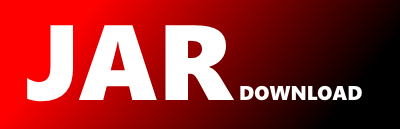
com.addc.commons.slp.SlpAttributeEnumeration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp;
import java.io.DataInputStream;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.addc.commons.Constants;
import com.addc.commons.slp.configuration.SLPConfig;
import com.addc.commons.slp.messages.SLPMessageHeader;
import com.addc.commons.slp.messages.UAMessage;
/**
* The SlpAttributeEnumeration supplies an enumerator for
* {@link ServiceLocationAttribute}s
*/
public class SlpAttributeEnumeration extends AbstractSlpEnumeration {
private static final Logger LOGGER= LoggerFactory.getLogger(SlpAttributeEnumeration.class);
/**
* Create a new SlpAttributeEnumeration
*
* @param config
* The configuration to use
* @param netManager
* The NetworkManagerImpl
* @param message
* The request message
* @throws ServiceLocationException
* If there is an error sending or receiving data
*/
public SlpAttributeEnumeration(SLPConfig config, NetworkManager netManager, UAMessage message)
throws ServiceLocationException {
super(config, netManager, message);
}
@Override
protected void parseResponse(DataInputStream dis, SLPMessageHeader header) throws IOException, ServiceLocationException {
if (header.getFunctionId() != SLPConstants.ATTRRPLY) {
String reason= MessageFormat.format("Expected function Id {0} received {1}", SLPConstants.ATTRRPLY, header.getFunctionId());
LOGGER.error(reason);
throw new ServiceLocationException(reason, SLPConstants.INTERNAL_SYSTEM_ERROR);
}
int size= dis.readShort();
LOGGER.debug("Received ATTRRPLY {} size {}", header.getFunctionId(), size);
byte[] attrs= new byte[size];
int x= dis.read(attrs);
if (x != size) {
String msg= MessageFormat.format("Only read {0} bytes of {1}", x, size);
LOGGER.warn(msg);
throw new IOException(msg);
}
String list= new String(attrs, Constants.UTF8);
AuthenticationBlock[] authBlocks= new AuthenticationBlock[dis.readByte()];
for (int j= 0; j < authBlocks.length; j++) {
authBlocks[j]= AuthenticationBlock.readBlock(config, dis);
}
if (SLPAttributeHelper.getInstance().verifyList(config.isSecurityEnabled(), list, authBlocks)) {
List slaList= SLPAttributeHelper.getInstance().readFromList(list);
for (ServiceLocationAttribute sla : slaList) {
received.add(sla);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy