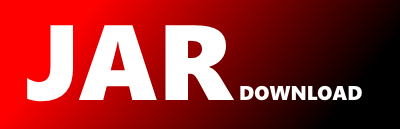
com.adobe.fontengine.font.cff.CFFByteArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: CFFByteArray.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import java.io.IOException;
import com.adobe.fontengine.font.FontByteArray;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
/** An immutable sequence of bytes, with methods to access
* those bytes as basic CFF types.
*
* The return type of the various accessors for the integral
* types is int
whenever the type fits
* in [-2^31 .. 2^31-1], long
otherwise. The
* only exception is getOffset
.
*/
final class CFFByteArray extends FontByteArray {
private CFFByteArray (int size) {
super(size);
}
/** Construct a CFFByteArray
from the data in buffer. This
* takes ownership of the data in 'buffer'. It doesn't copy the data.
*/
public CFFByteArray (FontByteArray buffer)
throws IOException, InvalidFontException, UnsupportedFontException {
super (buffer, false);
}
protected static final CFFByteArrayBuilder getCFFByteArrayBuilderInstance() {
return new CFFByteArrayBuilder();
}
protected static final CFFByteArrayBuilder getCFFByteArrayBuilderInstance(int size) {
return new CFFByteArrayBuilder(size);
}
/** Return the Card8 at offset
.
*/
protected final int getcard8 (int offset) throws InvalidFontException {
if (offset < 0 || offset >= getSize()) {
throw new InvalidFontException("Invalid index"); }
return getRawByte(offset);
}
/** Return the Int8 at offset
.
*/
protected final int getint8 (int offset) throws InvalidFontException {
if (offset < 0 || offset >= getSize()) {
throw new InvalidFontException("Invalid index"); }
return getSignedRawByte(offset);
}
/** Return the Card16 at offset
.
*/
protected final int getcard16 (int offset) throws InvalidFontException {
return (getcard8 (offset) << 8) | getcard8 (offset + 1);
}
/** Return the Int16 at offset
.
*/
protected final int getint16 (int offset) throws InvalidFontException {
return (getint8 (offset) << 8) | getcard8 (offset + 1);
}
/** Return the Card24 at offset
.
*/
protected final int getcard24 (int offset) throws InvalidFontException {
return (getcard8 (offset )) << 16
| (getcard8 (offset + 1)) << 8
| (getcard8 (offset + 2));
}
/** Return the Card32 at offset
(as a long).
*/
protected final long getcard32 (int offset) throws InvalidFontException {
return (getcard8 (offset )) << 24
| (getcard8 (offset + 1)) << 16
| (getcard8 (offset + 2)) << 8
| (getcard8 (offset + 3));
}
/** Return the Int32 at offset
.
*/
protected final int getint32 (int offset) throws InvalidFontException {
return ( getint8 (offset )) << 24
| (getcard8 (offset + 1)) << 16
| (getcard8 (offset + 2)) << 8
| (getcard8 (offset + 3));
}
/** Return the Offset Size at offset
.
*/
protected int getOffSize (int offset) throws InvalidFontException {
return getcard8 (offset);
}
/** Return the Offset at offset
.
* @param exceptionMsg the details for UnsupportedFontException
,
* if thrown
* @throws UnsupportedFontException if the value cannot be represented
* as an int.
* @throws InvalidFontException if the offset is not valid for the font.
*/
protected int getOffset (int offset, int offSize, String exceptionMsg)
throws UnsupportedFontException, InvalidFontException {
switch (offSize) {
case 1: {
return getcard8 (offset); }
case 2: {
return getcard16 (offset); }
case 3: {
return getcard24 (offset); }
default: {
if (getcard8 (offset) > 0x7f) {
throw new UnsupportedFontException (exceptionMsg); }
return (int) getcard32 (offset); }}
}
static final class CFFByteArrayBuilder extends FontByteArray.FontByteArrayBuilder {
protected CFFByteArrayBuilder () {
super(new CFFByteArray(1024));
}
protected CFFByteArrayBuilder (int size) {
super(new CFFByteArray(size));
}
/** Add bytes to represent n
as a Card32 at the end of the array. */
protected void addCard32 (int n) {
appendRawByte ((byte)((n >> 24) & 0xff));
appendRawByte ((byte)((n >> 16) & 0xff));
appendRawByte ((byte)((n >> 8) & 0xff));
appendRawByte ((byte)((n ) & 0xff));
}
/** Add bytes to represent n
as a Card24 at the end of the array. */
protected void addCard24 (int n) {
appendRawByte ((byte)((n >> 16) & 0xff));
appendRawByte ((byte)((n >> 8) & 0xff));
appendRawByte ((byte)((n ) & 0xff));
}
/** Add bytes to represent n
as a Card16 at the end of the array. */
protected void addCard16 (int n) {
appendRawByte ((byte)((n >> 8) & 0xff));
appendRawByte ((byte)((n ) & 0xff));
}
/** Add bytes to represent n
as a Card8 at the end of the array. */
protected void addCard8 (int n) {
appendRawByte ((byte)((n ) & 0xff));
}
protected void addOffset(int offsetSize, int offset) {
if (offsetSize == 1) {
addCard8 (offset); }
else if (offsetSize == 2) {
addCard16 (offset); }
else if (offsetSize == 3) {
addCard24 (offset); }
else {
addCard32 (offset); }
}
/** Replace the four bytes at pos
with Card32 representation of n
. */
protected void setCard32 (int pos, int n) {
setRawByte (pos++, (byte)((n >> 24) & 0xff));
setRawByte (pos++, (byte)((n >> 16) & 0xff));
setRawByte (pos++, (byte)((n >> 8) & 0xff));
setRawByte (pos++, (byte)((n ) & 0xff));
}
/** Add the count
bytes starting at offset
in
* b
at the end of the array.
*/
protected void addBytes (byte[] b, int offset, int count) {
append (b, offset, count);
}
/** Add the count
bytes starting at offset
in
* ba
at the end of the array.
*/
protected void addBytes (CFFByteArray ba, int offset, int count)
throws InvalidFontException {
append (ba, offset, count);
}
protected CFFByteArray toCFFByteArray () {
CFFByteArray retvalue = (CFFByteArray) this.byteArray;
this.byteArray = null; // kill ourselves
return retvalue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy