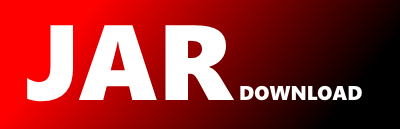
com.adobe.xfa.text.TextEmbed Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.text;
import com.adobe.xfa.gfx.GFXEnv;
import com.adobe.xfa.ut.UnitSpan;
/**
* This class represents an object embedded in a text stream. It serves
* as an abstract base class for application object implementations.
*
*
* The application must derive its own embedded object class(es). It
* can insert instances of its object into a stream as it sees fit.
* Insertion creates a copy; the application continues to own its
* instance and the stream owns the copy. The application must delete
* its own instance but must not delete the copy in the stream.
*
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public abstract class TextEmbed {
public final static int EMBED_AT_BASELINE = 0;
public final static int EMBED_AT_TOP = 1;
public final static int EMBED_AT_BOTTOM = 2;
private TextPosn moPosition = null;
private DispEmbed mpoDispEmbed = null;
/**
* Constructor.
*
* Create an embedded object that is not currently embedded in any
* stream.
*/
public TextEmbed () {
}
/**
* Copy constructor.
*
* Create an embedded object by copying another embedded object. Note
* that the actual embed stream/position is not copied; the object is
* not initially embedded in any stream.
*/
public TextEmbed (TextEmbed oSource) {
}
/**
* Destructor
*
* Destroy the embedded object.
*/
// public void finalize () {
// if (mpoWrapper != null) {
// mpoWrapper.Clear();
// }
// }
/**
* Tell the base class that the object has changed
*
* The derived class must support the ability to make changes through
* the derived object's API. AXTE does not make changes to the object.
* When the derived object has changed, it must tell the base class, in
* order to get the display updated.
* @param bEraseBkgnd Optional: TRUE (default) if the object's
* background is to be erased first; FALSE if not.
*/
public void update (boolean bEraseBkgnd) {
// if (mpoWrapper != null) {
// mpoWrapper.Update (bEraseBkgnd);
// }
}
/**
* Get the object's text context.
*
* The text context allows multiple streams to share common objects that
* are used at layout time, thereby reducing resource usage. This
* method returns a pointer to the text context in effect for this
* embedded object.
* @return Pointer to the text context; NULL if none is in effect.
*/
public TextContext getContext () {
return (moPosition.stream() == null) ? null : moPosition.stream().getContext();
}
/**
* Query the object's position in its owning stream
*
* An embedded object exists at some position in its containing stream.
* This method returns that position.
* @return Text position object which describes both the stream and
* index within that stream (see class TextPosn). If the object
* currently embedded in any stream, the returned position is not
* associated with any stream.
*/
public TextPosn position () {
return moPosition;
}
/**
* Return the graphical extent of the containing text object
* @return A pointer to a const rectangle describing the containing text
* object's extent. If the pointer is NULL, the extent cannot be
* determined.
*/
// public jfRect DispExtent () {
// jfRect poReturn = null;
//
// TextStream poStream = moPosition.Stream();
// if (poStream != null) {
// TextDisplay poDisplay = poStream.Display();
// if (poDisplay != null) {
// TextDispStr poDispStr = poDisplay.Stream();
// if (poDispStr != null) {
// poReturn = poDispStr.Extent();
// }
// }
// }
//
// return poReturn;
// }
/**
* Assignment operator
*
* Copy the content of the embedded object, but not its containing
* stream association.
* @param oSource Source embedded object to copy
*/
public void copyFrom (TextEmbed oSource) {
}
/**
* Pure virtual: Compare embedded objects
*
* The derived class must implement this method. The purpose is to
* compare this embedded object with another for equality.
* @param poCompare Pointer to object to compare against. The derived
* class must cast this to its own type. As long as the derived class
* follows the XTG jfObj convention for object types, it will never be
* called with the wrong type.
* @return TRUE if the objects are equal; FALSE if not.
*/
abstract public boolean isEqual (TextEmbed poCompare);
/**
* Pure virtual: Return the width of the object
* @return The width of the embedded object, in form units.
*/
abstract public UnitSpan width ();
/**
* Pure virtual: Return the height of the object
* @return The height of the embedded object, in form units.
*/
abstract public UnitSpan height ();
/**
* Overridable: Query whether the object enforces its height
*
* This is likely legacy functionality. The user can override the
* default line spacing for the font with a larger amount (e.g., for
* double-space text). An embedded object can suppress that override
* for the line in which it appears, resulting in default line spacing.
* We're not sure why.
* @return TRUE if the line spacing override is to be ignored; FALSE if
* not. The default implementation returns FALSE.
*/
public boolean enforceHeight () {
return false;
}
/**
* Pure virtual: Create a new instance
*
* The derived class must provide an implementation of Clone() that at
* least creates a new object of the correct (derived) type. This will
* be invoked by the parent stream when the caller adds one of its
* objects to the stream, in order to create the copy that the parent
* stream holds on to.
* @return Pointer to cloned copy. Note that this is a true clone that
* must copy all attributes of this embedded object, rather than
* creating an empty new instance.
*/
abstract public TextEmbed cloneEmbed ();
/**
* Pure virtual: Draw the object in the given graphic environment
*
* This method is a call-back that AXTE framework calls to draw the
* object on a given graphic environment. The object may draw itself in
* form co-ordinates, with the origin (0,0) at the top-left corner of
* the extent that the object previously returned by the Width() and
* Height() methods. Alternatively, the object may choose to work in
* device co-ordinates, using the graphic environment and its driver to
* determine the mapping.
* @param oEnv Graphic environment in which to draw the object.
*/
abstract public void gfxDraw (GFXEnv oEnv);
/**
* Overridable: Draw the object with invalidation area
*
* This method allows the object to optimize its drawing by painting
* only the part of it that overlaps a invalidation area. The object
* uses the same co-ordinates as in GfxDraw(). The default
* implementation simply ignores the invalidation area and calls
* GfxDraw().
* @param oEnv Graphic environment in which to draw the object.
* @param oPaintArea Invalidation rectangle in form co-ordinates.
*/
// public void GfxDrawArea (jfGfxEnv oEnv, jfRect oPaintArea) { /* oPaintArea */
// GfxDraw (oEnv);
// }
/**
* Overridable: Draw the object with invalidation area and optimization
* flag
*
* This method allows the object to optimize its drawing by painting
* only the part of it that overlaps a invalidation area, and also to
* apply an optimization flag. The object uses the same co-ordinates as
* in GfxDraw(). The default implementation simply ignores the
* invalidation area and and optimization flag, and just calls
* GfxDraw().
* @param oEnv Graphic environment in which to draw the object.
* @param eOpt Optimization flag. This is intended for embedded
* objects that contain their own text (e.g., barcodes) and controls the
* generation of text runs instead of individual characters.
* @param poPaintArea Invalidation rectangle in form co-ordinates.
* May be NULL, in which case the object must perform a full draw.
*/
// public void GfxDrawOpt (jfGfxEnv oEnv, TextPrefOpt.EnumPref eOpt, jfRect poPaintArea) {
// GfxDraw (oEnv);
// }
/**
* Overridable: Set the object's graphic source
*
* This method allows the derived class to cache graphics source
* information (attribute pools, font mapper) used by the stream
* containing the object. The default implementation does nothing.
* @param oGfxSource Graphic source used by the containing stream.
*/
public void gfxSource (TextGfxSource oGfxSource) {
}
/**
* Overridable: Move the object in a particular environment, due to
* scrolling
*
* The derived class typically provides an implementation for this if it
* creates a window on top of its space in the underlying text. If the
* object simply renders itself through the Gfx package, it need not
* privide an implementation. The default implementation does nothing.
* This method notifies the object that scrolling has caused the object
* to move in a particular graphic enviroment.
* @param poEnv Graphic environment in which the object has moves.
* @param oDisplacement Displacement vector, in form co-ordinates.
*/
// public void AdjustPosition (jfGfxEnv poEnv, jfCoordPair oDisplacement) {
// }
/**
* Overridable: Hide any object-maintained representation of itself
*
* The derived class typically provides an implementation for this if it
* creates a window on top of its space in the underlying text. If the
* object simply renders itself through the Gfx package, it need not
* privide an implementation. The default implementation does nothing.
* This method tells the object to remove any window or other external
* representation of itself.
*/
// public void Hide () {
// }
/**
* Overridable: Notify the object that it has been picked
*
* The AXTE framework calls this method when it detects a pick operation
* within the area occupied by an embedded object. The object may
* choose to act on that event to change its appearance, for example.
* The default implementation sets an internal Pick flag that the AXTE
* framework may subsequently request. Please see the two Picked()
* overloads for more information.
* @param oPickOffset Offset, in form units relative to the object's
* top left corner, where the pick occurred.
* @param poGfxEnv Graphic environment in which the pick occurred.
* @return FALSE if the object rejected the pick operation for some
* reason; TRUE otherwise. The default implementation always returns
* TRUE.
*/
// public boolean Pick (jfCoordPair oPickOffset, jfGfxEnv poGfxEnv) { /* oPickOffset */ /* poGfxEnv */
// mbPicked = true;
// return true;
// }
/**
* Overridable: Query whether the object has been picked
*
* This method works is called by the AXTE framework to determine
* whether the object has been picked. Please see method Pick() for
* more information about the picking operation.
* @return TRUE if the object has been picked; FALSE if not. The
* default implementation returns a flag set by the default Pick()
* implementation and the second Picked() overload.
*/
// public boolean Picked () {
// return mbPicked;
// }
/**
* Overridable: Set/reset the Pick flag
*
* This method is used by the AXTE framework to tell an object that it
* is no longer picked. A derived class can overload this method to
* restore the pre-pick appearance of the object. The default
* implementation simply caches the given flag value.
* @param bPicked New Picked state of the object; TRUE if picked;
* FALSE if not picked.
*/
// public void Picked (boolean bPicked) {
// mbPicked = bPicked;
// }
/**
* Overridable: Query the vertical placement rule for the object
*
* When text and embedded objects are mixed in a stream, embedded
* objects tend to appear bottom-aligned on the text baseline. The
* value EMBED_AT_BASELINE of the class's EmbedAtCode enumeration
* describes this situation However, the object can override this
* default placement in one of two additional ways: EMBED_AT_TOP -
* position at the top of the text line and grow down; or
* EMBED_AT_BOTTOM - position at the bottom (baseline+descent) of
* the text line and grow up.
* @return The embed enumeration value. The default implementation
* returns EMBED_AT_BASELINE.
*/
public int embedAt () {
return EMBED_AT_BASELINE;
}
void position (TextPosn oNewPosition) {
if (oNewPosition == null) {
moPosition = null;
} else {
moPosition = new TextPosn (oNewPosition);
}
}
/**
* Cascade the legacy level.
*
* This method allows the stream/object hierarchy to cascade the legacy
* level to embedded objects. The default implementation ignores the
* call.
*
* @param eLevel - New legacy level.
*/
public void setLegacyLevel (int eLevel) {
}
DispEmbed getDispEmbed () {
return mpoDispEmbed;
}
void setDispEmbed (DispEmbed poWrapper) {
mpoDispEmbed = poWrapper;
}
}