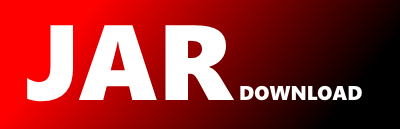
com.addc.commons.slp.ServiceLocationManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import com.addc.commons.Mutex;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* Implementation of the SLP ServiceLocationManager class defined in RFC 2614.
*/
public class ServiceLocationManager {
private final SLPConfig config;
private final NetworkManager netManager;
private final Mutex mutex= new Mutex();
private final List scopes= new ArrayList<>();
private Locator locator;
private Advertiser advertiser;
/**
* Returns the number of seconds since Jan 1, 1970
*
* @return the number of seconds since Jan 1, 1970
*/
public static int getTimestamp() {
long systemTime= System.currentTimeMillis();
return (int) (systemTime / 1000);
}
/**
* Create a new ServiceLocationManager
*
* @param config
* The configuration to use
* @param netManager
* The network manager to use
*/
public ServiceLocationManager(SLPConfig config, NetworkManager netManager) {
this.config= config;
this.netManager= netManager;
scopes.addAll(Arrays.asList(StringUtils.split(config.getScopes(), ',')));
}
/**
* Get a locator.
*
* @return a locator.
*/
public Locator getLocator() {
synchronized (mutex) {
if (locator == null) {
locator= new LocatorImpl(config, netManager);
}
}
return locator;
}
/**
* Get an Advertizer.
*
* @return an Advertizer.
*/
public Advertiser getAdvertiser() {
synchronized (mutex) {
if (advertiser == null) {
advertiser= new AdvertiserImpl(config, netManager);
}
}
return advertiser;
}
/**
* Get the config
*
* @return the config
*/
public SLPConfig getConfig() {
return config;
}
/**
* Get the netManager
*
* @return the netManager
*/
public NetworkManager getNetManager() {
return netManager;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy