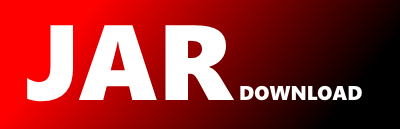
com.adobe.fontengine.font.EmbeddingPermission Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: EmbeddingPermission.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
import java.util.Comparator;
import java.util.Arrays;
import java.util.regex.Pattern;
/** Constants for embedding permissions.
*/
final public class EmbeddingPermission {
private static class XUID
{
final int organization;
final int uid2;
final int uid3;
XUID(int organization, int uid2,int uid3)
{
this.organization = organization;
this.uid2 = uid2;
this.uid3 = uid3;
}
}
private static class XUIDComparator implements Comparator
{
public int compare(Object o1, Object o2)
{
XUID x1 = (XUID)o1;
XUID x2 = (XUID)o2;
if (x1.organization != x2.organization)
return x1.organization - x2.organization;
if (x1.uid2 != x2.uid2)
return x1.uid2 - x2.uid2;
return x1.uid3 - x2.uid3;
}
}
private static final XUIDComparator comparator = new XUIDComparator();
/* This XUID list was copied from CoolType */
private static XUID xuidEmbedOKTableAll[] =
{
new XUID( 1, 11, 1800000), /* Munhwa MunhwaGothic-Regular */
new XUID( 1, 11, 1802000), /* TypeBank TypeBankM-e */
new XUID( 1, 11, 1803100), /* TypeBank TypeBankM-de */
new XUID( 1, 11, 1804200), /* TypeBank TypeBankG-e */
new XUID( 1, 11, 1805300), /* TypeBank TypeBankMaruG-r */
new XUID( 1, 11, 1806400), /* TypeBank TBKomachiRG-R */
new XUID( 1, 11, 1807500), /* TypeBank TBKoudoukenRG-R */
new XUID( 1, 11, 1808600), /* TypeBank TBRyokanRG-R */
new XUID( 1, 11, 1809700), /* TypeBank TBTsukijiRG-R */
new XUID( 1, 11, 1810800), /* TypeBank TBYukinariRG-R */
new XUID( 1, 11, 1811900), /* TypeBank TBKomachiG-E */
new XUID( 1, 11, 1813000), /* TypeBank TBKoudoukenG-E */
new XUID( 1, 11, 1814100), /* TypeBank TBRyokanG-E */
new XUID( 1, 11, 1815200), /* TypeBank TBTsukijiG-E */
new XUID( 1, 11, 1816300), /* TypeBank TBYukinariG-E */
new XUID( 1, 11, 1817400), /* TypeBank TBKomachiM-E */
new XUID( 1, 11, 1818500), /* TypeBank TBKoudoukenM-E */
new XUID( 1, 11, 1819600), /* TypeBank TBRyokanM-E */
new XUID( 1, 11, 1820700), /* TypeBank TBTsukijiM-E */
new XUID( 1, 11, 1821800), /* TypeBank TBYukinariM-E */
new XUID( 1, 11, 1822900), /* TypeBank TBKomachiM-DE */
new XUID( 1, 11, 1824000), /* TypeBank TBKoudoukenM-DE */
new XUID( 1, 11, 1825100), /* TypeBank TBRyokanM-DE */
new XUID( 1, 11, 1826200), /* TypeBank TBTsukijiM-DE */
new XUID( 1, 11, 1827300), /* TypeBank TBYukinariM-DE */
new XUID( 1, 11, 1828500), /* Adobe KozMin-ExtraLight */
new XUID( 1, 11, 1829600), /* Adobe KozMin-Heavy */
new XUID( 1, 11, 1830700), /* Adobe KozMin-Medium */
new XUID( 1, 11, 1837300), /* Adobe KozMin-Light */
new XUID( 1, 11, 1838400), /* Adobe KozMin-Regular */
new XUID( 1, 11, 1839500), /* Adobe KozMin-Bold */
new XUID( 1, 11, 2219600), /* Heisei HeiseiMin-W3H */
new XUID( 1, 11, 2254000), /* Heisei HeiseiMaruGo-W4 Shipping version */
new XUID( 1, 11, 2277900), /* Heisei HeiseiMin-W3 */
new XUID( 1, 11, 2279000), /* Munhwa Munhwa-Regular */
new XUID( 1, 11, 2279400), /* Heisei HeiseiKakuGo-W5 */
new XUID( 1, 11, 2285000), /* Heisei HeiseiMin-W9 */
new XUID( 1, 11, 2294900), /* TypeBank TBKomachiM-hv */
new XUID( 1, 11, 2296000), /* Heisei HeiseiKakuGo-W3 */
new XUID( 1, 11, 2297100), /* Heisei HeiseiKakuGo-W7 */
new XUID( 1, 11, 2298200), /* Heisei HeiseiKakuGo-W9 */
new XUID( 1, 11, 2400000), /* TypeBank TypeBankG-b */
new XUID( 1, 11, 2401100), /* TypeBank TypeBankG-hv */
new XUID( 1, 11, 2402200), /* TypeBank TypeBankM-m */
new XUID( 1, 11, 2403300), /* TypeBank TypeBankM-hv */
new XUID( 1, 11, 2418700), /* TypeBank TBKomachiM-M */
new XUID( 1, 11, 2419800), /* TypeBank TBKomachiG-B */
new XUID( 1, 11, 2420900), /* TypeBank TBKomachiG-hv */
new XUID( 1, 11, 2422000), /* TypeBank TBTsukijiM-hv */
new XUID( 1, 11, 2423100), /* TypeBank TBTsukijiM-M */
new XUID( 1, 11, 2424200), /* TypeBank TBTsukijiG-B */
new XUID( 1, 11, 2425300), /* TypeBank TBTsukijiG-hv */
new XUID( 1, 11, 2426400), /* TypeBank TBRyokanM-hv */
new XUID( 1, 11, 2427500), /* TypeBank TBRyokanM-M */
new XUID( 1, 11, 2428600), /* TypeBank TBRyokanG-B */
new XUID( 1, 11, 2429700), /* TypeBank TBRyokanG-hv */
new XUID( 1, 11, 2430800), /* TypeBank TBYukinariM-hv */
new XUID( 1, 11, 2431900), /* TypeBank TBYukinariM-M */
new XUID( 1, 11, 2433000), /* TypeBank TBYukinariG-B */
new XUID( 1, 11, 2434100), /* TypeBank TBYukinariG-hv */
new XUID( 1, 11, 2446600), /* Heisei HeiseiMin-W5 */
new XUID( 1, 11, 2447700), /* Heisei HeiseiMin-W7 */
new XUID( 1, 11, 2448800), /* TypeBank TBKoudoukenM-M */
new XUID( 1, 11, 2449900), /* TypeBank TBKoudoukenM-hv */
new XUID( 1, 11, 2451000), /* TypeBank TBKoudoukenG-B */
new XUID( 1, 11, 2452100), /* TypeBank TBKoudoukenG-hv */
new XUID( 1, 11, 2453200), /* TypeBank TypeBankG-r */
new XUID( 1, 11, 2454300), /* TypeBank TBKomachiG-R */
new XUID( 1, 11, 2455400), /* TypeBank TBKoudoukenG-R */
new XUID( 1, 11, 2456500), /* TypeBank TBRyokanG-R */
new XUID( 1, 11, 2457600), /* TypeBank TBTsukijiG-R */
new XUID( 1, 11, 2458700), /* TypeBank TBYukinariG-R */
new XUID( 1, 11, 2459800), /* Monotype MSung-Light */
new XUID( 1, 11, 2460900), /* Monotype MSung-Medium */
new XUID( 1, 11, 2461000), /* Monotype MHei-Medium */
new XUID( 1, 11, 2462100), /* Monotype MKai-Medium */
new XUID( 1, 11, 2464300), /* SinoType STHeiti-Regular */
new XUID( 1, 11, 2465100), /* SinoType STSong-Light */
new XUID( 1, 11, 2465900), /* SinoType STKaiti-Regular */
new XUID( 1, 11, 2466700), /* SinoType STFangsong-Light */
new XUID( 1, 11, 2467500), /* TypeBank TypeBankG-db */
new XUID( 1, 11, 2468600), /* TypeBank TypeBankG-m */
new XUID( 1, 11, 2471900), /* TypeBank TBKomachiG-M */
new XUID( 1, 11, 2473000), /* TypeBank TBKoudoukenG-M */
new XUID( 1, 11, 2474100), /* TypeBank TBRyokanG-M */
new XUID( 1, 11, 2475200), /* TypeBank TBTsukijiG-M */
new XUID( 1, 11, 2476300), /* TypeBank TBYukinariG-M */
new XUID( 1, 11, 2477400), /* TypeBank TBKomachiG-DB */
new XUID( 1, 11, 2478500), /* TypeBank TBKoudoukenG-DB */
new XUID( 1, 11, 2479600), /* TypeBank TBRyokanG-DB */
new XUID( 1, 11, 2480700), /* TypeBank TBTsukijiG-DB */
new XUID( 1, 11, 2481800), /* TypeBank TBYukinariG-DB */
new XUID( 1, 11, 2487900), /* Hanyang Hanyang HYKHeadLine-Bold */
new XUID( 1, 11, 2489000), /* Hanyang HYKHeadLine-Medium */
new XUID( 1, 11, 2490100), /* Hanyang HYRGoThic-Medium */
new XUID( 1, 11, 2491200), /* Hanyang HYGoThic-Medium */
new XUID( 1, 11, 2492300), /* Hanyang HYSMyeongJo-Medium */
new XUID( 1, 11, 2493400), /* Hanyang HYGungSo-Bold */
new XUID( 1, 11, 2494100), /* SoftMagic SMGothic-Light */
new XUID( 1, 11, 2494800), /* SoftMagic SMGothic-Medium */
new XUID( 1, 11, 2495500), /* SoftMagic SMGothic-DemiBold */
new XUID( 1, 11, 2496200), /* SoftMagic SMGothic-Bold */
new XUID( 1, 11, 2496900), /* SoftMagic SMMyungjo-Light */
new XUID( 1, 11, 2497600), /* SoftMagic SMMyungjo-Medium */
new XUID( 1, 11, 2498300), /* SoftMagic SMMyungjo-DemiBold */
new XUID( 1, 11, 2499000), /* SoftMagic SMMyungjo-Bold */
new XUID(203, 1, 100), /* Enfour Media Enfour-Mincho-Light */
new XUID(203, 1, 200), /* Enfour Media Enfour-Gothic-Medium */
new XUID(203, 1, 300), /* Enfour Media Enfour-Mincho-Bold */
new XUID(203, 1, 400), /* Enfour Media Enfour-Gothic-Bold */
new XUID(203, 1, 500), /* Enfour Media Enfour-MaruGo-Light */
new XUID(207, 1104, 3033145), /* Enfour Media TypeBankTsukijiM-M */
new XUID(207, 1108, 3136975), /* Enfour Media TypeBankTsukijiM-DE */
new XUID(207, 1109, 3138475), /* Enfour Media TypeBankTsukijiM-E */
new XUID(207, 1111, 3034645), /* Enfour Media TypeBankTsukijiM-Hv */
new XUID(207, 1203, 3167015), /* Enfour Media TypeBankTsukijiG-R */
new XUID(207, 1204, 3036145), /* Enfour Media TypeBankTsukijiG-M */
new XUID(207, 1206, 3139975), /* Enfour Media TypeBankTsukijiG-DB */
new XUID(207, 1207, 3037645), /* Enfour Media TypeBankTsukijiG-B */
new XUID(207, 1209, 3141475), /* Enfour Media TypeBankTsukijiG-E */
new XUID(207, 1211, 3039145), /* Enfour Media TypeBankTsukijiG-Hv */
new XUID(207, 1303, 3168515), /* Enfour Media TypeBankTsukijiRG-R */
new XUID(207, 1311, 3271387), /* Enfour Media TypeBankTsukijiRG-Hv */
new XUID(207, 1504, 3256387), /* Enfour Media TypeBankTsukijiYM-M */
new XUID(207, 1511, 3263887), /* Enfour Media TypeBankTsukijiYM-Hv */
new XUID(207, 2104, 3040645), /* Enfour Media TypeBankKomachiM-M */
new XUID(207, 2108, 3124975), /* Enfour Media TypeBankKomachiM-DE */
new XUID(207, 2109, 3126475), /* Enfour Media TypeBankKomachiM-E */
new XUID(207, 2111, 3042145), /* Enfour Media TypeBankKomachiM-Hv */
new XUID(207, 2203, 3164015), /* Enfour Media TypeBankKomachiG-R */
new XUID(207, 2204, 3043645), /* Enfour Media TypeBankKomachiG-M */
new XUID(207, 2206, 3127975), /* Enfour Media TypeBankKomachiG-DB */
new XUID(207, 2207, 3045145), /* Enfour Media TypeBankKomachiG-B */
new XUID(207, 2209, 3129475), /* Enfour Media TypeBankKomachiG-E */
new XUID(207, 2211, 3046645), /* Enfour Media TypeBankKomachiG-Hv */
new XUID(207, 2303, 3165515), /* Enfour Media TypeBankKomachiRG-R */
new XUID(207, 2311, 3272887), /* Enfour Media TypeBankKomachiRG-Hv */
new XUID(207, 2504, 3257887), /* Enfour Media TypeBankKomachiYM-M */
new XUID(207, 2511, 3265387), /* Enfour Media TypeBankKomachiYM-Hv */
new XUID(207, 3104, 3048145), /* Enfour Media TypeBankYukinariM-M */
new XUID(207, 3108, 3142975), /* Enfour Media TypeBankYukinariM-DE */
new XUID(207, 3109, 3144475), /* Enfour Media TypeBankYukinariM-E */
new XUID(207, 3111, 3049645), /* Enfour Media TypeBankYukinariM-Hv */
new XUID(207, 3203, 3170015), /* Enfour Media TypeBankYukinariG-R */
new XUID(207, 3204, 3051145), /* Enfour Media TypeBankYukinariG-M */
new XUID(207, 3206, 3145975), /* Enfour Media TypeBankYukinariG-DB */
new XUID(207, 3207, 3052645), /* Enfour Media TypeBankYukinariG-B */
new XUID(207, 3209, 3147475), /* Enfour Media TypeBankYukinariG-E */
new XUID(207, 3211, 3054145), /* Enfour Media TypeBankYukinariG-Hv */
new XUID(207, 3303, 3171515), /* Enfour Media TypeBankYukinariRG-R */
new XUID(207, 3311, 3274387), /* Enfour Media TypeBankYukinariRG-Hv */
new XUID(207, 3504, 3259387), /* Enfour Media TypeBankYukinariYM-M */
new XUID(207, 3511, 3266887), /* Enfour Media TypeBankYukinariYM-Hv */
new XUID(207, 4104, 3055645), /* Enfour Media TypeBankRyokanM-M */
new XUID(207, 4108, 3130975), /* Enfour Media TypeBankRyokanM-DE */
new XUID(207, 4109, 3132475), /* Enfour Media TypeBankRyokanM-E */
new XUID(207, 4111, 3057145), /* Enfour Media TypeBankRyokanM-Hv */
new XUID(207, 4203, 3173015), /* Enfour Media TypeBankRyokanG-R */
new XUID(207, 4204, 3058645), /* Enfour Media TypeBankRyokanG-M */
new XUID(207, 4206, 3133975), /* Enfour Media TypeBankRyokanG-DB */
new XUID(207, 4207, 3060145), /* Enfour Media TypeBankRyokanG-B */
new XUID(207, 4209, 3135475), /* Enfour Media TypeBankRyokanG-E */
new XUID(207, 4211, 3061645), /* Enfour Media TypeBankRyokanG-Hv */
new XUID(207, 4303, 3174515), /* Enfour Media TypeBankRyokanRG-R */
new XUID(207, 4311, 3275887), /* Enfour Media TypeBankRyokanRG-Hv */
new XUID(207, 4504, 3260887), /* Enfour Media TypeBankRyokanYM-M */
new XUID(207, 4511, 3268387), /* Enfour Media TypeBankRyokanYM-Hv */
new XUID(207, 5104, 3063145), /* Enfour Media TypeBankKoudoukenM-M */
new XUID(207, 5108, 3148975), /* Enfour Media TypeBankKoudoukenM-DE */
new XUID(207, 5109, 3150475), /* Enfour Media TypeBankKoudoukenM-E */
new XUID(207, 5111, 3064645), /* Enfour Media TypeBankKoudoukenM-Hv */
new XUID(207, 5203, 3176015), /* Enfour Media TypeBankKoudoukenG-R */
new XUID(207, 5204, 3066145), /* Enfour Media TypeBankKoudoukenG-M */
new XUID(207, 5206, 3151975), /* Enfour Media TypeBankKoudoukenG-DB */
new XUID(207, 5207, 3067645), /* Enfour Media TypeBankKoudoukenG-B */
new XUID(207, 5209, 3153475), /* Enfour Media TypeBankKoudoukenG-E */
new XUID(207, 5211, 3069145), /* Enfour Media TypeBankKoudoukenG-Hv */
new XUID(207, 5303, 3177515), /* Enfour Media TypeBankKoudoukenRG-R */
new XUID(207, 5311, 3277387), /* Enfour Media TypeBankKoudoukenRG-Hv */
new XUID(207, 5504, 3262387), /* Enfour Media TypeBankKoudoukenYM-M */
new XUID(207, 5511, 3269887), /* Enfour Media TypeBankKoudoukenYM-Hv */
new XUID(207, 10025, 3290537), /* Enfour Media TyposAlmighty-25 */
new XUID(207, 10026, 3292037), /* Enfour Media TyposAlmighty-26 */
new XUID(207, 10027, 3293537), /* Enfour Media TyposAlmighty-27 */
new XUID(207, 10028, 3295037), /* Enfour Media TyposAlmighty-28 */
new XUID(207, 10029, 3296537), /* Enfour Media TyposAlmighty-29 */
new XUID(207, 10033, 3307037), /* Enfour Media TyposAlmighty-33 */
new XUID(207, 10055, 3308537), /* Enfour Media TyposAlmighty-55 */
new XUID(207, 10077, 3310037), /* Enfour Media TyposAlmighty-77 */
new XUID(207, 10078, 3311537), /* Enfour Media TyposAlmighty-78 */
new XUID(207, 10099, 3313037), /* Enfour Media TyposAlmighty-99 */
new XUID(207, 10210, 3298037), /* Enfour Media TyposAlmighty-210 */
new XUID(207, 10211, 3299537), /* Enfour Media TyposAlmighty-211 */
new XUID(207, 10312, 3301037), /* Enfour Media TyposAlmighty-312 */
new XUID(207, 10314, 3302537), /* Enfour Media TyposAlmighty-314 */
new XUID(207, 10315, 3304037), /* Enfour Media TyposAlmighty-315 */
new XUID(207, 10319, 3305537), /* Enfour Media TyposAlmighty-319 */
new XUID(207, 10910, 3314537), /* Enfour Media TyposAlmighty-910 */
new XUID(207, 11010, 3316037), /* Enfour Media TyposAlmighty-1010 */
new XUID(207, 11111, 3317537), /* Enfour Media TyposAlmighty-1111 */
new XUID(207, 11313, 3319037), /* Enfour Media TyposAlmighty-1313 */
new XUID(207, 11414, 3320537), /* Enfour Media TyposAlmighty-1414 */
new XUID(207, 11515, 3322037), /* Enfour Media TyposAlmighty-1515 */
new XUID(207, 11717, 3323537), /* Enfour Media TyposAlmighty-1717 */
new XUID(207, 12020, 3325037), /* Enfour Media TyposAlmighty-2020 */
};
private static final String[] publicDomainFontNames =
{
"BauerBodoni-Black",
"BauerBodoni-BlackCond",
"BauerBodoni-BlackItalic",
"BauerBodoni-Bold",
"BauerBodoni-BoldCond",
"BauerBodoni-BoldItalic",
