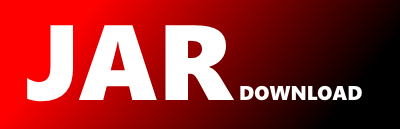
com.ats.tools.report.general.HtmlReportTestInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.general;
import com.ats.recorder.TestError;
import com.ats.tools.Utils;
import com.ats.tools.report.models.Summary;
import com.ats.tools.report.models.TestInfo;
import com.ats.tools.report.utils.HtmlActionUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.text.StringEscapeUtils;
import org.jetbrains.annotations.NotNull;
import java.io.File;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
public class HtmlReportTestInfo {
private static final String TABLE_VALUE_TEMPLATE = "${valuePlaceholder}";
private static final String TABLE_NO_VALUE_TEMPLATE = "${valuePlaceholder}";
private static final String TEST_NAME = "${testName}";
private static final String ACTIONS_COUNT = "${actionsCount}";
private static final String EXECUTION_TIME_MIL = "${executionTime}";
private static final String EXECUTION_TIME_SEC = "${executionTimeSec}";
private static final String EXECUTION_TIME_MIN = "${executionTimeMin}";
private static final String EXECUTION_TIME_HRS = "${executionTimeHrs}";
private static final String ID_HIDDEN_PLACEHOLDER_MIN = "${idHiddenPlaceHolderMin}";
private static final String ID_HIDDEN_PLACEHOLDER_SEC = "${idHiddenPlaceHolderSec}";
private static final String ID_HIDDEN_PLACEHOLDER_MIL = "${idHiddenPlaceHolderMil}";
private static final String ID_HIDDEN_PLACEHOLDER_HRS = "${idHiddenPlaceHolderHrs}";
private static final String ERROR_TEXT_COLOR_PLACEHOLDER = "${error-text-color-placeholder}";
private static final String EXECUTION_ERROR = "${executionError}";
private static final String ERROR_ACTION_URL = "${errorActionUrl}";
private static final String ERROR_ACTION_ID = "${errorActionId}";
private static final String REPORT_SUMMARY = "${reportSummary}";
private static final String AUTHOR = "${author}";
private static final String STARTED = "${started}";
private static final String FINISHED = "${finished}";
private static final String TEST_ID = "${testId}";
private static final String EXTERNAL_ID = "${externalId}";
private static final String TEST_DESCRIPTION = "${testDescription}";
private static final String PREREQUISITES = "${prerequisites}";
private static final String GROUPS = "${groups}";
public static final String EXECUTE_BUTTON_TEMPLATE = "${executeButton}";
public static final String EXECUTE_BUTTON_HTML_TEMPLATE = "${buttonLabel}";
private static final String TEST_CASE_ID = "${testCaseId}";
private static final String TEST_TITLE = "${testTitle}";
private static final String TEST_EXECUTION_STATUS_NAME_CLASS = "test-execution-status-name";
private static final String BLOCKING_ERROR_LABEL_PLACEHOLDER = "${blocking-error-label}";
private static final String EXECUTION_ERROR_TEMPLATE = "Line: ${executionErrorLine}, Error: ${executionErrorText}";
public static final String GROUP_NAME_HTML_TEMPLATE = "${groupName}";
public static final String EXTERNAL_LINK_HTML_TEMPLATE = "${externalResource}";
public static final String EXECUTION_ERROR_HTML_TEMPLATE = "\n" +
" Execution error\n" +
" ${executionError}${blocking-error-label}" +
" ";
public static final String REPORT_SUMMARY_HTML_TEMPLATE = "\n" +
" Report summary\n" +
" " +
" \n" +
" \n" +
" ";
public static final String TEST_NAME_HTML_TEMPLATE = "${testName}";
private static final String REPORT_HTML_LINK_PLACE_HOLDER = "${htmlReportLink}";
public static final String REPORT_EXECUTION_RESULT_HTML_TEMPLATE = "\n";
public static final String REPORT_EXECUTION_RESULT_HTML_TEMPLATE_PLACE_HOLDER = "${executionResulPlaceholder}";
public static final String EXECUTION_ERROR_HTML_PLACEHOLDER = "${executionErrorTemplate}";
public static final String EXECUTION_SUMMARY_HTML_PLACEHOLDER = "${executionSummaryTemplate}";
public static final String ACTION_TYPE_CHART = "${actionTypeChart}";
private String template;
private String result;
public HtmlReportTestInfo(TestInfo testInfo, String template, String htmlReportPath, String suiteName) {
String groups = testInfo.getGroups().stream().filter(group -> !StringUtils.isEmpty(group)).toList().isEmpty() ?
"" : testInfo.getGroups().stream().map(group -> buildGroupNameWithLink(testInfo, group))
.collect(Collectors.joining());
this.result = template.replace(AUTHOR, formatValue(testInfo.getAuthor()));
this.result = result.replace(STARTED, formatValue(testInfo.getStartedFormatted()));
this.result = result.replace(FINISHED, formatValue(testInfo.getFinishedFormated()));
this.result = result.replace(ACTION_TYPE_CHART, testInfo.getActionTypeChart());
this.result = result.replace(TEST_ID, formatValue(testInfo.getTestId()));
this.result = result.replace(EXTERNAL_ID, formatValue(testInfo.getExternalId()));
this.result = result.replace(TEST_DESCRIPTION, formatValue(testInfo.getDescription()));
this.result = result.replace(PREREQUISITES, formatValue(testInfo.getPrerequisite()));
this.result = result.replace(GROUPS, formatValue(groups));
this.result = result.replace(TEST_NAME, buildTestNameLink(testInfo));
this.result = StringUtils.replace(result, TEST_CASE_ID, testInfo.getTestName() + " " + suiteName);
this.result = result.replace(REPORT_HTML_LINK_PLACE_HOLDER, buildReportsLinks(htmlReportPath, testInfo.getTestName(), suiteName));
this.result = result.replace(EXECUTE_BUTTON_TEMPLATE, buildExecuteButton(testInfo, suiteName));
this.result = result.replace(TEST_TITLE, testInfo.getTestName());
if (testInfo.getSummary() != null) {
this.result = result.replace(ACTIONS_COUNT, testInfo.getSummary().getActions());
if (Long.parseLong(testInfo.getSummary().getDuration()) < 1000) {
this.result = result.replace(EXECUTION_TIME_MIL, Long.parseLong(testInfo.getSummary().getDuration()) % 1000 + " ms");
this.result = result.replace(ID_HIDDEN_PLACEHOLDER_MIN, "hidden");
this.result = result.replace(ID_HIDDEN_PLACEHOLDER_SEC, "hidden");
this.result = result.replace(ID_HIDDEN_PLACEHOLDER_HRS, "hidden");
} else {
this.result = result.replace(ID_HIDDEN_PLACEHOLDER_MIL, "hidden");
this.result = result.replace(EXECUTION_TIME_SEC, Utils.getSecondsForTimeline(Long.parseLong(testInfo.getSummary().getDuration())) + " sec");
this.result = result.replace(EXECUTION_TIME_MIN, Utils.getMinutesForTimeLine(Long.parseLong(testInfo.getSummary().getDuration())) + " min");
if (Long.parseLong(testInfo.getSummary().getDuration()) > 3600000) {
this.result = result.replace(EXECUTION_TIME_HRS, Long.parseLong(testInfo.getSummary().getDuration()) / 3600000 + " hr");
} else {
this.result = result.replace(ID_HIDDEN_PLACEHOLDER_HRS, "hidden");
}
}
String formattedExecutionResult = "";
if (testInfo.getSummary().getStatus().equals("1")) {
formattedExecutionResult = REPORT_EXECUTION_RESULT_HTML_TEMPLATE.replace("${executionResult}", "result-pass-icon");
} else {
formattedExecutionResult = REPORT_EXECUTION_RESULT_HTML_TEMPLATE.replace("${executionResult}", "result-failed-icon");
}
this.result = result.replace(REPORT_EXECUTION_RESULT_HTML_TEMPLATE_PLACE_HOLDER, formattedExecutionResult);
if (StringUtils.isNoneEmpty(testInfo.getSummary().getData())) {
this.result = result.replace(EXECUTION_SUMMARY_HTML_PLACEHOLDER,
REPORT_SUMMARY_HTML_TEMPLATE.replace(REPORT_SUMMARY, testInfo.getSummary().getData().replaceAll("'", "'")));
} else {
this.result = result.replace(EXECUTION_SUMMARY_HTML_PLACEHOLDER, "");
}
if (testInfo.getSummary().getTestErrors() != null && !testInfo.getSummary().getTestErrors().isEmpty()) {
String testErrors = testInfo.getSummary().getTestErrors().stream().map((TestError testError) -> buildExecutionErrorElement(testError, testInfo)).collect(Collectors.joining());
this.result = result.replace(EXECUTION_ERROR_HTML_PLACEHOLDER, testErrors);
this.result = result.replace(ERROR_ACTION_URL, HtmlActionUtils.getActionUrl(testInfo, testInfo.getSummary().getErrorScriptName(), testInfo.getSummary().getErrorLine()));
} else {
this.result = result.replace(EXECUTION_ERROR_HTML_PLACEHOLDER, "");
}
} else {
this.result = result.replace(EXECUTION_SUMMARY_HTML_PLACEHOLDER, "");
this.result = result.replace(EXECUTION_ERROR_HTML_PLACEHOLDER, "");
this.result = result.replace(ACTIONS_COUNT, "No value");
this.result = result.replace(EXECUTION_TIME_MIL, "No value");
this.result = result.replace(REPORT_EXECUTION_RESULT_HTML_TEMPLATE_PLACE_HOLDER, "No value");
}
}
@NotNull
private String buildExecutionErrorElement(TestError testError, TestInfo testInfo) {
String result = StringUtils.replace(EXECUTION_ERROR_HTML_TEMPLATE, EXECUTION_ERROR, buildExecutionErrorLine(testError));
result = TestError.TestErrorStatus.FAIL_STOP.equals(testError.getTestErrorStatus()) ? result.replace(BLOCKING_ERROR_LABEL_PLACEHOLDER, "Blocking-error") : result.replace(BLOCKING_ERROR_LABEL_PLACEHOLDER, "");
result = result.replace(ERROR_ACTION_ID, testInfo.getTestName() + ":" + testInfo.getSummary().getTestErrors().indexOf(testError));
result = result.replace(ERROR_ACTION_URL, HtmlActionUtils.getActionUrl(testInfo, testError.getScript(), String.valueOf(testError.getLine())));
result = result.replace(ERROR_TEXT_COLOR_PLACEHOLDER, TestError.TestErrorStatus.FAIL_PASS.equals(testError.getTestErrorStatus()) ? "error-text-brown" : "error-text-red");
return TestError.TestErrorStatus.FAIL_PASS.equals(testError.getTestErrorStatus()) ? result.replace(TEST_EXECUTION_STATUS_NAME_CLASS, TEST_EXECUTION_STATUS_NAME_CLASS + " soft-orange-background-color") : result;
}
@NotNull
private static String buildReportsLinks(String htmlReportLink, String testName, String suiteName) {
String atsvLink = EXTERNAL_LINK_HTML_TEMPLATE.replace("${externalResourceUri}", suiteName + File.separator + testName + ".atsv").replace("${externalResource}", "ATSV");
String atsvHtmlLink = EXTERNAL_LINK_HTML_TEMPLATE.replace("${externalResourceUri}", suiteName + File.separator + testName + "_atsv.html").replace("${externalResource}", "ATSV_HTML");
if (htmlReportLink != null) {
String path = suiteName + File.separator + testName + "_xml" + File.separator + "ats-test-report.html";
htmlReportLink = EXTERNAL_LINK_HTML_TEMPLATE.replace("${externalResourceUri}", path).replace("${externalResource}", "HTML");
} else {
htmlReportLink = "";
}
return atsvLink + atsvHtmlLink + htmlReportLink;
}
@NotNull
private static String buildGroupNameWithLink(TestInfo testInfo, String group) {
return GROUP_NAME_HTML_TEMPLATE.replace("${groupUri}", testInfo.getProject().getProjectId() + "/group/" + group).replace("${groupName}", group);
}
private String buildTestNameLink(TestInfo testInfo) {
return TEST_NAME_HTML_TEMPLATE.replace("${testNameUri}", testInfo.getProject().getProjectId() + "/script/" + testInfo.getTestName()).replace("${testName}", testInfo.getTestName()).replace("${testNameTitle}", testInfo.getTestName());
}
private String buildExecutionErrorLine(TestError testError) {
return EXECUTION_ERROR_TEMPLATE
.replace("${executionErrorLine}", String.valueOf(testError.getLine()))
.replace("${executionErrorText}", testError.getMessage());
}
private String formatValue(String value) {
return StringUtils.isEmpty(value) ?
TABLE_NO_VALUE_TEMPLATE.replace("${valuePlaceholder}", "No value") :
TABLE_VALUE_TEMPLATE.replace("${valuePlaceholder}", value);
}
private String buildExecuteButton(TestInfo testInfo, String suiteName) {
return EXECUTE_BUTTON_HTML_TEMPLATE.replace("${scriptNameUri}", testInfo.getProject().getProjectId() + "/run-script/" + testInfo.getTestName() + "/" + suiteName).replace("${buttonLabel}", "Execute");
}
public String getResult() {
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy