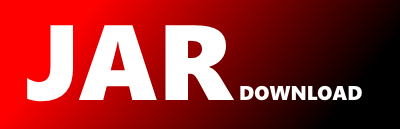
com.adobe.cq.dam.download.api.DownloadStorageService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2020 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
*/
package com.adobe.cq.dam.download.api;
import java.net.URI;
import java.util.Collection;
import java.util.Map;
import org.apache.sling.api.resource.ResourceResolver;
import org.osgi.annotation.versioning.ProviderType;
/**
* This service is responsible for persisting download information for a
* {@link DownloadService}. Provides the ability of creating a new download,
* as well as retrieving information for existing downloads.
*/
@ProviderType
public interface DownloadStorageService {
/**
* Creates and persists a new download, returning the ID of the newly generated item.
* @param resourceResolver Used to persist data and determine the current user.
* @return The ID of the new download.
* @throws DownloadException Thrown if there are issues creating the download.
*/
String createDownload(ResourceResolver resourceResolver) throws DownloadException;
/**
* Creates and persists a new download, returning the ID of the newly generated item.
* @param resourceResolver Used to persist data and determine the current user.
* @param parameters to apply to download process
* @return The ID of the new download.
* @throws DownloadException Thrown if there are issues creating the download.
*/
String createDownload(ResourceResolver resourceResolver, Map parameters) throws DownloadException;
/**
* Adds an artifact to an existing download. The artifact is the final item that will
* be returned as one of the service's download URIs, as specified in {@link DownloadProgress}.
* @param downloadId The download to which the new artifact should be added.
* @param artifactName The name of the artifact that will be added.
* @param asyncJobId The ID of the job that is generating this specific artifact. Can be
* used to identify this particular artifact within the download.
* @param files List of all of the files that will be included in the artifact.
* @param resolver Used to interact with the repository.
* @throws DownloadException Thrown if there are issues peristing the data.
*/
void addDownloadArtifact(String downloadId, String artifactName, String asyncJobId,
Collection files, ResourceResolver resolver) throws DownloadException;
/**
* Retrieves the current progress of a given download ID.
* @param downloadId The ID of the download whose status should be retrieved.
* @param resourceResolver Used to retrieve download information.
* @return Progress of a current download.
* @throws DownloadException Thrown if there are issues retrieving data.
*/
DownloadProgress getProgress(String downloadId, ResourceResolver resourceResolver) throws DownloadException;
/**
* Retrieves all download IDs available to a given user.
* @param resourceResolver User information will be used to retrieve data.
* @return List of download IDs.
* @throws DownloadException Thrown if there are issues retrieving the IDs.
*/
Collection getDownloadIds(ResourceResolver resourceResolver) throws DownloadException;
/**
* Purges the download identified by the given download ID (assuming the current user has permissions to do so)
* @param downloadId The ID of the download whose status should be purged.
* @param resourceResolver Used to retrieve download information.
* @throws DownloadException Thrown if there are issues purging data.
*/
void purgeDownload(String downloadId, ResourceResolver resourceResolver) throws DownloadException;
/**
* Adds access to the download identified by the given downloadId for the user names passed
* @param downloadId The ID of the download whose permissions should be extended.
* @param usernames Users that will be granted access to the download.
* @param resourceResolver Used to retrieve download information.
* @throws DownloadException Thrown if there are issues modifying permissions.
*/
void addUserAccess(String downloadId, String[] usernames, ResourceResolver resourceResolver) throws DownloadException;
/**
* Add the passed parameters to the passed download
* @param downloadId The ID of the download to which the parameters should be added.
* @param parameters The parameters to add; note, only supported parameters will be added.
* @param resourceResolver Used to retrieve download information.
* @throws DownloadException Thrown if there are issues modifying parameters.
*/
void addParameters(String downloadId, Map parameters, ResourceResolver resourceResolver) throws DownloadException;
/**
* Get the parameters of the passed download
* @param downloadId The ID of the download to which the parameters should be returned.
* @param resourceResolver Used to retrieve download information.
* @return A map of the parameters
* @throws DownloadException Thrown if there are issues reading parameters.
*/
Map getParameters(String downloadId, ResourceResolver resourceResolver) throws DownloadException;
/**
* Complete an archive which has been sent for post processing.
* This will trigger notifications containing the download url (unless notifications are suppressed).
* @param downloadId The ID of the download to complete.
* @param artifactId The ID of the artifact (within the download) to complete.
* @throws DownloadException Thrown if there are issues completing the post-processing stage
*/
void completePostProcessing(String downloadId, String artifactId) throws DownloadException;
/**
* Complete an archive which has been sent for post processing, overriding download url with the value provided.
* This will trigger notifications with the updated download url (unless notifications are suppressed).
* @param downloadId The ID of the download to complete.
* @param artifactId The ID of the artifact (within the download) to complete.
* @param binaryURL A {@link URI} representing the download url to use in-preference to the current artifact download link
* @throws DownloadException Thrown if there are issues completing the post-processing stage
*/
void completePostProcessing(String downloadId, String artifactId, URI binaryURL) throws DownloadException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy