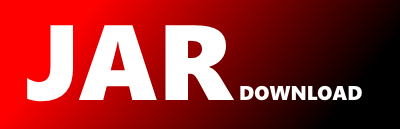
com.ats.tools.report.models.TestInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.models;
import com.ats.tools.Utils;
import java.time.Instant;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.List;
public class TestInfo {
private String cpuCount;
private String cpuSpeed;
private String externalId;
private String osInfo;
private String testId;
private String testName;
private String totalMemory;
private String description;
private String author;
private String prerequisite;
private String started;
private String startedFormatted;
private String quality;
private String durationChart;
private String actionTypeChart;
private List groups = new ArrayList<>();
private Project project;
private Summary summary;
private String defaultActionTypeChart = "iVBORw0KGgoAAAANSUhEUgAAANsAAABQCAYAAACQ9ABbAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAD7SURBVHhe7dOxAYAwDMCw0P9/Boa+EE/S4gv8vL8B1p1bYJnZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjaImA0iZoOI2SBiNoiYDSJmg4jZIGI2iJgNImaDiNkgYjZIzHzp4AScK7RsBQAAAABJRU5ErkJggg==";
@Override
public String toString() {
return "TestRecord{" +
"cpuCount=" + cpuCount +
", cpuSpeed=" + cpuSpeed +
", externalId='" + externalId + '\'' +
", osInfo='" + osInfo + '\'' +
", testId='" + testId + '\'' +
", testName='" + testName + '\'' +
", totalMemory=" + totalMemory +
", description='" + description + '\'' +
", author='" + author + '\'' +
", prerequisite='" + prerequisite + '\'' +
", started=" + started +
", startedFormatted='" + startedFormatted + '\'' +
", quality=" + quality +
", groups=" + groups +
", project=" + project +
", summary=" + summary +
'}';
}
public String getCpuCount() {
return cpuCount;
}
public void setCpuCount(String cpuCount) {
this.cpuCount = cpuCount;
}
public String getCpuSpeed() {
return cpuSpeed;
}
public void setCpuSpeed(String cpuSpeed) {
this.cpuSpeed = cpuSpeed;
}
public String getExternalId() {
return externalId;
}
public void setExternalId(String externalId) {
this.externalId = externalId;
}
public String getOsInfo() {
return osInfo;
}
public void setOsInfo(String osInfo) {
this.osInfo = osInfo;
}
public String getTestId() {
return testId;
}
public void setTestId(String testId) {
this.testId = testId;
}
public String getTestName() {
return testName;
}
public void setTestName(String testName) {
this.testName = testName;
}
public String getTotalMemory() {
return totalMemory;
}
public void setTotalMemory(String totalMemory) {
this.totalMemory = totalMemory;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPrerequisite() {
return prerequisite;
}
public void setPrerequisite(String prerequisite) {
this.prerequisite = prerequisite;
}
public String getStarted() {
return started;
}
public void setStarted(String started) {
this.started = started;
}
public String getStartedFormatted() {
return startedFormatted;
}
public void setStartedFormatted(String startedFormatted) {
this.startedFormatted = startedFormatted;
}
public String getQuality() {
return quality;
}
public void setQuality(String quality) {
this.quality = quality;
}
public List getGroups() {
return groups;
}
public void setGroups(List groups) {
this.groups = groups;
}
public Project getProject() {
return project;
}
public void setProject(Project project) {
this.project = project;
}
public Summary getSummary() {
return summary;
}
public void setSummary(Summary summary) {
this.summary = summary;
}
public String getDurationChart() {
return durationChart;
}
public void setDurationChart(String durationChart) {
this.durationChart = durationChart;
}
public String getActionTypeChart() {
return actionTypeChart == null ? defaultActionTypeChart : actionTypeChart;
}
public void setActionTypeChart(String actionTypeChart) {
this.actionTypeChart = actionTypeChart;
}
public String getFinishedFormated() {
return OffsetDateTime.ofInstant(Instant.ofEpochMilli(Utils.string2Long(getStarted()) + Utils.string2Long(summary.getDuration())),
ZoneId.systemDefault()).format(DateTimeFormatter.ofPattern("yyyy-MM-dd 'at' HH:mm:ss"));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy