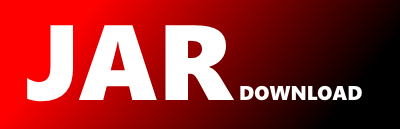
com.adobe.acs.commons.fam.actions.AssetActions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of acs-aem-commons-bundle Show documentation
Show all versions of acs-aem-commons-bundle Show documentation
Main ACS AEM Commons OSGi Bundle. Includes commons utilities.
/*
* ACS AEM Commons
*
* Copyright (C) 2013 - 2023 Adobe
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.adobe.acs.commons.fam.actions;
import static com.adobe.acs.commons.fam.actions.Actions.nameThread;
import org.osgi.annotation.versioning.ProviderType;
import com.adobe.acs.commons.functions.CheckedBiConsumer;
import com.adobe.acs.commons.functions.CheckedBiFunction;
import com.adobe.acs.commons.functions.CheckedConsumer;
import com.adobe.granite.asset.api.Asset;
import com.adobe.granite.asset.api.AssetManager;
import com.adobe.granite.asset.api.Rendition;
import java.util.Iterator;
import org.apache.sling.api.resource.ResourceResolver;
/**
* Assets utility functions.
*/
@ProviderType
public class AssetActions {
private AssetActions() {
// Utility class cannot be instantiated directly.
}
@SuppressWarnings("squid:S3776")
public static final CheckedBiConsumer withAllRenditions(
final CheckedBiConsumer action,
final CheckedBiFunction... filters) {
return (ResourceResolver r, String path) -> {
AssetManager assetManager = r.adaptTo(AssetManager.class);
Asset asset = assetManager.getAsset(path);
for (Iterator extends Rendition> renditions = asset.listRenditions(); renditions.hasNext();) {
Rendition rendition = renditions.next();
boolean skip = false;
if (filters != null) {
for (CheckedBiFunction filter : filters) {
if (!filter.apply(r, rendition.getPath())) {
skip = true;
break;
}
}
}
if (!skip) {
action.accept(r, path);
}
}
};
}
/**
* Remove all renditions except for the original rendition for assets
*
*/
public static final CheckedBiConsumer REMOVE_ALL_RENDITIONS =
(ResourceResolver r, String path) -> {
nameThread("removeRenditions-" + path);
AssetManager assetManager = r.adaptTo(AssetManager.class);
Asset asset = assetManager.getAsset(path);
for (Iterator extends Rendition> renditions = asset.listRenditions(); renditions.hasNext();) {
Rendition rendition = renditions.next();
if (!rendition.getName().equalsIgnoreCase("original")) {
asset.removeRendition(rendition.getName());
}
}
};
/**
* Remove all renditions with a given name
*
*/
public static final CheckedBiConsumer removeAllRenditionsNamed(final String name) {
return (ResourceResolver r, String path) -> {
nameThread("removeRenditions-" + path);
AssetManager assetManager = r.adaptTo(AssetManager.class);
Asset asset = assetManager.getAsset(path);
for (Iterator extends Rendition> renditions = asset.listRenditions(); renditions.hasNext();) {
Rendition rendition = renditions.next();
if (rendition.getName().equalsIgnoreCase(name)) {
asset.removeRendition(rendition.getName());
}
}
};
}
/**
* Remove all non-original renditions from an asset.
*
* @param path
* @return
*/
public static final CheckedConsumer removeRenditions(String path) {
return res -> REMOVE_ALL_RENDITIONS.accept(res, path);
}
/**
* Remove all renditions with a given name
*
* @param path
* @param name
* @return
*/
public static final CheckedConsumer removeRenditionsNamed(String path, String name) {
return res -> removeAllRenditionsNamed(name).accept(res, path);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy