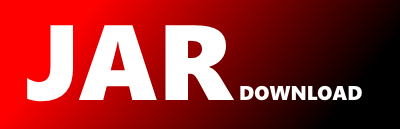
com.adobe.fontengine.font.cff.SyntheticFont Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* File: SyntheticFont.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import java.io.OutputStream;
import com.adobe.fontengine.font.CodePage;
import com.adobe.fontengine.font.Font;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.LineMetrics;
import com.adobe.fontengine.font.Matrix;
import com.adobe.fontengine.font.OrigFontType;
import com.adobe.fontengine.font.OutlineConsumer;
import com.adobe.fontengine.font.PDFFontDescription;
import com.adobe.fontengine.font.Permission;
import com.adobe.fontengine.font.ROS;
import com.adobe.fontengine.font.Rect;
import com.adobe.fontengine.font.Subset;
import com.adobe.fontengine.font.SubsetSimpleTrueType;
import com.adobe.fontengine.font.SubsetSimpleType1;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.font.XDCFontDescription;
/* Represents a synthetic font.
*/
final public class SyntheticFont extends CFFFont {
/** The index in fontSet
of our base font. */
protected final CFFFont base;
private final XDCFontDescription xdcDescription;
/** Create a subset for this font. */
public Subset createSubset() throws UnsupportedFontException {
throw new UnsupportedFontException("createSubset of CFF Synthetic fonts");
}
/** Construct a SyntheticFont
.
* @param stringIndex the StringIndex for this font
* @param base the base font of this font
* @param name the name of this font
* @param topDict the top Dict
of this font.
* @param data the underlying bytes from which the structures pointed
* by the top dict will be parsed
* @param digest the digest for the container of this font
*/
SyntheticFont (StringIndex stringIndex, CFFFont base, String name,
Dict topDict, CFFByteArray data, byte[] digest)
throws UnsupportedFontException, InvalidFontException {
super (stringIndex, null, topDict, name, digest);
xdcDescription = new SyntheticFontXDCFontDescription();
this.base = base;
}
//--------------------------------------------------------- general access ---
public int getNumGlyphs ()
throws UnsupportedFontException, InvalidFontException {
return base.getNumGlyphs ();
}
/** Return the name of glyph gid
.
*/
public String getGlyphName (int gid)
throws InvalidFontException, UnsupportedFontException {
return base.getGlyphName (gid);
}
public ROS getROS () {
return base.getROS();
}
public int getGlyphCid (int glyphID)
throws InvalidFontException, UnsupportedFontException {
return base.getGlyphCid(glyphID);
}
int[] getXUID () {
return base.getXUID();
}
String getNotice () {
return base.getNotice();
}
Integer getFSType () {
return base.getFSType();
}
OrigFontType getOrigFontType () {
return base.getOrigFontType();
}
/** Get the outline of glyph gid
.
* @param gid the glyph id of the glyph
* @param consumer the OutlineConsumer to receive the outline
*/
public void getGlyphOutline (int gid, OutlineConsumer consumer)
throws InvalidFontException, UnsupportedFontException {
base.getGlyphOutline (gid, consumer);
}
/** Get the outline of glyph gid
, using a specified parser.
* @param gid the glyph id of the glyph
* @param parser the Type2OutlineParser parser to use
* @param consumer the OutlineConsumer to receive the outline
*/
/** Return the outline of glyph gid
.
*/
public void getOutline (int gid, Type2OutlineParser parser, OutlineConsumer consumer)
throws InvalidFontException, UnsupportedFontException {
base.getOutline (gid, parser, consumer);
}
public double getStemVForGlyph(int gid) throws InvalidFontException {
return base.getStemVForGlyph(gid);
}
/** {@inheritDoc} */
public Matrix getFontMatrix () {
Matrix m = new Matrix (topDict.get (Dict.Key.FontMatrix, true).getValuesAsDouble ());
return base.getFontMatrix ().multiply (m);
}
/** {@inheritDoc} */
public double getHorizontalAdvance (int gid)
throws InvalidFontException, UnsupportedFontException {
return base.getHorizontalAdvance (gid);
}
double getItalicAngle () {
return base.getItalicAngle();
}
Rect getRawFontBBox () {
// we don't need to apply this font's matrix, as it is integrated
// in the result of getFontMatrix
return base.getRawFontBBox ();
}
// This matrix allows us to convert point in the font space to the
// metric space.
private Matrix getFontToMetricsMatrix () throws InvalidFontException, UnsupportedFontException {
Matrix m = getFontMatrix ();
double x = getUnitsPerEmX ();
double y = getUnitsPerEmY ();
return new Matrix (x * m.a, y * m.b, x * m.c, y * m.d, x * m.tx, y * m.ty);
}
public Rect getFontBBox () throws InvalidFontException, UnsupportedFontException {
Rect rawBBox = getRawFontBBox ();
if (rawBBox == null) {
return null; }
return rawBBox.applyMatrix (getFontToMetricsMatrix ());
}
public Rect getCoolTypeRawFontBBox ()
throws InvalidFontException, UnsupportedFontException {
return getFontBBox ();
}
//------------------------------------------------------------------- cmap ---
public int getGlyphForChar (int usv)
throws InvalidFontException, UnsupportedFontException {
return base.getGlyphForChar (usv);
}
double getDefaultWidthForFD (int fd) {
return base.getDefaultWidthForFD(fd);
}
double getNominalWidthForFD (int fd) {
return base.getNominalWidthForFD(fd);
}
int getFDForGlyph (int fullGid) throws InvalidFontException {
return base.getFDForGlyph(fullGid);
}
CharStrings getLocalSubrsForFD (int fd) {
return base.getLocalSubrsForFD(fd);
}
int getNumFDs () {
return base.getNumFDs();
}
CharStrings getCharStrings () {
return base.getCharStrings();
}
//----------------------------------------------- line metrics computation ---
/** Emulates the CoolType API CTFontDict:GetHorizontalMetrics CoolType API.
*
* The metrics are expressed in the design space of the font,
* i.e. they need to be converted through the metrics matrix.
*
*
This methods never returns null.
*
*
See also the {@link #getLineMetrics()} method.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
*/
public LineMetrics getCoolTypeLineMetrics ()
throws UnsupportedFontException, InvalidFontException {
return base.getCoolTypeLineMetrics ();
}
public Rect getCoolTypeIdeoEmBox ()
throws UnsupportedFontException, InvalidFontException {
return base.getCoolTypeIdeoEmBox ();
}
public Rect getCoolTypeIcfBox ()
throws UnsupportedFontException, InvalidFontException {
return base.getCoolTypeIcfBox ();
}
//----------------------------------------------- subsetting and streaming ---
public Permission getEmbeddingPermission(boolean wasEmbedded)
throws InvalidFontException, UnsupportedFontException {
return base.getEmbeddingPermission(wasEmbedded);
}
/** Subset and stream this font for PDF use.
* The stream is a CID-keyed CFF stream.
* @param out the OutputStream to which the bytes are streamed
*/
public void subsetAndStream (Subset subset, OutputStream out, boolean preserveROS, Integer fsType)
throws InvalidFontException, UnsupportedFontException {
throw new UnsupportedFontException ("subsetAndStream of CFF Synthetic fonts");
}
String getCopyright() {
return base.getCopyright();
}
String getFullName() {
return base.getFullName();
}
int getFirstChar() throws InvalidFontException, UnsupportedFontException {
return base.getFirstChar();
}
int getLastChar() throws InvalidFontException, UnsupportedFontException {
return base.getLastChar();
}
private class SyntheticFontXDCFontDescription extends CFFFontXDCFontDescription {
public int getCIDCount()
{
return -1;
}
public void subsetAndStream(Subset subset, OutputStream out, boolean preserveROS)
throws UnsupportedFontException
{
throw new UnsupportedFontException("subsetAndStream of CFF Synthetic fonts");
}
public void subsetAndStream(SubsetSimpleType1 t1Subset, OutputStream out)
throws UnsupportedFontException
{
throw new UnsupportedFontException("subsetAndStream of CFF Synthetic fonts");
}
public void subsetAndStream(SubsetSimpleTrueType ttSubset, OutputStream out)
throws UnsupportedFontException
{
throw new UnsupportedFontException("subsetAndStream of CFF Synthetic fonts");
}
public CodePage[] getXDCCodePages()
throws InvalidFontException, UnsupportedFontException
{
return base.getXDCFontDescription(null).getXDCCodePages();
}
public void stream(OutputStream out, boolean openTypeFontsAllowed) throws UnsupportedFontException {
throw new UnsupportedFontException("stream of CFF Synthetic fonts");
}
}
public PDFFontDescription getPDFFontDescription(Font font) {
return xdcDescription;
}
public XDCFontDescription getXDCFontDescription(Font font) {
return xdcDescription;
}
public void stream(OutputStream out, Integer fsType) throws UnsupportedFontException {
throw new UnsupportedFontException("stream of CFF Synthetic fonts");
}
}