"BauerBodoni-BoldItalicOsF",
"BauerBodoni-BoldOsF",
"BauerBodoni-Italic",
"BauerBodoni-ItalicOsF",
"BauerBodoni-Roman",
"BauerBodoni-RomanSC",
"BellCentennial-Address",
"BellCentennial-BoldListing",
"BellCentennial-BoldListingAlt",
"BellCentennial-NameAndNumber",
"BellCentennial-SubCaption",
"BrushScript",
"Candida-Bold",
"Candida-Italic",
"Candida-Roman",
"CenturyOldStyle-Bold",
"CenturyOldStyle-Italic",
"CenturyOldStyle-Regular",
"Charme",
"Courier",
"Courier-Bold",
"Courier-BoldOblique",
"Courier-Oblique",
"Folio-Bold",
"Folio-BoldCondensed",
"Folio-ExtraBold",
"Folio-Light",
"Folio-Medium",
"Futura",
"Futura-Bold",
"Futura-BoldOblique",
"Futura-Book",
"Futura-BookOblique",
"Futura-CondExtraBoldObl",
"Futura-Condensed",
"Futura-CondensedBold",
"Futura-CondensedBoldOblique",
"Futura-CondensedExtraBold",
"Futura-CondensedLight",
"Futura-CondensedLightOblique",
"Futura-CondensedOblique",
"Futura-ExtraBold",
"Futura-ExtraBoldOblique",
"Futura-Heavy",
"Futura-HeavyOblique",
"Futura-Light",
"Futura-LightOblique",
"Futura-Oblique",
"Hobo",
"Impressum-Bold",
"Impressum-Italic",
"Impressum-Roman",
"LetterGothic",
"LetterGothic-Bold",
"LetterGothic-BoldSlanted",
"LetterGothic-Slanted",
"NewsGothic",
"NewsGothic-Bold",
"NewsGothic-BoldOblique",
"NewsGothic-Oblique",
"OCRA",
"OCRB",
"Orator",
"Orator-Slanted",
"PrestigeElite",
"PrestigeElite-Bold",
"PrestigeElite-BoldSlanted",
"PrestigeElite-Slanted",
"Serifa-Black",
"Serifa-Bold",
"Serifa-Italic",
"Serifa-Light",
"Serifa-LightItalic",
"Serifa-Roman",
"SimonciniGaramond",
"SimonciniGaramond-Bold",
"SimonciniGaramond-Italic",
"StempelSchneidler-Black",
"StempelSchneidler-BlackItalic",
"StempelSchneidler-Bold",
"StempelSchneidler-BoldItalic",
"StempelSchneidler-Italic",
"StempelSchneidler-Light",
"StempelSchneidler-LightItalic",
"StempelSchneidler-MedItalic",
"StempelSchneidler-Medium",
"StempelSchneidler-Roman",
"Stencil",
"Weiss",
"Weiss-Bold",
"Weiss-ExtraBold",
"Weiss-Italic"
};
private static final Pattern trademarkAdobe = Pattern.compile("trademark\\s+of\\s+Adobe", Pattern.CASE_INSENSITIVE);
private static final Pattern adobe = Pattern.compile("Adobe", Pattern.CASE_INSENSITIVE);
private EmbeddingPermission () {
// this class provides a name space
}
final static public class SubsettingRestriction {
private SubsettingRestriction () {
// this class provides an enum
}
/** Embedding restriction: must embed the full font */
public static final SubsettingRestriction FULL_FONT_ONLY = new SubsettingRestriction ();
/** Embedding restriction: can embed a subset */
public static final SubsettingRestriction SUBSETTING_OK = new SubsettingRestriction ();
/** Embedding restriction: the font does not express a
* restriction on subsetting. */
public static final SubsettingRestriction UNKNOWN_SUBSETTING_RESTRICTION = new SubsettingRestriction ();
}
final static public class OutlineRestriction {
private OutlineRestriction () {
// this class provides an enum
}
/** Embedding restriction: must embed bitmaps only */
public static final OutlineRestriction BITMAP_ONLY = new OutlineRestriction ();
/** Embedding restriction: can embed outlines */
public static final OutlineRestriction OUTLINES_OK = new OutlineRestriction ();
/** Embedding restriction: the font does not express a
* restriction on outlines (older formats did not support
* this restriction) */
public static final OutlineRestriction UNKNOWN_OUTLINE_RESTRICTION = new OutlineRestriction ();
}
/**
* Interpret the provided fsType according to tech note 5147:
* Font Embedding Guidelines for Adobe Third-party Developers
* @param fsType
* @return the permissions associated with the fsType provided.
*/
static public Permission interpretFSType(int fsType)
{
if (fsType == (1 << 1)) {
return Permission.RESTRICTED;
} else if ((fsType & (1 << 9)) != 0) {
return Permission.RESTRICTED;
} else if ((fsType & (1 << 3)) != 0) {
return Permission.EDITABLE;
} else if ((fsType & (1 << 2)) != 0) {
return Permission.PREVIEW_AND_PRINT;
} else if (fsType == 0) {
return Permission.INSTALLABLE;
} else {
// the remaining cases are
return Permission.ILLEGAL_VALUE;
}
}
static private String getComparisonFontName(String fontname)
{
if (fontname.length() > 7 && fontname.charAt(6) == '+')
{
boolean removeSubsetPrefix = true;
for (int i = 0; i < 6; i++)
{
if (fontname.charAt(i) < 'A' || fontname.charAt(i) > 'Z')
{
removeSubsetPrefix = false;
break;
}
}
if (removeSubsetPrefix)
{
StringBuffer returnName = new StringBuffer(fontname);
return returnName.substring(7);
}
}
return fontname;
}
static public Permission getType1DefaultPermission(String notice, String fontname)
{
if (notice != null)
{
if (trademarkAdobe.matcher(notice).find())
return Permission.EDITABLE;
if (fontname != null)
{
fontname = getComparisonFontName(fontname);
if (adobe.matcher(notice).find()
&& Arrays.binarySearch(EmbeddingPermission.publicDomainFontNames, fontname) >= 0)
return Permission.EDITABLE;
}
}
return Permission.PREVIEW_AND_PRINT;
}
static public Permission getCIDDefaultPermission(int[] xuid)
{
if (xuid == null || xuid.length < 3)
{
return Permission.RESTRICTED;
}
if (Arrays.binarySearch(xuidEmbedOKTableAll, new XUID(xuid[0], xuid[1], xuid[2]), comparator) >= 0)
return Permission.PREVIEW_AND_PRINT;
return Permission.RESTRICTED;
}
static public Permission getTrueTypeDefaultPermission()
{
return Permission.PREVIEW_AND_PRINT;
}
static public Permission getDefaultWasEmbeddedPermission()
{
return Permission.PREVIEW_AND_PRINT;
}
static public Permission getOCFDefaultPermission()
{
return Permission.RESTRICTED;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy