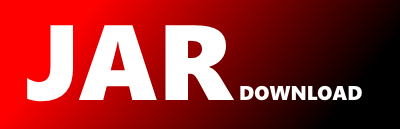
com.ats.tools.report.utils.ImageProcessingUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.utils;
import com.ats.tools.logger.levels.AtsLogger;
import org.w3c.dom.NodeList;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.*;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.stream.Collectors;
/**
* Class which provides functionality which processes the images.
*/
public class ImageProcessingUtils {
private static final int NUM_THREADS = Runtime.getRuntime().availableProcessors(); // Number of threads to use
/**
* Allows to extract image from Base64 "img" xml tag data. Uses tag attributes to determine file name and type.
* Generates default file name if there is no suitable attribute.
* Determines file type from Base64 content.
* Replaces Base64 content of "img" node with absolute path of extracted image.
*
* @param imgs List of image nodes.
* @param agilitestTempResourcesPath path to folder where images will be extracted to.
*/
public static void processImages(NodeList imgs, String agilitestTempResourcesPath) {
try (ExecutorService executor = Executors.newFixedThreadPool(NUM_THREADS)) {
for (int i = 0; i < imgs.getLength(); i++) {
if (imgs.item(i).getAttributes().getNamedItem("src") != null) {
String name;
String src = imgs.item(i).getAttributes().getNamedItem("src").getNodeValue();
String type = FileUtils.getFileType(src);
if (imgs.item(i).getAttributes().getNamedItem("id") == null) {
name = "screen_" + i;
} else {
name = imgs.item(i).getAttributes().getNamedItem("id").getNodeValue();
}
executor.submit(new ImageProcessor(src, name, agilitestTempResourcesPath, type));
if (!type.equals("mpeg")) {
imgs.item(i).getAttributes().getNamedItem("src").setNodeValue(agilitestTempResourcesPath + File.separator + name + "." + type);
}
imgs.item(i).getAttributes().getNamedItem("type").setNodeValue(type);
}
}
executor.shutdown();
}
}
public static void processVideos(String atsTestReportPath, File agilitestTempResourceFile, String agilitestTempResourcesPath) throws IOException {
List videosToBeProcessed = Arrays.stream(Objects.requireNonNull(agilitestTempResourceFile.listFiles()))
.filter(file -> file.getName().endsWith("mpeg"))
.map(file -> "videoPlaceholder" + file.getName().replace(".mpeg", ""))
.collect(Collectors.toList());
if (!videosToBeProcessed.isEmpty()) {
AtsLogger.printLog("processing "+ videosToBeProcessed.size() +" video file(s)");
replaceStringsInFile(atsTestReportPath, videosToBeProcessed, agilitestTempResourcesPath);
}
}
private static void replaceStringsInFile(String filePath, List oldStrings, String agilitestTempResourcesPath) {
File inputFile = new File(filePath);
File outputFile = new File(filePath + ".tmp");
try (InputStream inputStream = new FileInputStream(inputFile);
OutputStream outputStream = new FileOutputStream(outputFile)) {
Map replacementMap = new HashMap<>();
for (String oldString : oldStrings) {
String replacementString = Base64.getEncoder().encodeToString(Files.readAllBytes(Path.of(agilitestTempResourcesPath + File.separator + oldString.replace("videoPlaceholder", "") + ".mpeg")));
replacementMap.put(oldString, replacementString);
}
byte[] buffer = new byte[4096];
int bytesRead;
StringBuilder currentLine = new StringBuilder();
while ((bytesRead = inputStream.read(buffer)) != -1) {
for (int i = 0; i < bytesRead; i++) {
if (buffer[i] == '\n' || buffer[i] == '\r') {
processLine(currentLine.toString(), outputStream, replacementMap);
currentLine.setLength(0);
} else {
currentLine.append((char) buffer[i]);
}
}
}
processLine(currentLine.toString(), outputStream, replacementMap);
} catch (IOException | OutOfMemoryError e) {
e.printStackTrace();
System.exit(1);
}
if (inputFile.delete()) {
outputFile.renameTo(inputFile);
}
}
private static void processLine(String line, OutputStream outputStream, Map replacements) throws IOException {
for (Map.Entry entry : replacements.entrySet()) {
line = line.replace(entry.getKey(), entry.getValue());
}
outputStream.write(line.getBytes());
outputStream.write('\n');
}
/**
* Processes the images.
*/
private static class ImageProcessor implements Callable {
private final String src;
private final String name;
private final String tempResourcesPath;
private final String type;
public ImageProcessor(String src, String name, String tempResourcesPath, String type) {
this.src = src;
this.name = name;
this.tempResourcesPath = tempResourcesPath;
this.type = type;
}
@Override
public Void call() {
Base64.Decoder decoder = Base64.getDecoder();
byte[] decode = decoder.decode(src);
try (FileOutputStream fos = new FileOutputStream(tempResourcesPath + File.separator + name + "." + type)) {
fos.write(decode);
} catch (IOException e) {
AtsLogger.printLog("error saving file: " + e.getMessage());
}
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy