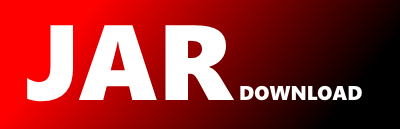
com.addc.commons.slp.ServiceLocationAttribute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* Implementation of the SLP ServiceLocationAttribute class defined in RFC 2614.
*/
public class ServiceLocationAttribute {
private final SLPAttributeHelper helper= SLPAttributeHelper.getInstance();
private final List> values= new ArrayList<>();
private String id;
/**
* Create a new ServiceLocationAttribute
*
* @param id
* The attribute id.
* @param values
* The attribute values.
* @throws ServiceLocationException
* if the id is null or contains a bad-tag
*
*/
public ServiceLocationAttribute(String id, List> values) throws ServiceLocationException {
if (id == null) {
throw new ServiceLocationException("The attribute id cannot be null", SLPConstants.TYPE_ERROR);
}
if (helper.containsBadTag(id)) {
throw new ServiceLocationException("The attribute id contains bad tags ('\\n', '\\r', '\\t', '_' or '*')", SLPConstants.TYPE_ERROR);
}
this.id= id;
if (values != null) {
this.values.addAll(values);
}
}
/**
* Create a new ServiceLocationAttribute
*
* @param attr
* The attribute String.
*/
@SuppressWarnings("PMD.AssignmentInOperand")
public ServiceLocationAttribute(String attr) {
if (attr.charAt(0) == '(') {
int pos= attr.indexOf('=');
int endPos= 0;
id= helper.decode(attr.substring(1, pos));
pos++;
while ((endPos= attr.indexOf(',', pos)) != -1) {
values.add(helper.unEscape(attr.substring(pos, endPos)));
pos= endPos + 1;
}
values.add(helper.unEscape(attr.substring(pos, attr.length() - 1)));
} else {
id= helper.decode(attr);
}
}
/**
* Get a copy of the values.
*
* @return a copy of the values.
*/
public List> getValues() {
return new ArrayList<>(values);
}
/**
* Get the id.
*
* @return the id.
*/
public String getId() {
return id;
}
/**
* Get the escaped string representation of this attribute
*
* @return the escaped string representation of this attribute
*/
public String getEscapedString() {
StringBuilder sb= new StringBuilder();
if (values.isEmpty()) {
sb.append(helper.escapeString(id));
} else {
sb.append('(').append(helper.escapeString(id)).append('=');
for (Iterator> iterator= values.iterator(); iterator.hasNext();) {
sb.append(iterator.next().getEscapedString());
if (iterator.hasNext()) {
sb.append(',');
}
}
sb.append(')');
}
return sb.toString();
}
@Override
public int hashCode() {
final int prime= 31;
int result= 1;
result= prime * result + id.hashCode();
for(AttributeValue> attr : values) {
result= prime * result + attr.hashCode();
}
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceLocationAttribute)) {
return false;
}
ServiceLocationAttribute other= (ServiceLocationAttribute) obj;
if (!id.equals(other.id)) {
return false;
}
if (values.size() != other.values.size()) {
return false;
}
Iterator> e1 = values.iterator();
Iterator> e2 = other.values.iterator();
while (e1.hasNext() && e2.hasNext()) {
AttributeValue> o1 = e1.next();
AttributeValue> o2 = e2.next();
if (!o1.equals(o2)) {
return false;
}
}
return true;
}
@Override
public String toString() {
StringBuffer buf= new StringBuffer();
if (values.isEmpty()) {
buf.append(id);
} else {
buf.append('(').append(id).append('=');
for (Iterator> iter= values.iterator();iter.hasNext();) {
buf.append(iter.next());
if (iter.hasNext()) {
buf.append(',');
}
}
buf.append(')');
}
return buf.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy