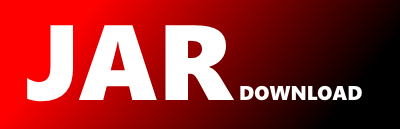
com.adgear.anoa.write.JacksonEncoders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anoa-tools Show documentation
Show all versions of anoa-tools Show documentation
Additional functionality complementing the anoa-core module, requiring additional upstream
dependencies such as jackson-databind and various jackson dataformats.
package com.adgear.anoa.write;
import com.adgear.anoa.Anoa;
import com.adgear.anoa.AnoaHandler;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.fasterxml.jackson.dataformat.csv.CsvSchema;
import java.util.function.Function;
/**
* Utility class for generating functions for serializing Jackson ObjectNode records. Unless
* specified otherwise, the functions should not be deemed thread-safe.
*/
public class JacksonEncoders {
protected JacksonEncoders() {
}
/**
* @return A function which serializes an ObjectNode into its JSON encoding
*/
static public Function json() {
return toBytes(new JsonConsumers()::to);
}
/**
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param Metadata type
* @return A function which serializes an ObjectNode record into its JSON encoding
*/
static public Function, Anoa> json(
AnoaHandler anoaHandler) {
return toBytes(anoaHandler, new JsonConsumers()::to);
}
/**
* @return A function which serializes an ObjectNode into its CBOR encoding
*/
static public Function cbor() {
return toBytes(new CborConsumers()::to);
}
/**
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param Metadata type
* @return A function which serializes an ObjectNode record into its CBOR encoding
*/
static public Function, Anoa> cbor(
AnoaHandler anoaHandler) {
return toBytes(anoaHandler, new CborConsumers()::to);
}
/**
* @param csvSchema CSV schema specification (separator, etc.)
* @return A function which serializes an ObjectNode into a CSV encoding
*/
static public Function csv(CsvSchema csvSchema) {
return toBytes(new CsvConsumers(csvSchema)::to).andThen(String::new);
}
/**
* @param anoaHandler {@code AnoaHandler} instance to use for exception handling
* @param csvSchema CSV schema specification (separator, etc.)
* @param Metadata type
* @return A function which serializes an ObjectNode record into a CSV encoding
*/
static public Function, Anoa> csv(
AnoaHandler anoaHandler,
CsvSchema csvSchema) {
return toBytes(anoaHandler, new CsvConsumers(csvSchema)::to);
}
static protected Function toBytes(
Function> fn) {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
WriteConsumer wc = fn.apply(baos);
return node -> {
baos.reset();
wc.accept(node);
wc.flushUnchecked();
return baos.toByteArray();
};
}
static protected Function, Anoa> toBytes(
AnoaHandler anoaHandler,
Function> fn) {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
WriteConsumer wc = fn.apply(baos);
return anoaHandler.functionChecked(node -> {
baos.reset();
wc.acceptChecked(node);
wc.flush();
return baos.toByteArray();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy