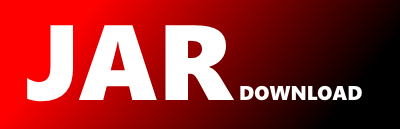
com.adobe.fontengine.font.SubsetDefaultImpl Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* File: SubsetDefaultImpl.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
/** A default implementation for the Subset
interface.
*
*
* This implementation is suitable for subclassing, if specific
* behaviour is needed beyond the correspondance between
* subset and full gids.
*/
public class SubsetDefaultImpl implements Subset {
/** Remember whether this subset is actually the whole font. */
protected final boolean doSubset;
/** The number of glyphs in the subset. */
protected int subsetGlyphCount;
/**
* If subsetted, the mapping from full GID to subset GID. This array has as
* many elements as there are glyphs in the full font. If not subsetted, null,
* which is to be interpreted as the identity mapping.
*/
protected int[] fullGid2subsetGid;
/** If subsetted, the mapping from subset GID to full GID. This array has
* as many elements are there are glyphs in the full font (because potentially
* every glyph can be in the subset), but only the values in the range
* [0..subsetGlyphCount[ are set.
* If not subsetted, null, which is to be interpreted as the
* identify mapping. */
protected int[] subsetGid2fullGid;
/** Invariants:
* If the font is not subsetted, there are no invariants.
* If the font is subsetted, the invariant are informally that
* the two arrays are in sync, and do represent a proper subset;
* more formally:
* - 0 <= subsetGlyphCount <= number of glyphs in font
* - for all i in [0..subsetGlyphCount[, subsetGid2fullGid [i] is in the range [0.. number of glyphs in font[
* - subsetGid2fullGid [0..subsetGlyphCount[ is a set of size subsetSubGlyphCount
* i.e. not two glyphs in the subset map to the same full glyph
* - for all i in [0..subsetGlyphCount[, fullGid2subsetGid [subsetGid2fullGid [i]] = i
*/
/** Synchronization: as methods on a Subset
object are called,
* subsetGlyphCount, (the content of) fullGid2subsetGid and (the content of)
* subsetGid2fullGid are modified. The invariants on those are protected
* by the Subset lock.
*/
/** Create a new subset for a font.
* @param numGlyphs the number of glyphs in the full font
* @param doSubset whether to effectively subset
*/
public SubsetDefaultImpl (int numGlyphs, boolean doSubset)
throws InvalidFontException, UnsupportedFontException {
this.doSubset = doSubset;
if (!doSubset) {
this.subsetGlyphCount = numGlyphs;
this.fullGid2subsetGid = null;
this.subsetGid2fullGid = null; }
else {
this.subsetGlyphCount = 0;
this.fullGid2subsetGid = new int [numGlyphs];
this.subsetGid2fullGid = new int [numGlyphs];
for (int i = subsetGlyphCount; i < numGlyphs; i++) {
fullGid2subsetGid [i] = -1; }}
}
public boolean doSubset()
{
return doSubset;
}
public int getNumGlyphs () {
synchronized (this) {
return subsetGlyphCount; }
}
public int getSubsetGid (int fullGid)
throws InvalidFontException, UnsupportedFontException {
if (doSubset) {
synchronized (this) {
try {
if (fullGid2subsetGid [fullGid] == -1) {
fullGid2subsetGid [fullGid] = subsetGlyphCount;
subsetGid2fullGid [subsetGlyphCount] = fullGid;
subsetGlyphCount++;
pullComponentGlyphs (fullGid); }
} catch (ArrayIndexOutOfBoundsException e) {
throw new InvalidFontException(e); // if we have found a gid beyond what is allowed, it is an invalid font.
}
return fullGid2subsetGid [fullGid]; }}
else {
return fullGid; }
}
public int getFullGid (int subsetGid) {
if (doSubset) {
synchronized (this) {
return subsetGid2fullGid [subsetGid]; }}
else {
return subsetGid; }
}
protected void pullComponentGlyphs (int fullGid)
throws UnsupportedFontException, InvalidFontException {
// subclasses should override if there is a notion of
// component glyph
}
public int getExistingSubsetGid(int fullGid)
{
if (doSubset) {
if (fullGid >= fullGid2subsetGid.length)
return -1;
return fullGid2subsetGid[fullGid];
} else {
return fullGid;
}
}
}