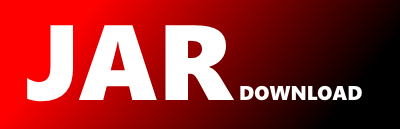
com.addc.commons.slp.messages.ServiceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.messages;
import java.io.DataOutputStream;
import java.io.IOException;
import com.addc.commons.slp.SLPConstants;
import com.addc.commons.slp.ServiceType;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* The ServiceRequest SLP Message.
*/
public class ServiceRequest extends UAMessage {
private ServiceType serviceType;
private String scopes;
private String searchFilter;
private String spi;
/**
* Create a new ServiceRequest with DEFAULT SPI
*
* @param config
* The configuration to use
* @param serviceType
* The service type
*/
public ServiceRequest(SLPConfig config, ServiceType serviceType) {
this(config, serviceType, "*");
}
/**
* Create a new ServiceRequest
*
* @param config
* The configuration to use
* @param serviceType
* The service type
* @param searchFilter
* The filter
*/
public ServiceRequest(SLPConfig config, ServiceType serviceType, String searchFilter) {
super(config, SLPConstants.SRVRQST);
this.serviceType= serviceType;
this.scopes= config.getScopes();
this.searchFilter= searchFilter == null ? "" : searchFilter;
if (config.isSecurityEnabled()) {
this.spi= config.getSPI();
} else {
spi= "";
}
}
@Override
public int calcSize() {
return 10 + getResponders().length() + serviceType.toString().length() + scopes.length() + searchFilter.length()
+ spi.length();
}
@Override
protected void writeBody(DataOutputStream out) throws IOException {
out.writeUTF(getResponders());
out.writeUTF(serviceType.toString());
out.writeUTF(scopes);
out.writeUTF(searchFilter);
out.writeUTF(spi);
}
@Override
public int hashCode() {
final int prime= 31;
int result= super.hashCode();
result= prime * result + scopes.hashCode();
result= prime * result + searchFilter.hashCode();
result= prime * result + serviceType.hashCode();
result= prime * result + spi.hashCode();
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceRequest)) {
return false;
}
ServiceRequest other= (ServiceRequest) obj;
return (super.equals(obj) && scopes.equals(other.scopes) && searchFilter.equals(other.searchFilter)
&& serviceType.equals(other.serviceType) && spi.equals(other.spi));
}
@Override
public String toString() {
StringBuilder builder= new StringBuilder();
builder.append("ServiceRequest [");
builder.append(super.toString());
builder.append(", serviceType=");
builder.append(serviceType);
builder.append(", scopes=");
builder.append(scopes);
builder.append(", searchFilter=");
builder.append(searchFilter);
builder.append(", spi=");
builder.append(spi);
builder.append(']');
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy