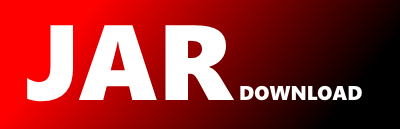
com.adobe.cq.dam.cfm.ContentVariation Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2015 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.adobe.cq.dam.cfm;
import org.jetbrains.annotations.NotNull;
import java.util.Calendar;
import aQute.bnd.annotation.ProviderType;
/**
* Implementations of this interface allow to access a variation of a content element
* through a stable API, agnostic to the underlying content structure.
*
* Variations can be synchronized with some other element. If the implementation does
* not provide enhances synchronization capabilities (which it is free to do), it should
* synchronize with the element content.
*
* The synchronization works by using the synchronization source, taking their content,
* optionally applying some rules and then updating the variation with that content.
*
* How the synchronization source is defined and how the source content is processed is
* implementation specific. This API only provides means to manage the synchronization
* state and triggering the synchronization.
*
* The synchronization state is represented by the {@link SyncStatus} enumeration.
*
* Transactional behavior: The caller is responsible for committing the respective
* {@link org.apache.sling.api.resource.ResourceResolver} after calling one or more
* methods that change a content fragment unless specified otherwise.
*/
@ProviderType
public interface ContentVariation extends Versionable {
/**
* Gets the (technical) name of the variation.
*
* @return The name of the variation
*/
String getName();
/**
* Gets the (human-readable) title of the variation.
*
* @return The title of the variation
*/
String getTitle();
/**
* Sets the current title of the variation in the provided object representation.
*
* @param title The new title of the variation
* @throws ContentFragmentException if the provided value couldn't be persisted
*/
void setTitle(String title) throws ContentFragmentException;
/**
* Gets a description for the variation.
*
* @return The description
*/
String getDescription();
/**
* Sets the current description of the variation in the provided object representation.
*
* @param description The new description of the variation
* @throws ContentFragmentException if the provided value couldn't be persisted
*/
void setDescription(String description) throws ContentFragmentException;
/**
* Gets the current value of the variation in a suitable object representation.
*
* Note that if you change the returned {@link FragmentData}, you will have to
* call {@link #setValue(FragmentData)} explicitly after manipulating the value.
*
* {@link DataType} provides additional information about how values are handled
* by the system.
*
* @return The value
* @since 1.1
*/
FragmentData getValue();
/**
* Sets the current value of the variation in the provided object representation.
*
* Please see {@link DataType} for more information about what "object
* representation" means.
*
* @param object The value
* @throws ContentFragmentException if the provided value couldn't be persisted or
* doesn't match the data type of the variation
* @since 1.1
*/
void setValue(FragmentData object) throws ContentFragmentException;
/**
* Gets the MIME type of the variation.
*
* @return The MIME type; refers to some text format
*/
String getContentType();
/**
* Gets the content of the variation in a suitable text representation.
*
* @return The content of the variation (in a text representation)
*/
String getContent();
/**
* Sets the content of the variation from the provided text value.
*
* The specified content & its MIME type must be textual. What MIME types are
* eventually supported is left to the implementation.
*
* For non-textual data types (added in 1.1), a MIME type of text/plain
* should be specified. The implementation is then responsible for translating the
* provided text value into a suitable object representation (or throw a
* {@link ContentFragmentException} if this is not possible). How the text value is
* converted into a suitable object value is left to the implementation. But it is
* required that a value returned by {@link #getContent()} can successfully be
* converted by {@link #setContent(String, String)}. In other words,
* {@code el.setContent(el.getContent(), el.getContentType()} must not throw a
* {@code ContentFragmentException} because of type conversion problems.
*
* @param content The content
* @param contentType The MIME type of the content
* @throws ContentFragmentException if the content could not be written or the provided
* text could not be converted to the data type of the
* underlying element correctly
* @throws IllegalArgumentException if the specified MIME type is not supported or not
* a textual MIME type
*/
void setContent(String content, String contentType) throws ContentFragmentException;
/**
* Gets the synchronization status of the variation.
*
* @return The synchronization state of the provided variation
*/
SyncStatus getSyncStatus();
/**
* Synchronizes the variation.
*
* @throws ContentFragmentException if the synchronization failed
*/
void synchronize() throws ContentFragmentException;
/**
* Gets the creation date for the variation.
*
* @return the creation date for the variation
*/
@NotNull
Calendar getCreated();
}