChartFactory.createStackedBarChart(
null, // chart title
null, // domain axis label
null, // range axis label
dataSet, // data
PlotOrientation.HORIZONTAL, // the plot orientation
false, // legend
false, // tooltips
false // urls
);
GroupedStackedBarRenderer renderer = new GroupedStackedBarRenderer();
renderer.setBarPainter( new StandardBarPainter() );
renderer.setRenderAsPercentages(true);
renderer.setDefaultItemLabelGenerator(labelGen);
renderer.setDefaultItemLabelsVisible(true);
renderer.setSeriesPaint(0, PASS_COLOR);
renderer.setSeriesPaint(1, FAIL_COLOR);
renderer.setSeriesPaint(2, SKIP_COLOR);
renderer.setSeriesItemLabelPaint(0, Color.WHITE);
renderer.setSeriesItemLabelPaint(1, Color.WHITE);
renderer.setSeriesItemLabelPaint(2, FAIL_COLOR);
CategoryPlot plot = (CategoryPlot) chart.getPlot();
plot.setRenderer(renderer);
plot.setFixedLegendItems(null);
chart.setBackgroundPaint(new Color(0xFF, 0xFF, 0xFF, 0));
plot .setBackgroundPaint(new Color(0xFF, 0xFF, 0xFF, 0));
plot.getDomainAxis().setVisible(false);
plot.getDomainAxis().setTickLabelsVisible(false);
plot.getRangeAxis().setVisible(false);
plot.getRangeAxis().setTickLabelsVisible(false);
plot.setOutlinePaint(null);
chart.getCategoryPlot().setRangeGridlinesVisible(false);
chart.setBorderVisible(false);
final ChartPanel chartPanel = new ChartPanel(chart);
chartPanel.setPreferredSize(new java.awt.Dimension(600, 36));
ByteArrayOutputStream baos = new ByteArrayOutputStream();
try {
ChartUtils.writeChartAsPNG(baos, chart, 600, 36);
} catch (IOException e) {
return null;
}
return Base64.getEncoder().encodeToString(baos.toByteArray());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy