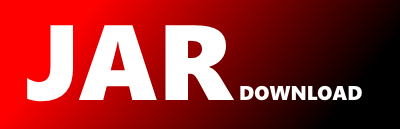
com.adgear.anoa.read.ThriftStreams Maven / Gradle / Ivy
package com.adgear.anoa.read;
import com.adgear.anoa.Anoa;
import com.adgear.anoa.AnoaHandler;
import com.fasterxml.jackson.core.JsonParser;
import org.apache.thrift.TBase;
import org.apache.thrift.protocol.TBinaryProtocol;
import org.apache.thrift.protocol.TCompactProtocol;
import org.apache.thrift.protocol.TJSONProtocol;
import org.apache.thrift.transport.TFileTransport;
import org.apache.thrift.transport.TIOStreamTransport;
import org.apache.thrift.transport.TTransport;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.util.function.Supplier;
import java.util.stream.Stream;
/**
* Utility class for deserializing Thrift records in a {@link java.util.stream.Stream}.
*/
final public class ThriftStreams {
private ThriftStreams() {
}
/**
* Stream from Thrift compact binary representations.
*
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
*/
static public Stream compact(
Supplier supplier,
InputStream inputStream) {
return compact(supplier, new TIOStreamTransport(inputStream));
}
/**
* Stream from Thrift compact binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
* @param Metadata type
*/
static public Stream> compact(
AnoaHandler anoaHandler,
Supplier supplier,
InputStream inputStream) {
return compact(anoaHandler, supplier, new TIOStreamTransport(inputStream));
}
/**
* Stream from Thrift compact binary representations.
*
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
*/
static public Stream compact(
Supplier supplier,
String fileName) {
try {
return compact(supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift compact binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> compact(
AnoaHandler anoaHandler,
Supplier supplier,
String fileName) {
try {
return compact(anoaHandler, supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift compact binary representations.
*
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
*/
static public Stream compact(
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(new TCompactProtocol(tTransport), supplier)
.asStream();
}
/**
* Stream from Thrift compact binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> compact(
AnoaHandler anoaHandler,
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(anoaHandler, new TCompactProtocol(tTransport), supplier)
.asStream();
}
/**
* Stream from Thrift standard binary representations.
*
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
*/
static public Stream binary(
Supplier supplier,
InputStream inputStream) {
return binary(supplier, new TIOStreamTransport(new BufferedInputStream(inputStream)));
}
/**
* Stream from Thrift standard binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
* @param Metadata type
*/
static public Stream> binary(
AnoaHandler anoaHandler,
Supplier supplier,
InputStream inputStream) {
return binary(anoaHandler,
supplier,
new TIOStreamTransport(new BufferedInputStream(inputStream)));
}
/**
* Stream from Thrift standard binary representations.
*
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
*/
static public Stream binary(
Supplier supplier,
String fileName) {
try {
return binary(supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift standard binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> binary(
AnoaHandler anoaHandler,
Supplier supplier,
String fileName) {
try {
return binary(anoaHandler, supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift standard binary representations.
*
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
*/
static public Stream binary(
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(new TBinaryProtocol(tTransport), supplier).asStream();
}
/**
* Stream from Thrift standard binary representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> binary(
AnoaHandler anoaHandler,
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(anoaHandler, new TBinaryProtocol(tTransport), supplier)
.asStream();
}
/**
* Stream from Thrift JSON representations.
*
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
*/
static public Stream json(
Supplier supplier,
InputStream inputStream) {
return json(supplier, new TIOStreamTransport(new BufferedInputStream(inputStream)));
}
/**
* Stream from Thrift JSON representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param inputStream stream from which to deserialize
* @param Thrift record type
* @param Metadata type
*/
static public Stream> json(
AnoaHandler anoaHandler,
Supplier supplier,
InputStream inputStream) {
return json(anoaHandler,
supplier,
new TIOStreamTransport(new BufferedInputStream(inputStream)));
}
/**
* Stream from Thrift JSON representations.
*
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
*/
static public Stream json(
Supplier supplier,
String fileName) {
try {
return json(supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift JSON representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param fileName name of file from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> json(
AnoaHandler anoaHandler,
Supplier supplier,
String fileName) {
try {
return json(anoaHandler, supplier, new TFileTransport(fileName, true));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Stream from Thrift JSON representations.
*
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
*/
static public Stream json(
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(new TJSONProtocol(tTransport), supplier).asStream();
}
/**
* Stream from Thrift JSON representations.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param supplier Thrift record instance supplier
* @param tTransport Thrift TTransport instance from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> json(
AnoaHandler anoaHandler,
Supplier supplier,
TTransport tTransport) {
return LookAheadIteratorFactory.thrift(anoaHandler, new TJSONProtocol(tTransport), supplier)
.asStream();
}
/**
* Stream with 'natural' object-mapping from JsonParser instance.
*
* @param recordClass Thrift record class object
* @param jacksonParser JsonParser instance from which to read
* @param Thrift record type
*/
static public Stream jackson(
Class recordClass,
JsonParser jacksonParser) {
return new ThriftReader<>(recordClass).stream(jacksonParser);
}
/**
* Stream with 'natural' object-mapping from JsonParser instance, with stricter type checking.
*
* @param recordClass Thrift record class object
* @param jacksonParser JsonParser instance from which to read
* @param Thrift record type
*/
static public Stream jacksonStrict(
Class recordClass,
JsonParser jacksonParser) {
return new ThriftReader<>(recordClass).streamStrict(jacksonParser);
}
/**
* Stream with 'natural' object-mapping from JsonParser instance.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param recordClass Thrift record class object
* @param jacksonParser JsonParser instance from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> jackson(
AnoaHandler anoaHandler,
Class recordClass,
JsonParser jacksonParser) {
return new ThriftReader<>(recordClass).stream(anoaHandler, jacksonParser);
}
/**
* Stream with 'natural' object-mapping from JsonParser instance, with stricter type checking.
*
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param recordClass Thrift record class object
* @param jacksonParser JsonParser instance from which to read
* @param Thrift record type
* @param Metadata type
*/
static public Stream> jacksonStrict(
AnoaHandler anoaHandler,
Class recordClass,
JsonParser jacksonParser) {
return new ThriftReader<>(recordClass).streamStrict(anoaHandler, jacksonParser);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy