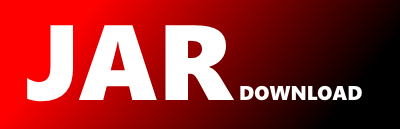
com.adaptrex.core.view.ModelComponent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adaptrex-core Show documentation
Show all versions of adaptrex-core Show documentation
The Core Adaptrex Framework
The newest version!
/*
* Copyright 2012 Adaptrex, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.adaptrex.core.view;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.apache.log4j.Logger;
import com.adaptrex.core.Adaptrex;
import com.adaptrex.core.AdaptrexProperties;
import com.adaptrex.core.ext.Association;
import com.adaptrex.core.ext.ExtConfig;
import com.adaptrex.core.ext.ModelDefinition;
import com.adaptrex.core.ext.ModelInstance;
import com.adaptrex.core.ext.RestProxy;
import com.adaptrex.core.persistence.api.AdaptrexPersistenceManager;
import com.adaptrex.core.utilities.StringUtilities;
public class ModelComponent {
private static Logger log = Logger.getLogger(ModelComponent.class);
private HttpServletRequest request;
private ExtConfig extConfig;
private Object entityId;
private String entityKey;
private Object entityValue;
private RestProxy restProxy;
private ConfigComponent configComponent;
private String namespace = null;
public ModelComponent(HttpServletRequest request, ConfigComponent configComponent, ExtConfig extConfig, String namespace) {
this.request = request;
this.configComponent = configComponent;
this.extConfig = extConfig;
this.namespace = namespace;
}
public ModelComponent(HttpServletRequest request, String entityClassName, String factoryName, String namespace) {
this.request = request;
this.namespace = namespace;
/*
* Get or create our config component
*/
this.configComponent = (ConfigComponent) request.getAttribute(ConfigComponent.ATTRIBUTE);
if (this.configComponent == null) {
configComponent = new ConfigComponent(request, namespace, false);
this.configComponent = (ConfigComponent) request.getAttribute(ConfigComponent.ATTRIBUTE);
}
this.extConfig = new ExtConfig(request.getServletContext(), entityClassName, factoryName);
};
public String getJavaScript() throws Exception {
ExtConfig config = this.extConfig;
AdaptrexPersistenceManager apm = config.getORMPersistenceManager();
if (config.getEntityClass() == null) {
String str = "Adaptrex Model Error: Persistent Class Not Found (" + this.extConfig.getClassName() + ")";
log.warn(str);
return StringUtilities.getErrorJavaScript(str);
}
if (this.namespace == null) {
this.namespace = configComponent.getNamespace();
}
String modelName = config.getModelName();
List modelDefinitionStrings = new ArrayList();
String fullModelName = namespace + ".model." + modelName;
boolean isTouch = this.configComponent.isTouch();
/*
* Get the model definition
*/
log.debug("Creating model definition for " + fullModelName);
ModelDefinition modelDefinition = new ModelDefinition(this.extConfig);
/*
* Add the rest API to the model
*/
modelDefinition.setProxy(this.restProxy);
modelDefinitionStrings.add("Ext.define('" + fullModelName + "', " +
(isTouch ? "{extend:'Ext.data.Model', config:" : "") +
StringUtilities.json(modelDefinition, this.request.getServletContext()) + (isTouch ? "}" : "") + ");\n");
/*
* Get the model's association definitions
*/
getAllAssociations(modelDefinition);
for (Association association : associations) {
ModelDefinition assocModelDef = association.getModelDefinition();
if (assocModelDef != null) {
/*
* If we have a rest proxy for the parent model, add one for associated models
*/
if (this.restProxy != null) {
String restPath = getRestPath(association.getEntityClassName().toLowerCase());
if (!restPath.equals("false")) {
RestProxy associatedProxy = new RestProxy(restPath, extConfig, this.configComponent.isTouch());
assocModelDef.setProxy(associatedProxy);
}
}
modelDefinitionStrings.add("Ext.define('" + assocModelDef.getModelName() + "', " +
(isTouch ? "{extend:'Ext.data.Model', config:" : "") +
StringUtilities.json(assocModelDef, this.request.getServletContext()) + (isTouch ? "}" : "") + ");\n");
}
}
/*
* If we have an entity ID or a key/value configuration on our component,
* we also return an online instance
* TODO: We should add the ability to load this via rest to enable associations
*/
String modelInstanceString = "";
if (this.entityId != null || entityKey != null) {
ModelInstance modelInstance = this.entityId != null
? apm.getModelInstance(this.extConfig, this.entityId)
: apm.getModelInstance(this.extConfig, this.entityKey, this.entityValue);
// ModelInstance modelInstance = this.entityId != null
// ? new ModelInstance(extConfig, this.entityId)
// : new ModelInstance(extConfig, this.entityKey, this.entityValue);
modelInstanceString = "Ext.ns('" + namespace + ".instance');" + "\n" +
namespace + ".instance." + this.extConfig.getModelName() + "=Ext.create('" +
fullModelName + "', " +
StringUtilities.json(modelInstance.getData(), this.request.getServletContext()) + ");\n";
}
StringBuffer javascript = new StringBuffer();
if (!configComponent.isWritten()) {
javascript.append(configComponent.getJavaScript());
}
javascript.append("\n");
return javascript.toString();
}
RestProxy getRestProxy() {
return this.restProxy;
}
private List associations = new ArrayList();
private void getAllAssociations(ModelDefinition baseModelDefinition) {
for (Association assoc : baseModelDefinition.getAssociations()) {
associations.add(assoc);
if (assoc.getModelDefinition() != null) {
getAllAssociations(assoc.getModelDefinition());
}
}
}
public ModelComponent applyModelName(String modelName) {
if (modelName != null) {
this.extConfig.setModelName(modelName);
}
return this;
}
public ModelComponent applyRest(Object restParam) {
String restPath = getRestPath(restParam);
if (!restPath.equals("false")) {
this.restProxy = new RestProxy(restPath, extConfig, this.configComponent.isTouch());
}
return this;
}
private String getRestPath(Object restParam) {
Adaptrex adaptrex = Adaptrex.get(request.getServletContext());
String restRoot = "/" + adaptrex.getProperties().get(AdaptrexProperties.REST_PATH, "rest") + "/";
String rest = null;
if (restParam instanceof Boolean) {
rest = String.valueOf(restParam);
} else {
rest = (String) restParam;
}
if (rest == null || rest.equals("false")) return "false";
String restPath = null;
if (rest.equals("true")) {
restPath = this.extConfig.getEntityClass().getSimpleName().toLowerCase();
if (this.extConfig.getFactoryName() != null) {
restPath = this.extConfig.getFactoryName() + "/" + restPath;
}
return this.request.getContextPath() + restRoot + restPath;
} else if (rest.startsWith("/")) {
return rest;
} else {
return this.request.getContextPath() + restRoot + rest;
}
}
public ModelComponent applyInclude(String includes) {
if (includes != null) this.extConfig.setIncludes(includes);
return this;
}
public ModelComponent applyExclude(String excludes) {
if (excludes != null) this.extConfig.setExcludes(excludes);
return this;
}
public ModelComponent applyAssociations(String associations) {
if (associations != null) this.extConfig.setAssociations(associations);
return this;
}
public ModelComponent applyEntityId(Object entityId) {
if (entityId != null) this.entityId = entityId;
return this;
}
public ModelComponent applyEntityKey(String entityKey) {
if (entityKey != null) this.entityKey = entityKey;
return this;
}
public ModelComponent applyEntityValue(String entityValue) {
if (entityValue != null) this.entityValue = entityValue;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy