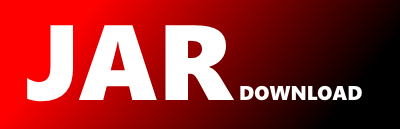
com.adobe.cq.projects.api.Project Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.cq.projects.api;
import org.apache.sling.api.adapter.Adaptable;
import org.apache.sling.api.resource.Resource;
import java.io.InputStream;
import java.util.Collection;
import java.util.List;
import java.util.Set;
/**
* Defines the interface for a CQ Project.
* Note that all changes are transient and need to be committed by the caller, see {@link ProjectManager} for details.
*/
public interface Project extends Adaptable {
/**
* Returns the title of the project.
* @return the title of the project.
*/
String getTitle();
/**
* Set a new title for the project.
* @param name the new name for the project
* @throws ProjectException if the operation fails
*/
void setTitle(String name);
/**
* Returns the description of the project.
* @return the description of the project.
*/
String getDescription();
/**
* Set a new description for the project.
* @param description the new description for the project
* @throws ProjectException if the operation fails
*/
void setDescription(String description);
/**
* Creates a new link or updates an existing link for the specified target.
* @param name the suggested name of the new link.
* @param target the target path or url of this link.
* @return the resource representing the link
* @throws ProjectException if the operation fails
*/
ProjectLink addLink(String name, String target);
/**
* Returns the links that are associated with the project.
* @return an iterator of the links associated with this project.
* @see ProjectLink
*/
Iterable getLinks();
/**
* The Asset folder associated with this project.
* This folder is used when new assets are uploaded through the project interface.
* @return a {@link Resource} for the Asset folder associated with this project.
*/
Resource getAssetFolder();
/**
* Associate a new image to this project's cover.
* This call will replace the exist cover image asset and add it to the
* asset resource collection.
* @param mimeType the mime type of the provided input stream
* @param stream a stream of image data.
* @throws ProjectException if updating the cover image fails.
*/
void setProjectCover(String mimeType, InputStream stream);
/**
* Returns the resource representing the project's cover image.
* @return a image asset resource, or null if there is no cover image specified.
*/
Resource getProjectCover();
/**
* Update the Project's members with the set of users and their associated roles.
* @param userIds a list of user ids
* @param roleIds a list of role ids
* @return the updated set of members.
* @throws ProjectException if there are issues updating the members of the team.
*/
Collection updateMembers(List userIds, List roleIds);
/**
* Return the members associated with the Project.
* @return a set of of {@link ProjectMember}s.
* @throws ProjectException if there are issues obtaining the members of the {@link Project}.
*/
Set getMembers();
/**
* specifies if a project is active
* @param active true when project is active
*/
void setActive(boolean active);
/**
* indicates if the project is active
* @return true when project is active
*/
boolean isActive();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy