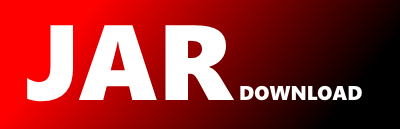
com.addc.commons.queue.BoundedNotifyingQueueTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-queues Show documentation
Show all versions of addc-queues Show documentation
The addc-queues library supplies support for internal persistent queues using an optional DERBY database for storage.
The newest version!
package com.addc.commons.queue;
import java.util.Timer;
import java.util.TimerTask;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The BoundedNotifyingQueueTimer starts a task that will empty a
* {@link BoundedNotifyingQueue} at given time intervals
*
*/
public class BoundedNotifyingQueueTimer {
private static final Logger LOGGER= LoggerFactory.getLogger(BoundedNotifyingQueueTimer.class);
private final BoundedNotifyingQueue> notifyingQueue;
private final long sendTimerPeriod;
private BNQTimerTask timerTask;
private Timer timer;
private TimerState state;
/**
* Constructor
*
* @param notifyingQueue
* The event queue to trigger
* @param delay
* The delay between ticks
*/
public BoundedNotifyingQueueTimer(BoundedNotifyingQueue> notifyingQueue, long delay) {
this.timer= new Timer(true);
this.notifyingQueue= notifyingQueue;
this.timerTask= new BNQTimerTask(notifyingQueue);
this.sendTimerPeriod= delay;
this.state= TimerState.STOPPED;
LOGGER.info("Created Bounded Notifying Queue timer");
}
/**
* Starts the event queue timer by scheduling a timer task based on the
* delay specified at creation time.
*/
public void start() {
if (state == TimerState.STOPPED) {
scheduleTimerTask();
state= TimerState.RUNNING;
LOGGER.info("Bounded Notifying Queue timer is started");
}
}
/**
* Stop the timer
*/
public void shutdown() {
if (state != TimerState.STOPPED) {
timer.cancel();
state= TimerState.STOPPED;
LOGGER.info("Bounded Notifying Queue timer is shut down");
}
}
/**
* Pause the timer
*/
public void pause() {
if (state == TimerState.RUNNING) {
state= TimerState.PAUSED;
timerTask.cancel();
LOGGER.debug("Bounded Notifying Queue timer is paused");
}
}
/**
* Resume the timer
*/
public void resume() {
if (state == TimerState.PAUSED) {
timer= new Timer(true);
scheduleTimerTask();
state= TimerState.RUNNING;
LOGGER.debug("Bounded Notifying Queue timer is resumed");
}
}
/**
* Get the state of the timer
*
* @return the state of the timer
*/
public TimerState getState() {
return state;
}
private void scheduleTimerTask() {
timerTask= new BNQTimerTask(notifyingQueue);
timer.schedule(timerTask, sendTimerPeriod, sendTimerPeriod);
}
/**
* A timer task which triggers the {@link BoundedNotifyingQueue} to empty
* and send its contents to the listeners
*/
private static class BNQTimerTask extends TimerTask {
private final BoundedNotifyingQueue> eventQueue;
private static final Logger LOG= LoggerFactory.getLogger(BNQTimerTask.class);
/**
* Constructor
*
* @param notifyingQueue
* the event queue should be triggered
*/
public BNQTimerTask(BoundedNotifyingQueue> eventQueue) {
this.eventQueue= eventQueue;
}
@Override
public void run() {
LOG.debug("Timer is triggering empty on Bounded Notifying Queue");
eventQueue.empty();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy