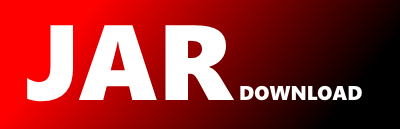
com.addc.commons.slp.messages.UAMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of addc-slp Show documentation
Show all versions of addc-slp Show documentation
The addc-slp library supplies client classes for registering objects with a Service Location Protocol Daemon and
for looking theses objects up later.
package com.addc.commons.slp.messages;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import com.addc.commons.ObjectEquals;
import com.addc.commons.slp.configuration.SLPConfig;
/**
* UAMessage is the base for User Agent messages
*/
public abstract class UAMessage extends SLPMessage {
private final List responders= new ArrayList<>();
private long sentAt= -1;
private int timesSent;
private int[] schedule;
/**
* Create a new UAMessage
*
* @param config
* The configuration to use
* @param msgType
* The message type
*/
protected UAMessage(SLPConfig config, int msgType) {
super(config, msgType);
}
/**
* Indicate a message was sent.
*/
public void sent() {
sentAt= System.currentTimeMillis();
timesSent++;
}
/**
* Set the transmission schedules,
*
* @param schedule
* the transmission schedules,
*/
public void setTransmitSchedule(int[] schedule) {
this.schedule= Arrays.copyOf(schedule, schedule.length);
}
/**
* Get the next timeout.
*
* @return the next timeout.
*/
public int getNextTimeout() {
int tout= 3000;
if (timesSent < schedule.length) {
int timeout= (int) (sentAt - System.currentTimeMillis() + schedule[timesSent - 1]);
if (timeout > 0) {
tout= timeout;
} else {
tout= 1;
}
}
return tout;
}
/**
* Add a responder.
*
* @param responder
* the responder to add.
*/
public void addResponder(String responder) {
responders.add(responder);
}
/**
* Get the responders.
*
* @return the responders.
*/
public String getResponders() {
StringBuilder resp= new StringBuilder();
if (responders != null) {
for (Iterator i= responders.iterator(); i.hasNext();) {
resp.append(i.next());
if (i.hasNext()) {
resp.append(',');
}
}
}
return resp.toString();
}
@Override
public int hashCode() {
final int prime= 31;
int result= super.hashCode();
result= prime * result + responders.hashCode();
result= prime * result + Arrays.hashCode(schedule);
result= prime * result + (int) (sentAt ^ (sentAt >>> 32));
result= prime * result + timesSent;
return result;
}
@Override
public boolean equals(Object obj) {
UAMessage other= (UAMessage) obj;
return (super.equals(obj) && ObjectEquals.areFieldsEqual(responders, other.responders)
&& Arrays.equals(schedule, other.schedule) && (sentAt == other.sentAt)
&& (timesSent == other.timesSent));
}
@Override
public String toString() {
StringBuilder builder= new StringBuilder();
builder.append(super.toString());
builder.append("sentAt=");
builder.append(sentAt);
builder.append(", timesSent=");
builder.append(timesSent);
builder.append(", schedule=");
builder.append(Arrays.toString(schedule));
builder.append(", responders=");
builder.append(responders);
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy