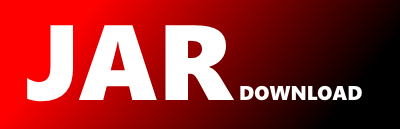
com.adobe.cq.inbox.api.preferences.domain.outofoffice.OOOWfModelDesignate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
*/
package com.adobe.cq.inbox.api.preferences.domain.outofoffice;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.List;
/**
* Class for the AEM Inbox Out Of Office Workflow Model Designate.
* It represents who will be assignee for which workflow model along with
* the excluded workflow models when user is out of office.
*/
public final class OOOWfModelDesignate {
private List wfModels;
private List wfModelException;
private String outOfOfficeDesignate;
/**
* A constant to specify to include
* all the current workflow models in the wfModels list.
*/
public static final String ALL_WORKFLOW_MODELS = "ALL_WORKFLOWS";
/**
* Returns the designated user when user is out of office
* @return The user id of the designated user
*/
@Nonnull public String getOutOfOfficeDesignate(){
return outOfOfficeDesignate;
}
/**
* Sets the designated user id when user is out of office
* @param outOfOfficeDesignate designated user id
*/
public void setOutOfOfficeDesignate(@Nonnull String outOfOfficeDesignate){
this.outOfOfficeDesignate = outOfOfficeDesignate;
}
/**
* Returns the list of workflow model id's for which task is delegated when user is out of office
* @return The list of workflow model id's for which task is delegated when user is out of office
*/
@Nonnull public List getWfModels() {
return wfModels;
}
/**
* Sets the list of workflow model id's for which task is delegated when user is out of office
* @param wfModels the list of workflow model id's. If it contains ALL_WORKFLOW_MODELS
then designated user will be set for all the workflow models
*/
public void setWfModels(@Nonnull List wfModels) {
this.wfModels = wfModels;
}
/**
* Returns the excluded workflow model id's when user is out of office
* or null if excluded workflow model id's are not present.
* This is applicable only when workflow model contain ALL_WORKFLOW_MODELS
.
* @return The excluded workflow model id's when user is out of office
*/
@Nullable public List getExcludedWfmodels() {
return wfModelException;
}
/**
* Sets the excluded workflow model id's when user is out of office
* or null if excluded workflow model id's are not present.
* @param excludedWfmodels the excluded workflow model id's when user is out of office
*/
public void setExcludedWfmodels(@Nullable List excludedWfmodels) {
this.wfModelException = excludedWfmodels;
}
/**
* Constructor for AEM Inbox Out Of Office Workflow Model Designate.
* @param outOfOfficeDesignate designated user id
* @param wfModels the list of workflow model id's. If it contains ALL_WORKFLOW_MODELS
then designated user will be set for all the workflow models
* @param excludedWfmodels the excluded workflow model id's when user is out of office
*/
public OOOWfModelDesignate(@Nonnull String outOfOfficeDesignate,@Nonnull List wfModels, @Nullable List excludedWfmodels){
this.outOfOfficeDesignate = outOfOfficeDesignate;
this.wfModels = wfModels;
this.wfModelException = excludedWfmodels;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy