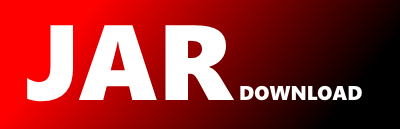
com.addc.commons.slp.messages.SLPMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.messages;
import java.io.DataOutputStream;
import java.io.IOException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* SLPMessage is the base for all messages sent from the SLP client.
*/
public abstract class SLPMessage {
private static final Logger LOGGER= LoggerFactory.getLogger(SLPMessage.class);
private final SLPConfig config;
private final int msgType;
private int xid;
private boolean multicast;
private boolean fresh;
/**
* Create a new SLPMessage
*
* @param config
* The configuration to use
* @param msgType
* The message type
*/
protected SLPMessage(SLPConfig config, int msgType) {
this.config= config;
this.msgType= msgType;
}
/**
* Sets the XID that this message was sent with.
*
* @param xid
* the XID
*/
public void setXid(int xid) {
this.xid= xid;
}
/**
* Query whether the address is multicast
*
* @return whether the address is multicast
*/
public boolean isMulticast() {
return multicast;
}
/**
* Get the fresh
*
* @return the fresh
*/
public boolean isFresh() {
return fresh;
}
/**
* Set the fresh
*
* @param fresh
* the fresh to set
*/
public void setFresh(boolean fresh) {
this.fresh= fresh;
}
/**
* Set whether the address is multicast
*
* @param multicast
* true if the address is multicast
*/
public void setMulticast(boolean multicast) {
this.multicast= multicast;
}
/**
* Gets the XID this message was sent with.
*
* @return the XID
*/
public int getXid() {
return xid;
}
/**
* Write the message.
*
* @param out
* The DataOutStream to write to.
* @param tcp
* true
if using a TCP connection
* @return true
if successful.
* @throws IOException
* If there is an IO error.
*/
public boolean writeMessage(DataOutputStream out, boolean tcp) throws IOException {
boolean result= false;
if (writeHeader(out, calcSize(), tcp)) {
writeBody(out);
result= true;
}
return result;
}
/**
* Implemented by subclasses to return the size required by the body of the
* message.
*
* @return The size of the message.
*/
public abstract int calcSize();
/**
* Implemented by subclasses to write the message body to a DataOutput. The
* number of bytes written should be equal to calcSize()
*
* @param out
* The DataOutStream to write to
* @throws IOException
* If there is an IO error.
*/
protected abstract void writeBody(DataOutputStream out) throws IOException;
/**
* Writes the standard SLP header to a DataOutput.
*
* @param out
* The DataOutStream to write to.
* @param bodySize
* The size of the message body.
* @return true if successful.
* @throws IOException
* If there is an IO error.
*/
private boolean writeHeader(DataOutputStream out, int bodySize, boolean tcp) throws IOException {
int msgSize= 14 + config.getLocale().getLanguage().length() + bodySize;
if (msgSize > config.getMTU()) {
LOGGER.warn("Message size ({}) exceeds MTU size ({})", msgSize, config.getMTU());
return false;
}
SLPMessageHeader header= new SLPMessageHeader(2, msgType, xid, msgSize, fresh, multicast, config.getLocale(),
tcp, config.getMTU());
header.write(out);
return true;
}
@Override
public int hashCode() {
final int prime= 31;
int result= 1;
result= prime * result + msgType;
result= prime * result + xid;
return result;
}
@Override
public boolean equals(Object obj) {
SLPMessage other= (SLPMessage) obj;
return ((msgType == other.msgType) && (xid == other.xid));
}
@Override
public String toString() {
StringBuilder builder= new StringBuilder();
builder.append("msgType=");
builder.append(msgType);
builder.append(", xid=");
builder.append(xid);
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy