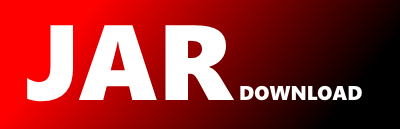
com.addc.server.ServerStopper Maven / Gradle / Ivy
package com.addc.server;
import java.io.IOException;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import javax.management.InstanceNotFoundException;
import javax.management.IntrospectionException;
import javax.management.MBeanException;
import javax.management.MBeanInfo;
import javax.management.MBeanOperationInfo;
import javax.management.MBeanServerConnection;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectInstance;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import javax.management.remote.JMXConnector;
import javax.management.remote.JMXConnectorFactory;
import javax.management.remote.JMXServiceURL;
import com.addc.commons.annotation.CoberturaIgnore;
import com.addc.commons.configuration.ConfigurationException;
import com.addc.commons.jmx.configuration.JMXConfig;
/**
* The ServerStopper supplies a command line program that will stop a server
* using the JMX interface
*/
@SuppressWarnings({ "PMD.DoNotCallSystemExit", "PMD.SystemPrintln" , "PMD.AvoidPrintStackTrace"})
public final class ServerStopper {
private final static ServerStopper INSTANCE= new ServerStopper();
/**
* Get the singleton ServerStopper
*
* @return the singleton ServerStopper
*/
@CoberturaIgnore
public static ServerStopper getInstance() {
return INSTANCE;
}
/**
* Run the class
*
* @param args The arguments (use pass to connect to server's JMX)
*/
@CoberturaIgnore
public static void main(String[] args) {
String user= null;
String passwd= null;
if (args.length >= 2) {
user= args[1];
if (args.length == 3) {
passwd= args[2];
}
}
ServerStopper stopper= ServerStopper.getInstance();
try {
stopper.stopService(user, passwd);
} catch (Exception e) {
e.printStackTrace();
System.exit(1);
}
System.exit(0);
}
@CoberturaIgnore
void stopService(String user, String passwd)
throws IOException, ConfigurationException, MalformedObjectNameException, InstanceNotFoundException,
IntrospectionException, ReflectionException, MBeanException {
JMXConnector connector= null;
try {
JMXConfig config= new JMXConfig("classpath:jmx.properties");
connector= getRemoteMBeanConnection(config.getServiceUrl(), user, passwd);
MBeanServerConnection remote= connector.getMBeanServerConnection();
ObjectName qName= new ObjectName("addc:*");
Set mBeans= remote.queryMBeans(qName, null);
ObjectInstance shutdown= null;
for (ObjectInstance object : mBeans) {
ObjectName oname= object.getObjectName();
if ("Shutdown".equals(oname.getKeyProperty("SubType"))) {
shutdown= object;
}
}
if (shutdown != null) {
MBeanInfo mbi= remote.getMBeanInfo(shutdown.getObjectName());
MBeanOperationInfo[] operations= mbi.getOperations();
remote.invoke(shutdown.getObjectName(), operations[0].getName(), new Object[0], new String[0]);
}
} finally {
if (connector != null) {
try {
connector.close();
} catch (IOException e) {
assert (e != null);
}
}
}
}
@CoberturaIgnore
private JMXConnector getRemoteMBeanConnection(JMXServiceURL serviceUrl, String user, String passwd)
throws IOException {
Map env= new ConcurrentHashMap<>();
String username= user;
String password= passwd;
if (user == null) {
username= System.console().readLine("User: ");
}
if (passwd == null) {
char[] pwd= System.console().readPassword("Password: ");
password= new String(pwd);
}
String[] credentials= { username, password };
env.put(JMXConnector.CREDENTIALS, credentials);
return JMXConnectorFactory.connect(serviceUrl, env);
}
private ServerStopper() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy