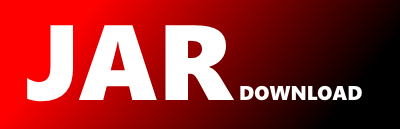
com.adaptrex.core.view.ConfigComponent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adaptrex-core Show documentation
Show all versions of adaptrex-core Show documentation
The Core Adaptrex Framework
The newest version!
/*
* Copyright 2012 Adaptrex, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.adaptrex.core.view;
import javax.servlet.http.HttpServletRequest;
import com.adaptrex.core.Adaptrex;
import com.adaptrex.core.AdaptrexProperties;
import com.adaptrex.core.utilities.StringUtilities;
/**
* The ConfigComponent is used to initialize Adaptrex on a webpage. It is used by
* the various available presentation framework components to set up an Ext namespace,
* create a basic context object for the page, and initialize other settings used by
* other Adaptrex components.
*/
public class ConfigComponent {
public static final String ATTRIBUTE = "com.adaptrex.configcomponent";
private HttpServletRequest request;
private AdaptrexProperties properties;
private boolean debug;
private String contextPath;
private String namespace;
private String weblibPath;
private String env;
private String sdkVersion;
private String theme;
private Boolean touch;
private boolean isWritten = false;
public ConfigComponent(HttpServletRequest request, String namespace, Boolean touch) {
this.request = request;
this.contextPath = request.getContextPath();
properties = Adaptrex.get(request.getServletContext()).getProperties();
this.namespace = namespace;
this.touch = touch;
if (this.touch == null)
this.touch = properties.get(AdaptrexProperties.SENCHATOUCH, "false").equals("true");
this.debug = properties.get(AdaptrexProperties.DEBUG, "false").equals("true");
this.weblibPath = properties.get(AdaptrexProperties.WEBLIB_PATH, "weblib");
this.env = properties.get(AdaptrexProperties.ENVIRONMENT, "production");
this.sdkVersion = properties.get(
this.touch ? AdaptrexProperties.SENCHATOUCH_FOLDER : AdaptrexProperties.EXT_FOLDER);
this.theme = properties.get(
this.touch ? AdaptrexProperties.SENCHATOUCH_THEME : AdaptrexProperties.EXT_THEME);
this.request.setAttribute(ConfigComponent.ATTRIBUTE, this);
};
public String getJavaScript() {
this.isWritten = true;
/*
* If we don't have any namespace, alert and drop out
*/
if (this.namespace == null) {
return StringUtilities.getErrorJavaScript(
"Adaptrex Configuration Error: Missing ExtJS or Sencha Touch Namespace", "",
"This can be configured in in the adaptrex config component parameter 'ns'"
);
}
/*
* Ext Version must be specified
*/
if (this.sdkVersion == null) {
return StringUtilities.getErrorJavaScript(
"Adaptrex Configuration Error: Missing ExtJS or Sencha Touch Version", "",
"This can be configured in adaptrex.properties: " + AdaptrexProperties.EXT_FOLDER,
" or " + AdaptrexProperties.SENCHATOUCH_FOLDER
);
}
String contextPath = this.contextPath.equals("/") ? "" : this.contextPath;
String sdkType = this.touch ? "sencha-touch" : "ext";
String localSdkFolder = contextPath + "/adaptrex/" + (this.touch ? "sencha-touch" : "extjs");
String sdkDevFile = this.touch ? "debug" : "dev";
/*
* If the URI ends with an index.* file, strip the /index.* to get the path to the folder
* If the URI doesn't end in a slash, add the trailing slash to set the root for the js folders
* If our page is @ the context root, serve app.js from the "index" folder
*/
String appPath = this.request.getRequestURI();
if (appPath.contains("index.")) appPath = appPath.split("index\\.")[0];
if (!appPath.endsWith("/")) appPath += "/";
if (appPath.equals(request.getContextPath() + "/")) appPath += "index/";
String d = debug ? "-debug" : "";
String extFilePath = this.env.equals("production")
? appPath + "build/" + (this.touch ? "app-sencha-touch" + d +".js" : "app-extjs" + d + ".js")
: contextPath + "/" + weblibPath + "/" + this.sdkVersion + "/" + sdkType + "-" + sdkDevFile + ".js";
String themePath = "";
String cssFolder = localSdkFolder + "/resources/css/";
if (this.touch) {
themePath = cssFolder + "sencha-touch.css";
} else {
themePath = cssFolder + "ext-all.css";
if (this.theme != null) {
if (this.theme.equals("gray")) {
themePath = cssFolder + "ext-all-gray.css";
} else if (this.theme.equals("access")) {
themePath = cssFolder + "ext-all-access.css";
} else if (this.theme.equals("neptune")) {
themePath = cssFolder + "ext-neptune.css";
} else if (this.theme.startsWith("/")) {
themePath = this.contextPath + this.theme;
} else {
themePath = cssFolder + this.theme + ".css";
}
}
}
/*
* Write our ext/adaptrex script tags
*/
StringBuilder output = new StringBuilder("\n");
output.append("\n");
output.append("\n");
if (this.theme != null && this.theme.contains("neptune")) {
output.append("\n");
}
if (!this.env.equals("production"))
output.append("\n");
output.append("\n");
return output.toString();
}
public boolean isWritten() {
return this.isWritten;
}
public String getNamespace() {
return this.namespace;
}
public ConfigComponent applyWeblibPath(String weblibPath) {
if (weblibPath != null) this.weblibPath = weblibPath;
return this;
}
/*
* Environment (dev, production, debug)
*/
public ConfigComponent applyEnv(String env) {
if (env != null) this.env = env;
return this;
}
public String getEnv() {
return this.env;
}
/*
* Ext Config
*/
public ConfigComponent applyExtVersion(String extVersion) {
if (extVersion != null) this.sdkVersion = extVersion;
return this;
}
public ConfigComponent applyExtTheme(String extTheme) {
if (extTheme != null) this.theme = extTheme;
return this;
}
public ConfigComponent applySenchaTouchVersion(String senchaTouchVersion) {
if (senchaTouchVersion != null) this.sdkVersion = senchaTouchVersion;
return this;
}
public ConfigComponent applySenchaTouchTheme(String senchaTouchTheme) {
if (senchaTouchTheme != null) this.theme = senchaTouchTheme;
return this;
}
public boolean isTouch() {
return this.touch;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy