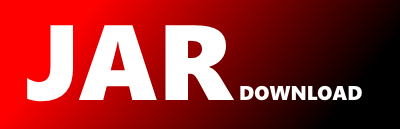
com.adobe.cq.dam.cfm.ContentFragment Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2015 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.adobe.cq.dam.cfm;
import java.util.Calendar;
import java.util.Iterator;
import java.util.Map;
import org.apache.sling.api.adapter.Adaptable;
import org.apache.sling.api.adapter.AdapterFactory;
import org.apache.sling.api.resource.Resource;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import com.day.cq.tagging.Tag;
import aQute.bnd.annotation.ProviderType;
/**
* Implementations of this interface allow to access content fragments through a stable
* API, independently from the actual content structure.
*
* Content fragments consist of one or more elements, which in turn may contain
* variations of their content - for example, a short variation for publishing the
* fragment on Twitter or maybe an on-the-fly variation when the fragment is reused
* on a different page than the original one.
*
* Each variation can be synchronized with the original element content, which in the
* simplest way means that the current content of the element is copied to the variation.
* Depending on the implementation, there might be more sophisticated ways that change
* the original content in some way.
*
* Additionally, each content fragment has a collection of associated content, which
* may contain references to assets, collections or other content fragments.
*
* Each content fragment has a set of meta data, which can be used for determining
* associated content.
*
* Usage pattern: To obtain a ContentFragment, simply adapt a {@link Resource} to
* ContentFragment.class
. This means that each implementing module has to
* provide a {@link AdapterFactory} that adapts Resources
to
* ContentFragment
.
*
* Transactional behavior: The caller is responsible for committing the respective
* {@link org.apache.sling.api.resource.ResourceResolver} after calling one or more
* methods that change a content fragment unless specified otherwise.
*
* In older versions of the API, one element (the "main" or "master" element) was
* considered to define the content fragment. This means, it had a special position within
* the content fragment and usually contained the "most important" or "most distinctive"
* content. With the advent of structured content fragments this is not the case anymore.
*
*/
@ProviderType
public interface ContentFragment extends Adaptable, Versionable {
/**
* Gets an iterator on the templates of predefined content elements of the fragment.
*
* @return The iterator on the content elements
*/
Iterator getElements();
/**
* Determines if the content fragment has an element of the specified name.
*
* @param elementName The name of the element to check
* @return True if there is an element of the provided name
*/
boolean hasElement(String elementName);
/**
* Creates a new element from the specified template.
*
* @param template The element template
* @return The newly created content element
* @throws ContentFragmentException if the change could not be persisted
*/
ContentElement createElement(ElementTemplate template)
throws ContentFragmentException;
/**
* Gets the content element of the specified name.
*
* @param elementName The name of the element; null
or empty string
* for the "main" or "master" element
* @return The element; null
*/
ContentElement getElement(String elementName);
/**
* Gets the (technical) name of the content fragment.
*
* @return The name of the content fragment
*/
String getName();
/**
* Gets the (human-readable) title of the content fragment.
*
* @return The title of the content fragment
*/
String getTitle();
/**
* Sets the (human-readable) title of the content fragment.
*
* @param title The new title
* @throws ContentFragmentException if the change could not be persisted
*/
void setTitle(String title) throws ContentFragmentException;
/**
* Gets the description of the content element.
*
* @return The description of the content element
*/
String getDescription();
/**
* Sets the description of the content fragment.
*
* @param description The new description
* @throws ContentFragmentException if the change could not be persisted
*/
void setDescription(String description) throws ContentFragmentException;
/**
* Gets a map of the fragment's meta data.
*
* @return The map of meta data
*/
Map getMetaData();
/**
* Sets a single meta data property.
*
* As meta data is not limited to the meta data provided through the template,
* this method is generic.
*
* @param name Name of the meta data property
* @param value Value of the meta data property
* @throws ContentFragmentException if the property could not be set/persisted
*/
void setMetaData(String name, Object value) throws ContentFragmentException;
/**
* Gets an iterator on all available variations that are available for the entire
* fragment.
*
* This is used to get a "plain view" on variations, as not all elements may have
* all variations.
*
* The list does not include the "master" or base variation!
*
* @return Iterator on available variations
*/
Iterator listAllVariations();
/**
* Gets the fragment template assigned to this content element.
*
* @return The fragment template
*/
FragmentTemplate getTemplate();
/**
* Creates a new variation.
*
* The variation is added to all elements of the fragment.
*
* The content of each fragment must be initialized with a copy of the element
* content.
*
* Note that the specified (technical) name might not be the final name. In some
* cases - like there is already a variation with the specified name - the
* implementation may change it. To determine the final name of the variation, use the
* {@link VariationTemplate#getName() getName} method of the return value.
*
* Also note that you can pass a {@code null} value for either the {@code name} or
* the {@code title} parameter, but not both.
*
* @param name The (technical) name of the variation to be created; {@code null} if the
* name should be derived from the title
* @param title The (human-readable) title of the variation to be created; may be
* {@code null} if an explicit (technical) name is specified
* @param description The (optional) description of the variation
* @return The intermediate template that represents the newly created variation
* @throws ContentFragmentException if the change could not be persisted
*/
VariationTemplate createVariation(String name, String title, String description)
throws ContentFragmentException;
/**
* Removes the specified global variation from the fragment.
*
* @param name The name of the global variation to remove
* @throws ContentFragmentException if the variation could not be removed properly or
* there is no such variation available
*/
void removeVariation(String name) throws ContentFragmentException;
/**
* Gets an iterator on associated content.
*
* @return Iterator on associated content
*/
Iterator getAssociatedContent();
/**
* Adds the provided {@link Resource} as associated content.
*
* @param content The new associated content
* @throws ContentFragmentException if the resource could not be added
*/
void addAssociatedContent(Resource content) throws ContentFragmentException;
/**
* Removes the provided {@link Resource} as associated content.
*
* @param content The associated content to remove
* @throws ContentFragmentException if the resource could not be removed
*/
void removeAssociatedContent(Resource content) throws ContentFragmentException;
/**
* Returns the date the content fragment was last modified.
*
* @return the last modified date or {@code null} if not available yet
*/
@Nullable
Calendar getLastModifiedDate();
/**
* Returns the most recent modified date of this content fragment or any
* (recursively-referenced) fragment it references.
*
* @return the last recursive modification date or creation date if none available
* @throws ContentFragmentException if the last recursive modification date could not
* be determined correctly
*/
@NotNull
Calendar getLastModifiedDeep() throws ContentFragmentException;
/**
* Sets the passed tags on the fragment. This will delete all existing tags for the fragment and replace them with the passed ones. For
* deleting all tags for the fragment use an empty array.
*
* @param tags The tags to be set on the fragment
* @throws ContentFragmentException if the tags could not be set/persisted
*/
void setTags(@NotNull Tag[] tags) throws ContentFragmentException;
/**
* Gets all the tags associated with this fragment as an array. If no tags are available an empty array is returned.
*
* @return An array with the existing tags or an empty array if no tags exist
* @throws ContentFragmentException if the tags could not be read
*/
@NotNull
Tag[] getTags() throws ContentFragmentException;
/**
* Sets the passed tags on the variation of the fragment. This will delete all existing tags for that variation and replace them with
* the passed ones. For deleting all tags for that variation use an empty array.
*
* @param tags The tags to be set on the fragment
* @param variationName The name of the variation
* @throws ContentFragmentException if the tags could not be set/persisted or if there is no such variation
*/
void setVariationTags(@NotNull Tag[] tags, @NotNull String variationName) throws ContentFragmentException;
/**
* Gets all the tags for the passed variation name as an array. If no tags are available an empty array is returned.
*
* @param variationName The name of the variation
* @return An array with the existing tags or an empty array if no tags exist
* @throws ContentFragmentException if there is no such variation or if the tags could not be read
*/
@NotNull
Tag[] getVariationTags(@NotNull String variationName) throws ContentFragmentException;
}