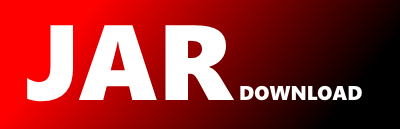
com.adobe.fontengine.SparseArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: SparseArray.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine;
import java.io.Serializable;
import java.util.AbstractList;
import java.util.HashMap;
/**
* SparseArray
*/
// TODO_sgill - better implementation
final public class SparseArray extends AbstractList implements Serializable
{
/* Serialization signature is explicitly set and should be
* incremented on each release to prevent compatibility.
*/
static final long serialVersionUID = 1;
HashMap entries;
public SparseArray() {
super();
entries = new HashMap();
}
public Object get(int index)
{
Entry entry = (Entry) entries.get(new Integer(index));
return entry == null ? null : entry.value;
}
public int size()
{
return entries.size();
}
public void add(int index, Object element)
{
entries.put(new Integer(index), new Entry(index, element));
}
public Object remove(int index)
{
return entries.remove(new Integer(index));
}
public Object set(int index, Object element)
{
Entry entry = (Entry) entries.get(new Integer(index));
Object originalElement = entry.value;
entry.value = element;
return originalElement;
}
public boolean equals(Object obj)
{
if (obj == null)
{
return false;
}
if (obj == this)
{
return true;
}
if (!(obj instanceof SparseArray))
{
return false;
}
return entries.equals(((SparseArray) obj).entries);
}
public int hashCode()
{
return entries.hashCode();
}
static private class Entry implements Serializable
{
static final long serialVersionUID = 1;
int index;
Object value;
Entry(int index, Object value)
{
this.index = index;
this.value = value;
}
public boolean equals(Object obj)
{
if (obj == null)
{
return false;
}
if (obj == this)
{
return true;
}
if (!(obj instanceof Entry))
{
return false;
}
return value.equals(((Entry) obj).value);
}
public int hashCode()
{
return value.hashCode();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy