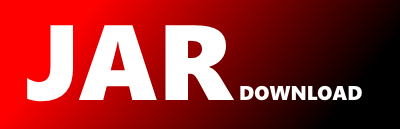
com.activitystream.model.relations.Relation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of as-sdk Show documentation
Show all versions of as-sdk Show documentation
AS-SDK is a java library to allow easy interoperability with Activity Stream.
package com.activitystream.model.relations;
import com.activitystream.model.*;
import com.activitystream.model.core.AbstractMapElement;
import com.activitystream.model.entities.BusinessEntity;
import com.activitystream.model.entities.EntityReference;
import com.activitystream.model.interfaces.*;
import com.activitystream.model.stream.AbstractBaseEvent;
import com.activitystream.model.stream.AbstractStreamItem;
import com.activitystream.model.stream.CustomerEvent;
import com.activitystream.model.stream.TransactionEvent;
import com.activitystream.model.validation.InvalidPropertyContentError;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import java.util.function.Consumer;
public class Relation extends AbstractMapElement implements EmbeddedStreamElement, EnrichableElement {
protected static final Logger logger = LoggerFactory.getLogger(Relation.class);
private final static List EXPANDED_ASPECTS =
Arrays.asList(ASConstants.ASPECTS_PRESENTATION, ASConstants.ASPECTS_CLIENT_DEVICE, ASConstants.ASPECTS_TIMED, ASConstants.ASPECTS_CLIENT_IP,
ASConstants.ASPECTS_TRAFFIC_SOURCE, ASConstants.ASPECTS_DIMENSIONS, ASConstants.ASPECTS_METRICS, ASConstants.ASPECTS_CLASSIFICATION,
ASConstants.ASPECTS_ADDRESS,
ASConstants.ASPECTS_DEMOGRAPHY, ASConstants.ASPECTS_INVENTORY);
private RelationsType relationsType = null;
private boolean isExpanded = false;
private Direction direction = null;
public Relation(Map relations, BaseStreamElement root) {
setRoot(root);
if (relations instanceof Relation) {
logger.error("Trying to create a relation with a relation 1 : " + relations);
} else {
setMappedRelations(relations);
}
}
public Relation(Object value, BaseStreamElement root) {
setRoot(root);
if (value instanceof Relation) {
logger.error("Trying to create a relation with a relation 2 : " + value);
} else if (value instanceof Map) {
setMappedRelations((Map) value);
} else if (value instanceof List) {
logger.warn("WTF - relations is a list: " + value);
} else {
logger.warn("WTF item information can not be: " + value);
addProblem(new InvalidPropertyContentError("ItemRelations information are incorrect: " + value));
}
verify();
}
public Relation(String type, Object value) {
this(type, value, null);
}
public Relation(String type, BusinessEntity entity) {
this(type, entity, null);
}
public Relation(String type, Object value, BaseStreamElement root) {
setRoot(root);
Map relationsMap = new LinkedHashMap<>();
relationsMap.put(type, value);
if (value instanceof Relation) {
logger.error("Trying to create a relation with a relation 3 : " + value);
} else if (value instanceof BusinessEntity) {
this.setRelationsType(RelationsType.resolveTypesString(type));
this.setRelatedItem((BusinessEntity) value);
} else if (value instanceof Map) {
this.setMappedRelations(relationsMap);
} else if (value instanceof String) {
this.setRelationsType(RelationsType.resolveTypesString(type));
this.setRelatedItem(entityForValue(value, root));
} else if (value instanceof EntityReference) {
this.setRelationsType(RelationsType.resolveTypesString(type));
this.setRelatedItem(entityForValue(value, root));
} else if (value instanceof List) {
logger.error("Unsupported list value for relations: " + value);
} else {
addProblem(new InvalidPropertyContentError("ItemRelations information are incorrect: " + value));
}
}
/************ Access ************/
public BaseStreamElement getRelatedEntityItem() {
return (this.isRelatedItemABusinessEntity()) ? (BusinessEntity) this.getRelatedItem() : null;
}
public BusinessEntity getRelatedBusinessEntity() {
return (BusinessEntity) getRelatedEntityItem();
}
public boolean isRelatedItemABusinessEntity() {
return this.getRelatedItem() != null && this.getRelatedItem() instanceof BusinessEntity;
}
public RelationsType getRelationsType() {
return this.relationsType;
}
public void setRelationsType(RelationsType relType) {
this.relationsType = relType;
}
public BaseStreamElement getRelatedItem() {
if (this.getRelationsType() != null && this.get(this.getRelationsType()) instanceof BaseStreamElement)
return (BaseStreamElement) get(this.getRelationsType());
return null;
}
public void setRelatedItem(BaseStreamElement relatedItem) {
if (this.getRelationsType() != null) put(this.getRelationsType(), relatedItem);
else logger.error("No Relationship Type specified before setting related entity!!!!");
}
public String getDirection() {
return (String) get("$direction");
}
@Override
public boolean equals(Object o) {
if (o instanceof Relation) {
return ((Relation) o).getRelationsType().getRelationsType().equals(this.getRelationsType().getRelationsType()) &&
((Relation) o).getRelatedItem().equals(this.getRelatedItem());
}
return super.equals(o);
}
@Override
public void simplify() {
BusinessEntity entity = getRelatedBusinessEntity();
if (entity != null) super.put(this.getRelationsType(), new BusinessEntity(entity.getEntityReference().getEntityReference(), this));
}
/************ Utility functions ************/
public boolean isValid() {
return true;
}
public boolean isOfType(String type) {
if (this.getRelationsType() == null) return false;
for (String relType : this.getRelationsType().getRelationsType().split(":")) {
if (relType.equalsIgnoreCase(type)) return true;
}
return false;
}
public boolean isVisible() {
BusinessEntity entity = getRelatedBusinessEntity();
if (entity != null)
return entity.getStatusAspect().isVisible();
return true;
}
/**
* Goes through the relation information and transforms it into valid relations.
* Takes the event relation and puts it first.
* Then it takes alternative relations and adds it to the related entity (if multiple reltions used)
*
* @param valueMap
*/
public void setMappedRelations(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy