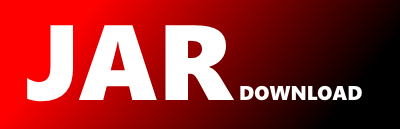
com.addc.commons.slp.SLPRegistrarThread Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.addc.commons.Constants;
import com.addc.commons.alerts.Alert;
import com.addc.commons.alerts.AlertType;
import com.addc.commons.alerts.Level;
import com.addc.commons.alerts.Notifier;
import com.addc.commons.i18n.I18nText;
import com.addc.commons.i18n.I18nTextFactory;
import com.addc.commons.i18n.Translator;
import com.addc.commons.shutdown.Stoppable;
/**
* The SLPRegistrarThread supplies a thread that renews the registration with the SLP Daemon every time it expires until the thread
* terminates when it deregisters the service.
*/
public class SLPRegistrarThread extends Thread implements Stoppable {
private static final Logger LOGGER= LoggerFactory.getLogger(SLPRegistrarThread.class);
private static final String SLP_REG_FAIL= I18nText.markKey("Failed to register service {0}");
private static final String SLP_DEREG_FAIL= I18nText.markKey("Failed to deregister service {0}");
private static final String AGENT_KEY= "AgentName";
private final ServiceURL serviceURL;
private final ServiceLocationManager slpManager;
private final Translator translator;
private final List attributes= new ArrayList<>();
private Advertiser slpAdvertiser;
private Notifier notifier;
private boolean shutdown;
/**
* Create a new SLPRegistrarThread
*
* @param manager
* The {@link ServiceLocationManager} to use
* @param url
* The url to register
* @param serviceName
* The name of the service to register
* @param notifier
* The notifier to use (may be null)
*
* @throws SLPRegistrarException
* if the URL is invalid
*/
@SuppressWarnings("PMD.AvoidThrowingNullPointerException")
public SLPRegistrarThread(ServiceLocationManager manager, ServiceURL url, String serviceName, Notifier notifier)
throws SLPRegistrarException {
super("SLPRegistrar-" + serviceName);
if (manager == null) {
throw new NullPointerException("The ServiceLocationManager cannot be null");
}
if (url == null) {
throw new NullPointerException("The ServiceURL cannot be null");
}
if (serviceName == null) {
throw new NullPointerException("The Service Name cannot be null");
}
setPriority(3);
this.notifier= notifier;
this.serviceURL= url;
this.slpManager= manager;
LOGGER.info("Registering URL for {}:{}", serviceName, url);
// Prepare Lookup Attributes
// Add the scope
List> attrValues= new ArrayList<>();
attrValues.add(new StringAttributeValue(serviceName));
ServiceLocationAttribute attr2;
try {
attr2= new ServiceLocationAttribute(AGENT_KEY, attrValues);
} catch (ServiceLocationException e) {
LOGGER.error("Failed to create attribute", e);
throw new SLPRegistrarException("Failed to create attribute", e);
}
attributes.add(attr2);
this.translator= I18nTextFactory.getTranslator(Constants.BASENAME, Locale.getDefault());
}
@Override
public void run() {
LOGGER.info("starts");
boolean registered= false;
int errorCount= 0;
while (!shutdown) {
if (slpAdvertiser == null) {
slpAdvertiser= slpManager.getAdvertiser();
}
try {
slpAdvertiser.register(serviceURL, attributes);
errorCount= 0;
if (!registered) {
LOGGER.info("Registered URL: {}", serviceURL);
registered= true;
}
} catch (ServiceLocationException e) {
LOGGER.warn("Failed to register with SLP", e);
errorCount++;
if (errorCount >= 10) {
slpAdvertiser= null;
registered= false;
alert(Level.WARN, SLP_REG_FAIL, serviceURL);
errorCount= 0;
}
}
if (!shutdown) {
try {
sleep(serviceURL.getLifetime() * 1000L);
} catch (InterruptedException e) {
LOGGER.debug("Interrupted", e);
}
}
}
if (slpAdvertiser != null) {
try {
slpAdvertiser.deregister(serviceURL);
LOGGER.info("Deregistered URL: {}", serviceURL);
} catch (ServiceLocationException e) {
LOGGER.warn("Failed to deregister with SLP", e);
alert(Level.WARN, SLP_DEREG_FAIL, serviceURL);
}
}
LOGGER.info("ends");
}
@Override
public void shutdown() {
setPriority(9);
LOGGER.info("Received shutdown request.");
shutdown= true;
interrupt();
try {
join();
} catch (InterruptedException e) {
LOGGER.debug("", e);
}
}
/**
* Get the notifier
*
* @return the notifier
*/
public Notifier getNotifier() {
return notifier;
}
/**
* Set the notifier
*
* @param notifier
* the notifier to set
*/
public void setNotifier(Notifier notifier) {
this.notifier= notifier;
}
private void alert(Level level, String message, Object... args) {
if (notifier != null) {
AlertType alertType= new AlertType(message, level);
Set sources= new HashSet<>();
sources.add("SLP");
notifier.notifyAlert(new Alert(alertType, sources, args), translator);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy