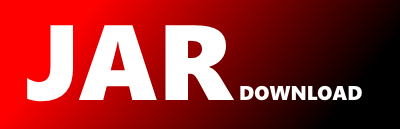
com.addc.commons.slp.messages.ServiceRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.messages;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import com.addc.commons.ObjectEquals;
import com.addc.commons.slp.AuthenticationBlock;
import com.addc.commons.slp.SLPAttributeHelper;
import com.addc.commons.slp.SLPConstants;
import com.addc.commons.slp.ServiceLocationAttribute;
import com.addc.commons.slp.ServiceLocationException;
import com.addc.commons.slp.ServiceLocationManager;
import com.addc.commons.slp.ServiceURL;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* The ServiceRegistration SLP Message.
*/
public class ServiceRegistration extends SLPMessage {
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder builder= new StringBuilder();
builder.append("ServiceRegistration [");
builder.append(super.toString());
builder.append(", serviceUrl=");
builder.append(serviceUrl);
builder.append(", attributes=");
builder.append(attributes);
builder.append(", scopes=");
builder.append(scopes);
builder.append(super.toString());
builder.append(']');
return builder.toString();
}
private final SLPAttributeHelper helper= SLPAttributeHelper.getInstance();
private final SLPConfig config;
private final ServiceURL serviceUrl;
private final List attributes;
private final List attrAuths;
private final String scopes;
/**
* Create a new ServiceRegistration message
*
* @param config
* The configuration to use
* @param serviceUrl
* The service URL.
* @param attributes
* The attributes to add
*/
public ServiceRegistration(SLPConfig config, ServiceURL serviceUrl, List attributes) {
super(config, SLPConstants.SRVREG);
super.setFresh(true);
this.serviceUrl= serviceUrl;
this.attributes= new ArrayList<>(attributes);
this.scopes= config.getScopes();
this.attrAuths= new ArrayList<>();
this.config= config;
}
/**
* Sign the message
*
* @throws ServiceLocationException
* If fails
*/
public void sign() throws ServiceLocationException {
if (config.isSecurityEnabled()) {
serviceUrl.sign();
if (!attributes.isEmpty()) {
try {
String[] spis= StringUtils.split(config.getSPI(), ',');
int timestamp= ServiceLocationManager.getTimestamp();
timestamp+= serviceUrl.getLifetime();
for (String spi : spis) {
byte[] data= getAuthData(spi, timestamp);
attrAuths.add(new AuthenticationBlock(config, 2, spi, timestamp, data));
}
} catch (IOException e) {
throw new ServiceLocationException("Could not sign URL", e, SLPConstants.AUTHENTICATION_FAILED);
}
}
}
}
@Override
protected void writeBody(DataOutputStream out) throws IOException {
serviceUrl.writeExternal(out); // URL entry
out.writeUTF(serviceUrl.getServiceType().toString());
out.writeUTF(scopes);
out.writeUTF(helper.getAttributesString(attributes));
// # of attr auths
out.writeByte(attrAuths.size());
for (AuthenticationBlock authBlock : attrAuths) {
authBlock.writeBlock(out);
}
}
@Override
public int calcSize() {
int size= 7 + serviceUrl.calcSize() + serviceUrl.getServiceType().size() + scopes.length()
+ helper.getAttributesString(attributes).length();
for (AuthenticationBlock authBlock : attrAuths) {
size+= authBlock.calcSize();
}
return size;
}
@Override
public int hashCode() {
final int prime= 31;
int result= super.hashCode();
result= prime * result + attrAuths.hashCode();
result= prime * result + attributes.hashCode();
result= prime * result + scopes.hashCode();
result= prime * result + serviceUrl.hashCode();
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceRegistration)) {
return false;
}
ServiceRegistration other= (ServiceRegistration) obj;
return (super.equals(obj) && ObjectEquals.areFieldsEqual(attrAuths, other.attrAuths)
&& ObjectEquals.areFieldsEqual(attributes, other.attributes)
&& ObjectEquals.areFieldsEqual(scopes, other.scopes)
&& ObjectEquals.areFieldsEqual(serviceUrl, other.serviceUrl));
}
private byte[] getAuthData(String spIndex, int timestamp) throws IOException {
ByteArrayOutputStream bos= new ByteArrayOutputStream();
DataOutputStream dos= new DataOutputStream(bos);
dos.writeUTF(spIndex);
dos.writeUTF(helper.getAttributesString(attributes));
dos.writeInt(timestamp);
return bos.toByteArray();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy