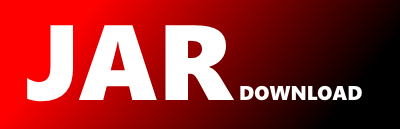
com.adobe.fontengine.font.cff.Encoding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: Encoding.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*/
package com.adobe.fontengine.font.cff;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
/** Represents an encoding.
*/
public final class Encoding {
/* Our strategy is to just keep our thumb on the underlying bytes,
* and to interpret them when needed. */
/** The container for our bytes, if the encoding is not predefined.
* If the encoding is predefined, than data
is null
. */
public final CFFByteArray data;
/** The offset of our bytes in data
if the encoding is
* not predefined.
* If the encoding is predefined, than data
is null
* and offset
is 0 or 1.
* Note that the value 0 is ambiguous, as it can be either the isoAdobe
* predefined charset (if data is null) or an offset in data (if data is
* not null). */
public final int offset;
/** Construct an Encoding
from a CFFByteArray
.
* offset
is interpreted to handle the predefined encodings;
* in other words, clients do not have to worry about those.
*
* @param data the CFFByteArray to get data from, if offset
is
* not 0 or 1
* @param offset if 0, this is the standard predefined encoding; if 1, this
* the expert predefined encoding; otherwise, the bytes of data
starting at
* offset
are used.
*/
Encoding (CFFByteArray data, int offset) throws InvalidFontException {
if (offset < 2) {
this.data = null; }
else {
this.data = data; }
this.offset = offset;
}
/** Return the gid for a character code.
*
* @param charCode the character code to lookup.
* @return the gid
for that character code
* (can be 0 for .notdef)
*/
public int charCode2gid (int charCode, Charset charset)
throws InvalidFontException, UnsupportedFontException {
if (data == null) {
if (offset == 0) {
return charset.sid2gid (standardEncoding [charCode]); }
if (offset == 1) {
return charset.sid2gid (expertEncoding [charCode]); }
throw new InvalidFontException ("invalid charset offset (" + offset + ")"); }
int o = offset;
int format = data.getcard8 (o);
o++;
boolean supplementalData = (format & 0x80) != 0;
format = format & 0x7f;
int currentGid = 1;
switch (format) {
case 0: {
int nEncodedGlyphs = data.getcard8 (o++);
for (; currentGid <= nEncodedGlyphs; currentGid++) {
int currentCharCode = data.getcard8 (o++);
if (currentCharCode == charCode) {
return currentGid; }}
break; }
case 1: {
int nRanges = data.getcard8 (o++);
// invariant:
// - there are nRanges left to consider
// - if there is at least one range, then o points to it and currentGid [[not currentCode]] is the first glyph encoded by that range
while (nRanges > 0) {
int currentCharCode = data.getcard8 (o++);
int nLeft = data.getcard8 (o++);
if (currentCharCode <= charCode && charCode <= currentCharCode + nLeft) {
return currentGid + (charCode - currentCharCode); }
currentGid += nLeft + 1;
nRanges--;}
break; }
default: {
throw new UnsupportedFontException ("CFF charset in format " + format); }}
if (supplementalData) {
int nSupplements = data.getcard8 (o++);
for (int i = 0; i < nSupplements; i++) {
int currentCharCode = data.getcard8 (o++);
if (currentCharCode == charCode) {
return charset.sid2gid (data.getcard16 (o)); }
o += 2; }}
return 0;
}
/** The predefined standard encoding. */
private static final int[] standardEncoding = {
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63,
64,
65,
66,
67,
68,
69,
70,
71,
72,
73,
74,
75,
76,
77,
78,
79,
80,
81,
82,
83,
84,
85,
86,
87,
88,
89,
90,
91,
92,
93,
94,
95,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
96,
97,
98,
99,
100,
101,
102,
103,
104,
105,
106,
107,
108,
109,
110,
0,
111,
112,
113,
114,
0,
115,
116,
117,
118,
119,
120,
121,
122,
0,
123,
0,
124,
125,
126,
127,
128,
129,
130,
131,
0,
132,
133,
0,
134,
135,
136,
137,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
138,
0,
139,
0,
0,
0,
0,
140,
141,
142,
143,
0,
0,
0,
0,
0,
144,
0,
0,
0,
145,
0,
0,
146,
147,
148,
149,
0,
0,
0,
0};
private static final int[] expertEncoding = {
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
1,
229,
230,
0,
231,
232,
233,
234,
235,
236,
237,
238,
13,
14,
15,
99,
239,
240,
241,
242,
243,
244,
245,
246,
247,
248,
27,
28,
249,
250,
251,
252,
0,
253,
254,
255,
256,
257,
0,
0,
0,
258,
0,
0,
259,
260,
261,
262,
0,
0,
263,
264,
265,
0,
266,
109,
110,
267,
268,
269,
0,
270,
271,
272,
273,
274,
275,
276,
277,
278,
279,
280,
281,
282,
283,
284,
285,
286,
287,
288,
289,
290,
291,
292,
293,
294,
295,
296,
297,
298,
299,
300,
301,
302,
303,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
304,
305,
306,
0,
0,
307,
308,
309,
310,
311,
0,
312,
0,
0,
313,
0,
0,
314,
315,
0,
0,
316,
317,
318,
0,
0,
0,
158,
155,
163,
319,
320,
321,
322,
323,
324,
325,
0,
0,
326,
150,
164,
169,
327,
328,
329,
330,
331,
332,
333,
334,
335,
336,
337,
338,
339,
340,
341,
342,
343,
344,
345,
346,
347,
348,
349,
350,
351,
352,
353,
354,
355,
356,
357,
358,
359,
360,
361,
362,
363,
364,
365,
366,
367,
368,
369,
370,
371,
372,
373,
374,
375,
376,
377,
378};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy