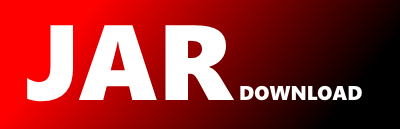
com.adobe.xfa.text.StrItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.text;
import java.util.List;
import com.adobe.xfa.text.markup.MarkupOut;
/**
* @exclude from published api.
*/
abstract class StrItem {
private final int meType;
private int mnCount;
StrItem (int eType) {
meType = eType;
mnCount = 1;
}
void setCount (int nCount) {
mnCount = nCount;
}
// Return the number of poistions occupied by this object.
final int count () {
return mnCount;
}
// Used for building lists/strings of contained objects.
void addField (List oFields) {
}
void addEmbed (List oEmbeds) { /* oEmbeds */
}
TextAttr markup (MarkupOut oMarkup, int nStart, int nSize, boolean bFlattenFields, TextAttr poPrevAttr) {
return poPrevAttr;
}
// Return type or object at a given position.
final int strType () {
return meType;
}
int charAt (int nIndex) {
return '\0';
}
TextAttr attrAt (int nIndex) {
return null;
}
TextField fieldAt (int nIndex) {
return null;
}
TextEmbed embedAt (int nIndex) {
return null;
}
TextNullFrame nullFrameAt (int nIndex) {
return null;
}
// Comparison. Must be equal in class type and contents.
abstract boolean isEqual (StrItem poCompare);
// Handle editing of text and other objects.
StrItem[] split (int nIndex) {
return null;
}
boolean coalesce (StrItem poAfter, int nIndex) {
return false;
}
boolean canCoalesce (StrItem poAfter) {
return false;
}
void delete (int nStart, int nSize) {
}
// Make new object containing full or partial copy of contents.
abstract StrItem cloneItem (TextGfxSource oGfxSource);
StrItem clonePart (TextGfxSource oGfxSource, int nStart, int nSize) {
return cloneItem (oGfxSource);
}
// Special for the handling of attributes.
int attrState () {
return Pkg.ATTR_STATE_NORMAL;
}
void attrState (int eNewState) {
}
void overrideAttr (TextAttr oOverride) {
}
// Attribute pool setting.
void gfxSource (TextGfxSource oGfxSource) {
}
void cascadeLegacyLevel (int eLevel) {
}
// For debugging.
abstract void debug (int indent);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy