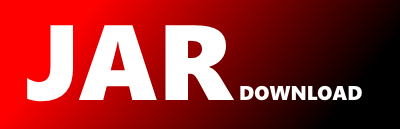
com.adobe.fontengine.font.PDFFontDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: PDFFontDescription.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
import java.io.IOException;
import java.io.OutputStream;
/**
* A helper class useful for building pdf font objects.
*
* Metrics
*
* Metrics returned by this class are expressed in 1/1000th of em.
*
*
Synchronization
*
* This class is immutable after contruction and contains no mutable
* static data. It is therefore threadsafe.
*/
abstract public class PDFFontDescription {
/** Fetches the preferred postscript name for the font. The
* Postscript name is not required, in which case this returns null.
*/
abstract public String getPostscriptName() throws InvalidFontException, UnsupportedFontException;
/** Fetches the preferred family name associated with this font. The
* family name is not required, in which case this returns null.
*/
abstract public String getFontFamily() throws InvalidFontException, UnsupportedFontException;
/**
* Fetch the font's primary vertical stem width
* @return the stemv scaled to 1000 ppem.
*/
abstract public double getStemV() throws UnsupportedFontException, InvalidFontException;
/**
* Fetch the font bounding box. The font bbox is not required
* in fonts, in which case this returns null.
* @return the fontbbox scaled to 1000 ppem.
*/
abstract public Rect getFontBBox() throws InvalidFontException, UnsupportedFontException;
/**
* Fetch the height above the baseline of capital letters
* @return the capHeight scaled to 1000 ppem.
*/
abstract public double getCapHeight () throws UnsupportedFontException, InvalidFontException;
/**
* Fetch the height above the baseline for lowercase letters.
* @return the xHeight scaled to 1000 ppem.
*/
abstract public double getXHeight() throws UnsupportedFontException, InvalidFontException;
/**
* Fetch the italic angle.
*
* This is the angle of the primary vertical strokes in the font given in degrees
* counter clockwise from vertical (e.g. most non-italic fonts will have 0 for their
* italic angle; italic fonts will often have italic angles around -15).
*/
abstract public double getItalicAngle() throws InvalidFontException, UnsupportedFontException;
/**
* The number of glyphs in the font.
*/
abstract public int getNumGlyphs () throws InvalidFontException, UnsupportedFontException;
/**
* Fetch the horizontal advance width for the specified glyph.
* @param glyphID the glyph to be fetched
* @return the advance width scaled to 1000 ppem.
* @throws InvalidGlyphException thrown when the glyph contains data that cannot be interpretted and cannot
* be ignored
* @throws UnsupportedFontException thrown when the font contains data that indicates that AFE does not support
* that class of fonts.
*/
abstract public double getAdvance(int glyphID) throws InvalidFontException, UnsupportedFontException;
/** Get the Registry, Ordering and Supplement associated with the font.
* If the font does not have a ROS, return null. The ROS is described in
* technote 5014: "Adobe CMap and CIDFont Files Specification" found at
* http://partners.adobe.com/public/developer/en/font/5014.CIDFont_Spec.pdf
*/
public abstract ROS getROS()
throws UnsupportedFontException, InvalidFontException;
/**
* Get the CID count associated with the CIDKeyedFont
*/
public abstract int getCIDCount() throws UnsupportedFontException, InvalidFontException;
/**
* Fetch the CID for a given glyph. This function must only be called
* if getROS returns a non-null ROS.
* @param glyphID
* @return the CID associated with glyphID
* @throws UnsupportedFontException
* @throws InvalidFontException
*/
public abstract int getGlyphCid(int glyphID)
throws UnsupportedFontException, InvalidFontException;
public abstract boolean pdfFontIsTrueType()
throws InvalidFontException, UnsupportedFontException;
public abstract String getGlyphName(int gid)
throws InvalidFontException, UnsupportedFontException;
public abstract String getBase14Name();
public abstract boolean isSerifFont()
throws InvalidFontException, UnsupportedFontException;
public abstract boolean isSmallCapFont()
throws InvalidFontException, UnsupportedFontException;
public abstract boolean isAllCapFont()
throws InvalidFontException, UnsupportedFontException;
/**
* Streams a font suitable for embedding in a PDF for editting situations.
* @param out The stream to which the font is streamed.
* @param openTypeFontsAllowed True iff the target pdf can contain OpenType fonts (i.e., it is at least PDF 1.7)
*/
public abstract void stream(OutputStream out, boolean openTypeFontsAllowed)
throws InvalidFontException, UnsupportedFontException, IOException;
public abstract void subsetAndStream(Subset subset, OutputStream out, boolean preserveROS)
throws InvalidFontException, UnsupportedFontException, IOException;
public abstract void subsetAndStream(SubsetSimpleType1 subset, OutputStream out)
throws InvalidFontException, UnsupportedFontException, IOException;
public abstract void subsetAndStream(SubsetSimpleTrueType subset, OutputStream out)
throws InvalidFontException, UnsupportedFontException, IOException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy