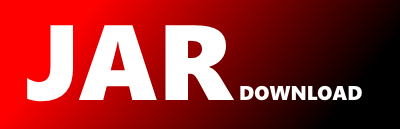
com.adobe.fontengine.font.PDFEncodingBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: PDFEncodingBuilder.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
import com.adobe.fontengine.font.opentype.OpenTypeFont;
import com.adobe.fontengine.font.opentype.PDFEncodingBuilderImpl;
/**
* An object that aids in creation of composite fonts for nonembeddable fonts with
* pdf. This object can enumerate encodings and codepoints are directly mapped to glyphs
* in a font. Only encodings that can be mapped to CMaps that can be used as encodings with
* composite fonts are described.
*/
abstract public class PDFEncodingBuilder {
/**
* Creates an instance of a PDFEncodingBuilder to be used with a given Font and Subset.
* @param font
* @param subset
* @return a PDFEncodingBuilder that can be used with the Font and Subset or null if
* no such encoding builder can be created.
* @throws InvalidFontException
* @throws UnsupportedFontException
*/
public static PDFEncodingBuilder getInstance(Font font, Subset subset)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
FontData data = ((FontImpl)font).getFontData();
if (data instanceof OpenTypeFont && ((OpenTypeFont)data).cmap != null)
return new PDFEncodingBuilderImpl((OpenTypeFont)data, subset);
else
return null;
}
/**
* Fetch the codepoint associated with a glyph in the encoding e.
* @param subsetGlyphID the glyphID in a subset of a font.
* @param e The encoding to fetch. e must have been returned from this.getEncoding
* with the same subsetGlyphID.
* @return the code point.
*/
abstract public int getCodePoint(int subsetGlyphID, Encoding e);
/**
* Fetch the encoding that can be used with a glyph.
* @param subsetGlyphID the glyphID in a subset of the font.
* @return The encoding to be used or null if subsetGlyphID is not directly encoded in the font.
*/
abstract public Encoding getEncoding(int subsetGlyphID);
final public static class Encoding {
private final String description;
private Encoding(String description) {this.description = description;}
/**
* Code points returned in UNICODE are Unicode Scalar Values. A UTF CMap may
* therefore be an appropriate encoding to use in the pdf.
*/
public final static Encoding UNICODE = new Encoding("Unicode Scalar Values");
/**
* Code points are in Microsoft code page 932, so a Japanese CMap may be
* an appropriate encoding.
*/
public final static Encoding MS_SHIFT_JIS = new Encoding("Shift JIS");
/**
* Code points are in Microsoft code page 936, so a Simplified Chinese
* CMap may be an appropriate encoding.
*/
public final static Encoding MS_PRC = new Encoding("PRC");
/**
* Code points are in Microsoft code page 950, so a Traditional Chinese
* CMap may be an appropriate encoding.
*/
public final static Encoding MS_BIG5 = new Encoding("Big 5");
/**
* Code points are in Microsoft Wansung, a subset of Microsoft codepage
* 949, so a Korean CMap may be an appropriate encoding.
*/
public final static Encoding MS_WANSUNG = new Encoding("Wansung");
/**
* Code points are in Microsoft code page 1361, so a Korean CMap that
* covers Johab may be an appropriate encoding.
*/
public final static Encoding MS_JOHAB = new Encoding("Johab");
public String toString() {return description;}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy