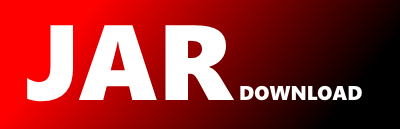
com.ats.tools.report.utils.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.utils;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Base64;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.jfree.data.json.impl.JSONArray;
import org.jfree.data.json.impl.JSONObject;
import com.ats.tools.ResourceContent;
import com.ats.tools.logger.levels.AtsLogger;
import com.ats.tools.report.models.Project;
import com.ats.tools.report.models.Script;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* Custom file utils class.
*/
public class FileUtils {
private static final String jpegTypeHex = "FFFFFFFFFFFFFFD8FFFFFFFFFFFFFF";
private static final String pngTypeHex = "FFFFFF89504E47";
private static final String mpegTypeHex = "00018";
/**
* Allows to check file type of Base64 data, work with png or jpeg files.
*
* @param base64Data
* @return either "jpeg" or "png" file types.
*/
public static String getFileType(String base64Data) {
String dataTypeInHex = "";
byte[] decode = Base64.getDecoder().decode(base64Data);
for (int i = 0; i <= 3; i++) {
dataTypeInHex += Integer.toHexString(decode[i]).toUpperCase();
}
if (dataTypeInHex.startsWith(jpegTypeHex)) {
return "jpeg";
} else if (dataTypeInHex.startsWith(pngTypeHex)) {
return "png";
} else if (dataTypeInHex.startsWith(mpegTypeHex)) {
return "mpeg";
} else {
throw new RuntimeException("Wrong file format detected.");
}
}
public static List listFiles(Path path, String extension) throws IOException {
List result;
try (Stream walk = Files.walk(path)) {
result = walk
.filter(Files::isRegularFile)
.filter(p -> p.getFileName().toString().endsWith(extension))
.collect(Collectors.toList());
}
return result;
}
/**
* Deletes the folder. Recursively deletes all the files in the folder, and then deletes the folder itself.
*
* @param folder folder to be deleted.
*/
public static void deleteFolder(File folder) {
if (folder.isDirectory()) {
File[] files = folder.listFiles();
if (files != null) {
for (File file : files) {
deleteFolder(file);
}
}
}
if (!folder.delete()) {
AtsLogger.printLog("failed to delete: " + folder.getPath());
}
}
public static void clearTempDir(String agilitestResourcesPrefix) {
Path tempDirectoryPath = Path.of(System.getProperty("java.io.tmpdir"));
File[] files = tempDirectoryPath.toFile().listFiles();
if (files != null) {
Arrays.stream(files).filter(file -> file.getPath().contains(agilitestResourcesPrefix)).forEach(FileUtils::deleteFolder);
}
}
public static File copyResource(String resName, Path dest) {
String customTemplatesPath = "/reports/templates/";
Path filePath = dest.resolve(resName);
if (!Files.exists(filePath)) {
InputStream is = ResourceContent.class.getResourceAsStream(customTemplatesPath + resName);
InputStream in = new BufferedInputStream(is);
File targetFile = dest.resolve(resName).toFile();
try {
OutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile));
byte[] buffer = new byte[1024];
int lengthRead;
while ((lengthRead = in.read(buffer)) > 0) {
out.write(buffer, 0, lengthRead);
out.flush();
}
in.close();
is.close();
out.close();
} catch (IOException e) {
AtsLogger.printLog("not able to copy resource...");
}
}
return filePath.toFile();
}
public static String getBase64LogoProperty(Project project, String outputFolderPath) {
String logoPath = com.ats.script.Project.REPORT_LOGO_URL;
InputStream input = null;
try {
input = Thread.currentThread().getContextClassLoader().getResourceAsStream(logoPath);
} catch (Exception e) {}
if(input == null) {
try {
input = Thread.currentThread().getContextClassLoader().getResourceAsStream("src/" + logoPath);
} catch (Exception e) {
e.printStackTrace();
}
}
if(input != null) {
try {
return "data:image/png;base64," + new String(Base64.getEncoder().encode(input.readAllBytes()));
} catch (IOException e) {
AtsLogger.printLog("cannot get project's report logo data");
}
}
return project.getATS_LOGO();
}
public static List