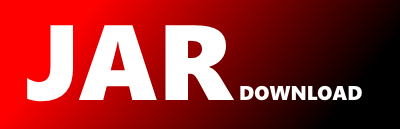
javax.jcr.version.VersionHistory Maven / Gradle / Ivy
/*
* Copyright 2009 Day Management AG, Switzerland. All rights reserved.
*/
package javax.jcr.version;
import javax.jcr.AccessDeniedException;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.ReferentialIntegrityException;
import javax.jcr.RepositoryException;
import javax.jcr.UnsupportedRepositoryOperationException;
/**
* A VersionHistory
object wraps an nt:versionHistory
* node. It provides convenient access to version history information.
*/
public interface VersionHistory extends Node {
/**
* Returns the identifier of the versionable node for which this is the
* version history.
*
* @return the identifier of the versionable node for which this is the
* version history.
* @throws RepositoryException if an error occurs.
* @deprecated As of JCR 2.0, {@link #getVersionableIdentifier} should be
* used instead.
*/
public String getVersionableUUID() throws RepositoryException;
/**
* Returns the identifier of the versionable node for which this is the
* version history.
*
* @return the identifier of the versionable node for which this is the
* version history.
* @throws RepositoryException if an error occurs.
* @since JCR 2.0
*/
public String getVersionableIdentifier() throws RepositoryException;
/**
* Returns the root version of this version history.
*
* @return a Version
object.
* @throws RepositoryException if an error occurs.
*/
public Version getRootVersion() throws RepositoryException;
/**
* This method returns an iterator over all the versions in the line of
* descent from the root version to that base version within this
* history that is bound to the workspace through which this
* VersionHistory
was accessed.
*
* Within a version history H
, B
is the base
* version bound to workspace W
if and only if there exists a
* versionable node N
in W
whose version history
* is H
and B
is the base version of
* N
.
*
* The line of descent from version V1
to
* V2
, where V2
is a successor of V1
,
* is the ordered list of versions starting with V1
and
* proceeding through each direct successor to V2
.
*
* The versions are returned in order of creation date, from oldest to
* newest.
*
* Note that in a simple versioning repository the behavior of this method
* is equivalent to returning all versions in the version history in order
* from oldest to newest.
*
* @return a VersionIterator
object.
* @throws RepositoryException if an error occurs.
*/
public VersionIterator getAllLinearVersions() throws RepositoryException;
/**
* Returns an iterator over all the versions within this version history. If
* the version graph of this history is linear then the versions are
* returned in order of creation date, from oldest to newest. Otherwise the
* order of the returned versions is implementation-dependent.
*
* @return a VersionIterator
object.
* @throws RepositoryException if an error occurs.
*/
public VersionIterator getAllVersions() throws RepositoryException;
/**
* This method returns all the frozen nodes of all the versions in this
* version history in the same order as {@link #getAllLinearVersions}.
*
* @return a NodeIterator
object.
* @throws RepositoryException if an error occurs.
* @since JCR 2.0
*/
public NodeIterator getAllLinearFrozenNodes() throws RepositoryException;
/**
* Returns an iterator over all the frozen nodes of all the versions of this
* version history. Under simple versioning the order of the returned nodes
* will be the order of their creation. Under full versioning the order is
* implementation-dependent.
*
* @return a NodeIterator
object.
* @throws RepositoryException if an error occurs.
* @since JCR 2.0
*/
public NodeIterator getAllFrozenNodes() throws RepositoryException;
/**
* Retrieves a particular version from this version history by version
* name.
*
* @param versionName a version name
* @return a Version
object.
* @throws VersionException if the specified version is not in this version
* history.
* @throws RepositoryException if an error occurs.
*/
public Version getVersion(String versionName) throws VersionException, RepositoryException;
/**
* Retrieves a particular version from this version history by version
* label.
*
* @param label a version label
* @return a Version
object.
* @throws VersionException if the specified label
is not in
* this version history.
* @throws RepositoryException if an error occurs.
*/
public Version getVersionByLabel(String label) throws VersionException, RepositoryException;
/**
* Adds the specified label to the specified version.
*
* The label must be a JCR name in either qualified or expanded form and
* therefore must conform to the syntax restriction that apply to such
* names. In particular a colon (":") should not be used unless it is
* intended as a prefix delimiter in a qualified name.
*
* Adding a version label to a version corresponds to adding a reference
* property with a name specified by the label
parameter to the
* jcr:versionLabels
sub node of the nt:versionHistory
* node. The reference property points to the nt:version
node
* that represents the specified version.
*
* This is a workspace-write method and therefore the change is made
* immediately.
*
* Within a particular version history, a given label may appear a maximum
* of once. If the specified label is already assigned to a version in this
* history and moveLabel
is true
then the label is
* removed from its current location and added to the version with the
* specified versionName
. If moveLabel
is
* false
, then an attempt to add a label that already exists
* will fail.
*
* @param versionName the name of the version to which the label is to be
* added.
* @param label the label to be added, A JCR name in either expanded or
* qualified form.
* @param moveLabel if true
, then if label
is
* already assigned to a version in this version history, it is moved to the
* new version specified; if false
, then attempting to assign
* an already used label will throw a LabelExistsVersionException
.
* @throws LabelExistsVersionException if moveLabel
is
* false
, and an attempt is made to add a label that already
* exists in this version history.
* @throws VersionException if the specified version does not exist in this
* version history or if the specified version is the root version
* (jcr:rootVersion
).
* @throws RepositoryException if another error occurs.
*/
public void addVersionLabel(String versionName, String label, boolean moveLabel) throws LabelExistsVersionException, VersionException, RepositoryException;
/**
* Removes the specified label from among the labels of this version
* history. The label must be a JCR name in either qualified or expanded
* form. This corresponds to removing a property from the
* jcr:versionLabels
child node of the nt:versionHistory
* node that represents this version history.
*
* This is workspace-write method and therefore the change is made
* immediately.
*
* @param label a version label. A JCR name in either expanded or qualified
* form.
* @throws VersionException if the name label does not exist in this version
* history.
* @throws RepositoryException if another error occurs.
*/
public void removeVersionLabel(String label) throws VersionException, RepositoryException;
/**
* Returns true
if any version in the history has the given
* label
. The label must be a JCR name in either qualified or
* expanded form.
*
* @param label a version label. A JCR name in either expanded or qualified
* form.
* @return a boolean
.
* @throws RepositoryException if an error occurs.
*/
public boolean hasVersionLabel(String label) throws RepositoryException;
/**
* Returns true if the given version has the given label
. The
* label must be a JCR name in either qualified or expanded form.
*
* @param version a Version object
* @param label a version label. A JCR name in either expanded or qualified
* form.
* @return a boolean
.
* @throws VersionException if the specified version
is not of
* this version history.
* @throws RepositoryException if another error occurs.
*/
public boolean hasVersionLabel(Version version, String label) throws VersionException, RepositoryException;
/**
* Returns all version labels of the history or an empty array if there are
* none.
*
* @return a String
array containing all the labels of the
* version history.
* @throws RepositoryException if an error occurs.
*/
public String[] getVersionLabels() throws RepositoryException;
/**
* Returns all version labels of the given version
- empty
* array if none. Throws a VersionException
if the specified
* version
is not in this version history.
*
* @param version a Version object
* @return a String
array containing all the labels of the
* given version
* @throws VersionException if the specified version
is not in
* this version history.
* @throws RepositoryException if another error occurs.
*/
public String[] getVersionLabels(Version version) throws VersionException, RepositoryException;
/**
* Removes the named version from this version history and automatically
* repairs the version graph. If the version to be removed is
* V
, V
's predecessor set is P
and
* V
's successor set is S
, then the version graph
* is repaired s follows:
- For each member of
P
, remove
* the reference to V
from its successor list and add
* references to each member of S
. - For each member of
*
S
, remove the reference to V
from its
* predecessor list and add references to each member of P
.
*
Note that this change is made immediately; there is no need to call
* save
. In fact, since the the version storage is read-only
* with respect to normal repository methods, save
does not
* even function in this context.
*
* @param versionName the name of a version in this version history.
* @throws ReferentialIntegrityException if the specified version is
* currently the target of a REFERENCE
property elsewhere in
* the repository (not necessarily in this workspace) and the current
* Session
has read access to that REFERENCE
* property.
* @throws AccessDeniedException if the current Session does not have
* permission to remove the specified version or if the specified version is
* currently the target of a REFERENCE
property elsewhere in
* the repository (not just in this workspace) and the current
* Session
does not have read access to that
* REFERENCE
property.
* @throws UnsupportedRepositoryOperationException
* if this operation is not
* supported by the implementation.
* @throws VersionException if the named version is not in this version
* history.
* @throws RepositoryException if another error occurs.
*/
public void removeVersion(String versionName) throws ReferentialIntegrityException, AccessDeniedException, UnsupportedRepositoryOperationException, VersionException, RepositoryException;
}