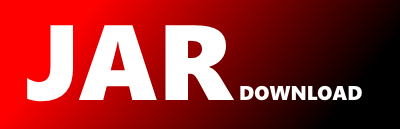
com.adobe.fontengine.font.Rect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: Rect.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
/** A bounding box.
*
* AFE's coordinates are in y-positive.
* Values are: xmin, ymin, xmax, ymax where xmin <= xmax and ymin <= ymax.
*
*
Synchronization
*
* These objects are immutable.
*/
final public class Rect {
public final double xmin;
public final double ymin;
public final double xmax;
public final double ymax;
/** A rectangle with xmin = ymin = xmax = ymax = 0. */
public static final Rect emptyRect = new Rect (0.0d, 0.0d, 0.0d, 0.0d);
public Rect (double xmin, double ymin, double xmax, double ymax) {
this.xmin = xmin;
this.ymin = ymin;
this.xmax = xmax;
this.ymax = ymax;
}
/**
* Create a Rect from an array of doubles.
* @param vals An array of length 4 whose entries are in the order xmin, ymin, xmax, ymax.
*/
public Rect(double[] vals) {
this.xmin = vals[0];
this.ymin = vals[1];
this.xmax = vals[2];
this.ymax = vals[3];
}
/**
* Convert this Rect through a matrix.
* @param m the matrix
* @return the transformed bounding box
*/
public Rect applyMatrix (Matrix m) {
if (m.isIdentity ()) {
return this; }
double tlX, tlY, trX, trY, brX, brY, blX, blY;
tlX = m.applyToXYGetX (xmin, ymax);
tlY = m.applyToXYGetY (xmin, ymax);
trX = m.applyToXYGetX (xmax, ymax);
trY = m.applyToXYGetY (xmax, ymax);
brX = m.applyToXYGetX (xmax, ymin);
brY = m.applyToXYGetY (xmax, ymin);
blX = m.applyToXYGetX (xmin, ymin);
blY = m.applyToXYGetY (xmin, ymin);
return new Rect (
Math.min (Math.min (tlX, trX), Math.min (brX, blX)),
Math.min (Math.min (tlY, trY), Math.min (brY, blY)),
Math.max (Math.max (tlX, trX), Math.max (brX, blX)),
Math.max (Math.max (tlY, trY), Math.max (brY, blY)));
}
public Rect toEmSpace (double unitsPerEmX, double unitsPerEmY) {
return new Rect (xmin / unitsPerEmX, ymin / unitsPerEmY,
xmax / unitsPerEmX, ymax / unitsPerEmY);
}
public Rect toDesignSpace (double unitsPerEmX, double unitsPerEmY) {
return new Rect (xmin * unitsPerEmX, ymin * unitsPerEmY,
xmax * unitsPerEmX, ymax * unitsPerEmY);
}
public String toString() {
return "[ " + Double.toString(xmin) + " " + Double.toString(ymin) + " "
+ Double.toString(xmax) + " " + Double.toString(ymax) + " ]";
}
public boolean equals (Object obj) {
if (obj != null) {
if (this == obj) {
return true; }
else if (obj instanceof Rect) {
Rect r = (Rect) obj;
return ( Double.compare (xmin, r.xmin) == 0
&& Double.compare (ymin, r.ymin) == 0
&& Double.compare (xmax, r.xmax) == 0
&& Double.compare (ymax, r.ymax) == 0); }}
return false;
}
public int hashCode() {
return (int)xmin ^ (int)xmax ^ (int)ymin ^ (int)ymax;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy