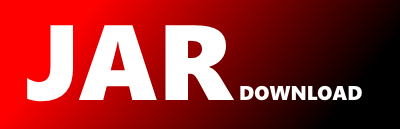
com.adobe.fontengine.font.WrapperFontData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/**
*
*/
package com.adobe.fontengine.font;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Set;
import com.adobe.agl.util.ULocale;
import com.adobe.fontengine.fontmanagement.CacheSupportInfo;
import com.adobe.fontengine.fontmanagement.Platform;
import com.adobe.fontengine.fontmanagement.fxg.FXGFontDescription;
import com.adobe.fontengine.fontmanagement.platform.PlatformFontDescription;
import com.adobe.fontengine.fontmanagement.postscript.PostscriptFontDescription;
import com.adobe.fontengine.inlineformatting.css20.CSS20FontDescription;
import com.adobe.fontengine.inlineformatting.css20.CSS20Attribute.CSSStretchValue;
/**
* @author sgill
*
*/
public class WrapperFontData extends FontData
{
protected FontData fontData;
public WrapperFontData(FontData fontData)
{
super(fontData.getContainerFingerprint());
this.fontData = fontData;
}
public FontData getFontData()
throws InvalidFontException, UnsupportedFontException
{
return fontData;
}
public Subset createSubset()
throws InvalidFontException, UnsupportedFontException
{
return fontData.createSubset();
}
public boolean equals(Object obj)
{
return fontData.equals(obj);
}
public byte[] getContainerFingerprint()
{
return fontData.getContainerFingerprint();
}
public double getCoolTypeCapHeight()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeCapHeight();
}
public Rect getCoolTypeFontBBox()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCoolTypeFontBBox();
}
public Rect getCoolTypeGlyphBBox(int glyphID)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeGlyphBBox(glyphID);
}
public int getCoolTypeGlyphForChar(int unicodeScalarValue)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCoolTypeGlyphForChar(unicodeScalarValue);
}
public Rect getCoolTypeIcfBox()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeIcfBox();
}
public Rect getCoolTypeIdeoEmBox()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeIdeoEmBox();
}
public LineMetrics getCoolTypeLineMetrics()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeLineMetrics();
}
public boolean getCoolTypeProportionalRomanFromFontProperties()
throws InvalidFontException
{
return fontData.getCoolTypeProportionalRomanFromFontProperties();
}
public CoolTypeScript getCoolTypeScript()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeScript();
}
public UnderlineMetrics getCoolTypeUnderlineMetrics()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeUnderlineMetrics();
}
public double getCoolTypeUnitsPerEm()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeUnitsPerEm();
}
public double getCoolTypeXHeight()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCoolTypeXHeight();
}
public CSS20FontDescription[] getCSS20FontDescription()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCSS20FontDescription();
}
public Permission getEmbeddingPermission(boolean wasEmbedded)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getEmbeddingPermission(wasEmbedded);
}
public Rect getFontBBox()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getFontBBox();
}
public FXGFontDescription[] getFXGFontDescription(Platform platform, ULocale locale)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getFXGFontDescription(platform, locale);
}
public Rect getGlyphBBox(int gid)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getGlyphBBox(gid);
}
public int getGlyphForChar(int unicodeScalarValue)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getGlyphForChar(unicodeScalarValue);
}
public void getGlyphOutline(int gid, OutlineConsumer consumer)
throws InvalidFontException, UnsupportedFontException
{
fontData.getGlyphOutline(gid, consumer);
}
public double getHorizontalAdvance(int gid)
throws InvalidGlyphException, UnsupportedFontException, InvalidFontException
{
return fontData.getHorizontalAdvance(gid);
}
public LineMetrics getLineMetrics()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getLineMetrics();
}
public int getNumGlyphs()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getNumGlyphs();
}
public PDFFontDescription getPDFFontDescription(Font font)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getPDFFontDescription(font);
}
public PlatformFontDescription[] getPlatformFontDescription(Platform platform, ULocale locale)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getPlatformFontDescription(platform, locale);
}
public double[] getPointSizeRange()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getPointSizeRange();
}
public PostscriptFontDescription[] getPostscriptFontDescription()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getPostscriptFontDescription();
}
public CSS20FontDescription getPreferredCSS20FontDescription()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getPreferredCSS20FontDescription();
}
public Scaler getScaler()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getScaler();
}
public Scaler getScaler(ScanConverter c)
throws InvalidFontException, UnsupportedFontException
{
return fontData.getScaler(c);
}
public CatalogDescription getSelectionDescription()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getSelectionDescription();
}
public SWFFont4Description getSWFFont4Description(boolean wasEmbedded)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getSWFFont4Description(wasEmbedded);
}
public SWFFontDescription getSWFFontDescription(boolean wasEmbedded)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getSWFFontDescription(wasEmbedded);
}
public double getUnitsPerEmX()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getUnitsPerEmX();
}
public double getUnitsPerEmY()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getUnitsPerEmY();
}
public XDCFontDescription getXDCFontDescription(Font font)
throws UnsupportedFontException, InvalidFontException
{
return fontData.getXDCFontDescription(font);
}
public boolean hasCoolTypeProportionalRoman()
throws InvalidFontException, UnsupportedFontException
{
return fontData.hasCoolTypeProportionalRoman();
}
public int hashCode()
{
return fontData.hashCode();
}
public boolean isSymbolic()
throws UnsupportedFontException, InvalidFontException
{
return fontData.isSymbolic();
}
public void subsetAndStream(Subset subset, OutputStream out, boolean preserveROS)
throws InvalidFontException, UnsupportedFontException, IOException
{
fontData.subsetAndStream(subset, out, preserveROS);
}
public String toString()
{
return fontData.toString();
}
protected Rect getCoolTypeRawFontBBox()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCoolTypeFontBBox();
}
protected Set getCSSFamilyNames()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCSSFamilyNames();
}
protected CSSStretchValue getCSSStretchValue()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCSSStretchValue();
}
protected int getCSSWeight()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getCSSWeight();
}
protected String getPreferredCSSFamilyName()
throws InvalidFontException, UnsupportedFontException
{
return fontData.getPreferredCSSFamilyName();
}
protected boolean isCSSStyleItalic()
throws InvalidFontException, UnsupportedFontException
{
return fontData.isCSSStyleItalic();
}
protected boolean isCSSStyleNormal()
throws InvalidFontException, UnsupportedFontException
{
return fontData.isCSSStyleNormal();
}
protected boolean isCSSStyleOblique()
throws InvalidFontException, UnsupportedFontException
{
return fontData.isCSSStyleOblique();
}
protected boolean isCSSVariantNormal()
throws InvalidFontException, UnsupportedFontException
{
return fontData.isCSSVariantNormal();
}
protected boolean isCSSVariantSmallCaps()
throws InvalidFontException, UnsupportedFontException
{
return fontData.isCSSVariantSmallCaps();
}
public CacheSupportInfo getCacheSupportInfo()
throws UnsupportedFontException, InvalidFontException
{
return fontData.getCacheSupportInfo();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy