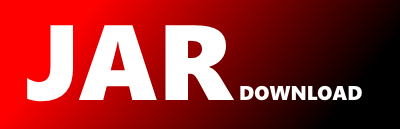
com.activitystream.model.aspects.AbstractMapAspect Maven / Gradle / Ivy
package com.activitystream.model.aspects;
import com.activitystream.model.ASConstants;
import com.activitystream.model.core.AbstractMapElement;
import com.activitystream.model.interfaces.AspectInterface;
import com.activitystream.model.interfaces.BaseStreamElement;
import com.activitystream.model.entities.BusinessEntity;
import com.activitystream.model.entities.EntityChangeMap;
import com.activitystream.model.entities.EntityReference;
import com.activitystream.model.validation.InvalidPropertyContentError;
import org.joda.time.DateTime;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
@SuppressWarnings("unchecked")
public abstract class AbstractMapAspect extends AbstractMapElement implements AspectInterface {
protected static final Logger logger = LoggerFactory.getLogger(AbstractMapAspect.class);
boolean autoGenerated = false;
public AbstractMapAspect() {
}
public AbstractMapAspect(Map values, BaseStreamElement root) {
super(values, root);
}
@Override
public void markAsAutoGenerated() {
this.autoGenerated = true;
}
@Override
public boolean isAutoGenerated() {
return this.autoGenerated;
}
@Override
public boolean isInherited() {
return containsKey(ASConstants.FIELD_INHERITED_VIA);
}
@Override
public void loadFromValue(Object value) {
if (value instanceof Map) {
setMapValues((Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy