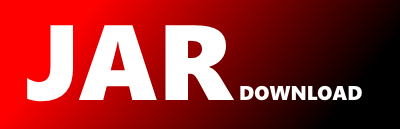
com.addc.commons.slp.messages.AttributeRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.messages;
import java.io.DataOutputStream;
import java.io.IOException;
import com.addc.commons.slp.SLPConstants;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* The AttributeRequest SLP Message.
*/
public class AttributeRequest extends UAMessage {
private final String scopes;
private final String attributeIds;
private final String url;
private String spi;
/**
* Create a new AttributeRequest
*
* @param config
* The SLP configuration to use
* @param url
* The URL to query
* @param attributeIds
* A comma delimited list of attribute ids
*/
public AttributeRequest(SLPConfig config, String url, String attributeIds) {
super(config, SLPConstants.ATTRRQST);
this.url= url;
this.scopes= config.getScopes();
this.attributeIds= attributeIds== null ? "" : attributeIds;
if (config.isSecurityEnabled()) {
spi= config.getSPI();
} else {
spi= "";
}
}
@Override
public int calcSize() {
return 10 + getResponders().length() + url.length() + scopes.length() + attributeIds.length() + spi.length();
}
@Override
protected void writeBody(DataOutputStream out) throws IOException {
out.writeUTF(getResponders());
out.writeUTF(url);
out.writeUTF(scopes);
out.writeUTF(attributeIds);
out.writeUTF(spi);
}
@Override
public int hashCode() {
final int prime= 31;
int result= super.hashCode();
result= prime * result + attributeIds.hashCode();
result= prime * result + scopes.hashCode();
result= prime * result + spi.hashCode();
result= prime * result + url.hashCode();
return result;
}
@Override
@SuppressWarnings({ "PMD.CyclomaticComplexity", "PMD.NPathComplexity" })
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AttributeRequest)) {
return false;
}
AttributeRequest other= (AttributeRequest) obj;
return (super.equals(obj) && attributeIds.equals(other.attributeIds) && scopes.equals(other.scopes)
&& spi.equals(other.spi) && url.equals(other.url));
}
@Override
public String toString() {
StringBuilder builder= new StringBuilder();
builder.append("AttributeRequest [");
builder.append(super.toString());
builder.append(", scopes=");
builder.append(scopes);
builder.append(", attributeIds=");
builder.append(attributeIds);
builder.append(", url=");
builder.append(url);
builder.append(", spi=");
builder.append(spi);
builder.append(']');
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy