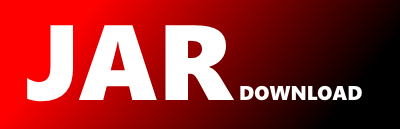
com.adobe.cq.dam.download.api.DownloadService Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2020 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
*/
package com.adobe.cq.dam.download.api;
import org.apache.sling.api.resource.ResourceResolver;
import org.osgi.annotation.versioning.ProviderType;
import com.adobe.cq.dam.download.spi.DownloadTargetProcessor;
/**
*
* A service that allows consumers to specify a list of targets, which will be translated
* into one or more URIs that the consumer can then use to download binaries representing
* the requested targets.
*
*
* The service works asynchronously, which means that the consumer will need to check with
* the service to see if the URIs are available yet. The initial request to generate the
* URIs will return immediately with an identifer that can be used to check the current
* status of the URI creation process.
*
*
* Usage
*
* A basic algorithm for using the service may be as follows:
*
*
* - Build a list of targets for which the service should provide URIs.
* - Request the targets from the service and remember the download ID.
* - Use the provided download ID to poll the service until the operation is complete.
* - If successful, use the provided URIs to retrieve the requested binaries.
* - If unsuccessful, handle the failures accordingly.
*
*
* Example
*
* Sample code for consuming the service may resemble the following.
*
*
* @Component
* public class MyDownloader {
*
* @Reference
* private DownloadService downloadService;
*
* @Reference
* private DownloadApiFactory apiFactory;
*
* public void getDownloads(ResourceResolver resolver) throws DownloadException {
* // add target parameters needed for an asset. See DownloadTargetProcessor
* // documentation for additional details.
* Map<String, Object> assetParameters = new HashMap<String, Object>();
* assetParameters.put("path", "/content/dam/myasset.jpg");
*
* // create a manifest for specifying the targets to download
* DownloadManifest manifest = apiFactory.createDownloadManifest();
*
* // add one or more targets to the manifest. in this case we're downloading a single asset
* manifest.addTarget(apiFactory.createDownloadTarget("asset", new ValueMapDecorator(assetParameters)));
*
* // request the download and remember the ID
* String downloadId = downloadService.download(manifest, resolver);
*
* // NOTE: this construct is for example purposes only, and should not be used as-is.
* // instead, utilize a non-blocking polling mechanism
* boolean finished = false;
* while (!finished) {
* // please don't do this :)
* sleep(5000);
*
* // retrieve the current status of the download
* DownloadProgress progress = downloadService.getProgress(downloadId, resolver);
*
* // note that DownloadProgress can be used to retrieve various pieces of information
* // about the operation, including the number of items being included and the
* // current percent complete
* if (progress.isComplete()) {
* // the process has finished
* finished = true;
*
* // retrieve successfully generated URIs
* for (DownloadArtifact artifact : progress.getArtifacts()) {
* if (artifact.getBinaryURI() != null) {
* // execute code to request the URI and process its binary data
* doSomethingWithURI(artifact.getBinaryURI());
* } else {
* handleFailure(artifact.getFailureReason());
* }
* }
* }
* }
* }
* }
*
*
* Customization
*
*
* The service provides the ability to register additional {@link DownloadTargetProcessor}
* implementations that can handle custom {@link DownloadTarget} types. The process is as simple
* as providing a new OSGI Component that implements the processor interface.
*
*
* Customization Example
*
*
* The following is a simplified illustration of how a new {@link DownloadTargetProcessor}
* might work.
*
*
*
* @Component
* public class MyCustomTargetProcessor implements DownloadTargetProcessor {
*
* @Reference
* private DownloadApiFactory apiFactory;
*
* @Override
* public Collection<DownloadFile> processTarget(DownloadTarget target, ResourceResolver resolver) throws DownloadException {
* // use target's parameters. these parameters can be whatever your processor needs.
* int assetSize = target.get("assetSize", Integer.class); // in bytes
* String archivePath = target.get("archivePath", String.class);
*
* // note that the URL should be externally accessible, and should provide
* // the binary for the target asset. downstream entities will use this URL
* // to bundle the asset into the final list of URLs that will be returned
* // by the DownloadService.
* String assetURL = target.get("assetURL", String.class);
*
* HashMap<String, Object> fileParams = new HashMap<>();
* fileParams.put("archivePath", archivePath);
*
* // create a new archive file
* DownloadFile file = apiFactory.createDownloadFile(Optional.of(assetSize), new URI(assetURL), fileParams);
*
* // provide one or more files that are the result of the processing
* List<DownloadFile> files = new ArrayList<DownloadFile>();
* files.add(file);
*
* return files;
* }
*
* @Override
* public String getType() {
* // this indicates that DownloadTarget instances with this type should
* // be passed to this processor.
* return "myCustomType";
* }
* }
*
*
*
* The result of the above implementation is that {@link DownloadTarget} instances with the type
* of "myCustomType" will be routed to this processor implementation.
*
*
* @see DownloadManifest
* @see DownloadTarget
* @see DownloadException
* @see DownloadTargetProcessor
* @see DownloadProgress
*/
@ProviderType
public interface DownloadService {
/**
*
* Initiates the process of generating a list of URIs for retrieving the binaries of
* a requested set of targets.
*
*
* The operation is asynchronous, so this method will return an identifier that should be used
* to request the status of the operation. See {@link #getProgress(String, ResourceResolver)}.
*
* @param download Specifies which targets should be included in the download. Target types may vary
* and are customizable, but some standard types include folders, assets, or renditions.
* @param resourceResolver Will be used by the service to retrieve Resource information that may be
* required to determine which binaries to include in the download.
* @return An identifier for checking the status of the operation.
* @throws DownloadException Will be thrown if there are failures while trying to initiate the operation.
* Note that failures encountered during the operation will be reported as part of the status.
* @see DownloadManifest
* @see DownloadException
* @see org.apache.sling.api.resource.ResourceResolver
*/
String download(DownloadManifest download, ResourceResolver resourceResolver) throws DownloadException;
/**
*
* Retrieves the current progress of a requested download creation process.
*
*
* In general, the download operation has 3 major states:
*
*
* - The operation is in-progress, and the artifacts are still being generated.
* - The process finished successfully, and the artifacts are ready.
* - The request failed, and error information is available.
*
*
* Use this method to determine the current state of a download request.
*
* @param downloadId The ID of the download to check. This value is provided by the {@link #download(DownloadManifest, ResourceResolver)}
* method.
* @param resourceResolver Will be used to authorize the user and look up status information.
* @return The current progress of the download request. Use this to check if the download is complete,
* retrieve percent complete, get failure information, or retrieve the download URIs.
* @throws DownloadException Thrown if the ID doesn't exist or if there are issues retrieving status information.
* @see DownloadProgress
* @see DownloadException
*/
DownloadProgress getProgress(String downloadId, ResourceResolver resourceResolver) throws DownloadException;
/**
*
* Retrieves the ids of all downloads accessible by the current user, and which he can request the Progress of.
*
* @param resourceResolver the user's resource resolver
* @return the String download ids which the user has access to.
* @throws DownloadException Thrown if there are issues retrieving the IDs.
*/
Iterable getDownloadIds(ResourceResolver resourceResolver) throws DownloadException;
/**
*
* Retrieves the currently available DownloadTargetProcessors.
*
* @return the available DownloadTargetProcessors.
*/
Iterable getDownloadTargetProcessors();
}