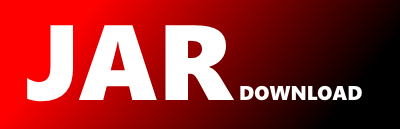
com.adobe.fontengine.font.IteratingOutlineConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.fontengine.font;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* A class that consumes an outline that can later be iterated.
*/
class IteratingOutlineConsumer implements OutlineConsumer {
List elements = new ArrayList();
static final class Element {
Element(ElementType type, double x1, double y1) {
this.type = type;
this.x1 = x1;
this.y1 = y1;
}
Element(double x1, double y1, double x2, double y2) {
this.type = ElementType.quad;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
Element(double x1, double y1, double x2, double y2, double x3, double y3) {
this.type = ElementType.cube;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
this.x3 = x3;
this.y3 = y3;
}
ElementType type;
double x1;
double y1;
double x2;
double y2;
double x3;
double y3;
}
static final class ElementType {
private String type;
private ElementType(String type) {this.type = type;}
public String toString() {return type;}
final static ElementType move = new ElementType("move");
final static ElementType quad = new ElementType("quadratic");
final static ElementType cube = new ElementType("cubic");
final static ElementType line = new ElementType("line");
}
public void curveto(double x2, double y2, double x3, double y3, double x4,
double y4) {
elements.add(new Element(x2, y2, x3, y3, x4, y4));
}
public void curveto(double x2, double y2, double x3, double y3) {
elements.add(new Element(x2, y2, x3, y3));
}
public void endchar() {}
public void lineto(double x, double y) {
elements.add(new Element(ElementType.line, x, y));
}
public void moveto(double x, double y) {
elements.add(new Element(ElementType.move, x, y));
}
public void setMatrix(Matrix newMatrix) {}
Iterator iterator() {
return elements.iterator();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy