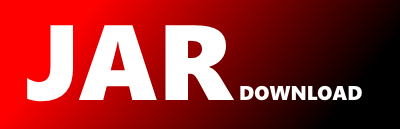
com.adobe.fontengine.font.cff.CIDComponentFont Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: CIDComponentFont.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import java.util.List;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.Matrix;
import com.adobe.fontengine.font.OutlineConsumer;
import com.adobe.fontengine.font.UnsupportedFontException;
/** A component in a CID-keyed font.
*/
final class CIDComponentFont {
/** Our component Dict
. */
protected final Dict fontDict;
/** Our private Dict
. */
protected final Dict privateDict;
/** Our local subroutines. */
protected final CharStrings localSubrs;
/** Construct a CIDComponentFont
.
* @param data the CFF structure
* @param offset the offset of this component's dict in data
* @param size the size of this component's dict in data
* @param stringIndex the StringIndex in which to resolve SIDs.
*/
public CIDComponentFont (CFFByteArray data, int offset, int size,
StringIndex stringIndex)
throws InvalidFontException, UnsupportedFontException {
fontDict = new Dict (data, offset, size, stringIndex);
Dict.OffsetSizeValue v2 = fontDict.get (Dict.Key.Private, false);
if (v2 == null) {
throw new InvalidFontException ("Missing privateDict in CID component font"); }
privateDict = new Dict (data, v2.offset, v2.size, stringIndex);
Dict.OffsetValue o3 = privateDict.get (Dict.Key.Subrs, false);
if (o3 != null) {
localSubrs = new CharStrings (data, v2.offset + o3.offset); }
else {
localSubrs = null; }
}
/** Construct a CIDComponentFont
.
* @param fontDict this component's dict
* @param privateDict this component's private dict
* @param localSubrs this component's local subroutines
*/
public CIDComponentFont (Dict fontDict,
Dict privateDict,
CharStrings localSubrs) {
this.fontDict = fontDict;
this.privateDict = privateDict;
this.localSubrs = localSubrs;
}
/** Get the outline of glyph gid
, using a specified parser.
* @param gid the glyph id of the glyph
* @param parser the Type2OutlineParser parser to use
* @param consumer the OutlineConsumer to receive the outline
* @param m the top dictionary font matrix for the cid font
*/
/** Return the outline of glyph gid
.
*/
public void getOutline (CharStrings charStrings, int gid,
CharStrings globalSubrs,
Type2OutlineParser parser,
OutlineConsumer consumer,
Dict.NumbersValue topMatrix)
throws InvalidFontException, UnsupportedFontException {
parser.parse (charStrings, gid, localSubrs, globalSubrs, consumer,
getOutlineMatrix (topMatrix), null);
}
public double getStemV() {
Dict.NumbersValue value = privateDict.get(Dict.NumbersKey.StdVW, false);
if (value != null) {
return value.getFirstValueAsDouble(); }
value = privateDict.get(Dict.NumbersKey.StemSnapV, false);
if (value!=null) {
return value.getFirstValueAsDouble(); }
return 0;
}
/**
* Combine the top dict matrix and this component matrix.
* @param topMatrix the top dictionary's matrix for the whole cid font.
*/
Matrix getOutlineMatrix (Dict.NumbersValue topMatrix) {
Dict.NumbersValue componentMatrix = fontDict.get (Dict.Key.FontMatrix, false);
if (topMatrix == null && componentMatrix == null) {
return new Matrix (fontDict.get (Dict.Key.FontMatrix, true).getValuesAsDouble ()); }
else if (topMatrix == null) {
return new Matrix (componentMatrix.getValuesAsDouble ()); }
else if (componentMatrix == null) {
return new Matrix (topMatrix.getValuesAsDouble ()); }
else {
Matrix m1 = new Matrix (componentMatrix.getValuesAsDouble ());
return m1.multiply (new Matrix (topMatrix.getValuesAsDouble ())); }
}
static class WidthConsumer extends Type2ConsumerDefaultImpl {
public double width;
public boolean widthSeen = false;
public boolean width (double w) {
widthSeen = true;
width = w;
return false; // we had enough
}
}
public double getHorizontalAdvance (CharStrings charStrings, int gid,
CharStrings globalSubrs)
throws InvalidFontException, UnsupportedFontException {
Type2Parser parser = new Type2Parser ();
WidthConsumer consumer = new WidthConsumer ();
parser.parse (charStrings, gid, localSubrs, globalSubrs, consumer, null);
if (consumer.widthSeen) {
Dict.NumbersValue v = privateDict.get (Dict.Key.nominalWidthX, true);
return v.getFirstValueAsDouble () + consumer.width; }
else {
Dict.NumbersValue v = privateDict.get (Dict.Key.defaultWidthX, true);
return v.getFirstValueAsDouble (); }
}
/** Collect the strings used by this component.
* @param strings a list in which unique strings are collected. */
public void collectStrings (List /**/ strings) {
fontDict.collectStrings (strings);
privateDict.collectStrings (strings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy