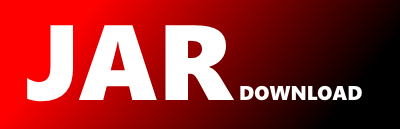
com.addc.commons.slp.configuration.SLPConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.configuration;
import java.io.IOException;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.cert.X509Certificate;
import java.util.Arrays;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import org.apache.commons.lang.StringUtils;
import com.addc.commons.passwd.Password;
import com.addc.commons.passwd.PasswordChecker;
import com.addc.commons.properties.BoundsFactory;
import com.addc.commons.properties.PropertiesParser;
import com.addc.commons.security.keys.PemKeyCertHelper;
import com.addc.commons.slp.SLPConstants;
/**
* An SLPConfiguration object holds all configurable properties.
*/
public class SLPConfig implements SLPConfigurationMBean {
private String scopes;
private int mcastMaxWait;
private int[] mcastTimeouts;
private int[] dgramTimeouts;
private int mtu;
private Locale locale;
private boolean securityEnabled;
private String spi;
private List daAddresses;
private boolean enabled;
private int port;
private PublicKey publicKey;
private PrivateKey privateKey;
private String privateKeyFile;
private String publicKeyFile;
private Password privateKeyPass;
/**
*
* Create a new SLPConfig
*
* @param parser
* The parser to use to get the properties
*/
public SLPConfig(PropertiesParser parser) {
this(parser, "");
}
/**
* Create a new SLPConfig
*
* @param parser
* The parser to use to get the properties
* @param prefix
* The prefix for the property keys
*/
public SLPConfig(PropertiesParser parser, String prefix) {
PasswordChecker checker= new PasswordChecker(parser.getProperties(), parser.getParserErrors());
this.enabled= parser.parseBoolean(prefix + SLPConfigConstants.SLP_ENABLED);
this.scopes= parser.parseString(prefix + SLPConfigConstants.USE_SCOPES, SLPConfigConstants.USE_SCOPES_DEFAULT);
this.port= parser.parseInteger(prefix + SLPConfigConstants.SLP_PORT, BoundsFactory.getIntBoundsGt(0),
SLPConstants.SLP_RESERVED_PORT);
String value= parser.parseString(prefix + SLPConfigConstants.DA_ADDRESSES,
SLPConfigConstants.DA_ADDRESSES_DEFAULT);
String[] parts= StringUtils.split(value, ',');
daAddresses= Arrays.asList(parts);
this.mcastMaxWait= parser.parseInteger(prefix + SLPConfigConstants.MCAST_MAX_WAIT,
SLPConfigConstants.MCAST_MAX_WAIT_DEFAULT);
value= parser.parseString(prefix + SLPConfigConstants.MCAST_TIMEOUTS,
SLPConfigConstants.MCAST_TIMEOUTS_DEFAULT);
this.mcastTimeouts= parseTimeouts(value, parser.getParserErrors());
value= parser.parseString(prefix + SLPConfigConstants.DATAGRAM_TIMEOUTS,
SLPConfigConstants.DATAGRAM_TIMEOUTS_DEFAULT);
this.dgramTimeouts= parseTimeouts(value, parser.getParserErrors());
this.mtu= parser.parseInteger(prefix + SLPConfigConstants.MTU, BoundsFactory.getIntBoundsGte(68),
SLPConfigConstants.MTU_DEFAULT);
value= parser.parseString(prefix + SLPConfigConstants.LOCALE, SLPConfigConstants.LOCALE_DEFAULT);
locale= new Locale(value);
this.securityEnabled= parser.parseBoolean(prefix + SLPConfigConstants.SECURITY_ENABLED, false);
if (securityEnabled) {
this.spi= parser.parseString(prefix + SLPConfigConstants.SPI, SLPConfigConstants.SPI_DEFAULT);
this.privateKeyFile= parser.parseString(prefix + SLPConfigConstants.PRIVATE_KEY);
this.privateKeyPass= checker.getClearPassword(prefix + SLPConfigConstants.PRIVATE_KEY_PASS);
this.publicKeyFile= parser.parseString(prefix + SLPConfigConstants.PUBLIC_KEY);
} else {
this.privateKey= null;
this.publicKey= null;
this.spi= null;
}
}
@Override
public String getScopes() {
return scopes;
}
@Override
public int getMcastMaxWait() {
return mcastMaxWait;
}
@Override
public int[] getMcastTimeouts() {
return Arrays.copyOf(mcastTimeouts, mcastTimeouts.length);
}
@Override
public int[] getDatagramTimeouts() {
return Arrays.copyOf(dgramTimeouts, dgramTimeouts.length);
}
@Override
public int getMTU() {
return mtu;
}
@Override
public Locale getLocale() {
return locale;
}
@Override
public boolean isSecurityEnabled() {
return securityEnabled;
}
@Override
public String getSPI() {
return spi;
}
public List getDaAddresses() {
return daAddresses;
}
/**
* Get the Public Key.
*
* @param spIndex
* The Security Parameter Index to use.
* @return the Public Key.
* @throws IOException
* If the file cannot be read
*/
public PublicKey getPublicKey(String spIndex) throws IOException {
if ((spi == null) || !spi.equals(spIndex)) {
return null;
}
if (publicKey == null) {
X509Certificate[] chain= PemKeyCertHelper.getInstance().readX509CertChainFromPEM(publicKeyFile);
if (chain.length > 0) {
publicKey= chain[0].getPublicKey();
}
}
return publicKey;
}
/**
* Get the Private Key.
*
* @param spIndex
* The Security Parameter Index to use.
* @return the Private Key.
* @throws IOException
* If the file cannot be read
*/
public PrivateKey getPrivateKey(String spIndex) throws IOException {
if ((spi == null) || !spi.equals(spIndex)) {
return null;
}
if (privateKey == null) {
privateKey= PemKeyCertHelper.getInstance().readPrivateKeyFromPEM(privateKeyFile,
privateKeyPass.getPasswd());
}
return privateKey;
}
/**
* Parse timeouts.
*
* @param s
* The timeouts string.
* @return an array of timeouts
*/
private int[] parseTimeouts(String s, Set errors) {
String[] parts= StringUtils.split(s, ',');
int[] timeouts= new int[parts.length];
int i= 0;
for (String str : parts) {
int tout= 0;
try {
tout= Integer.parseInt(str.trim());
if (tout < 500) {
errors.add("Timeouts must be >= 500 (" + tout + ")");
}
} catch (@SuppressWarnings("unused") NumberFormatException e) {
errors.add("Invalid timeout (" + str + ")");
}
timeouts[i++]= tout;
}
return timeouts;
}
@Override
public boolean isEnabled() {
return enabled;
}
@Override
public int getPort() {
return port;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy