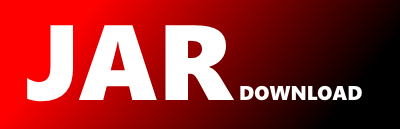
com.adobe.fontengine.font.postscript.CIDtoUnicode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: CIDtoUnicode.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.postscript;
import java.io.Serializable;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.util.MissingResourceException;
import com.adobe.fontengine.font.UnsupportedFontException;
final public class CIDtoUnicode implements Serializable {
/* Serialization signature is explicitly set and should be
* incremented on each release to prevent compatibility.
*/
static final long serialVersionUID = 1;
String registry;
String ordering;
int [] map;
public CIDtoUnicode (String registry, String ordering, int[] map) {
this.registry = registry;
this.ordering = ordering;
this.map = new int [map.length];
System.arraycopy (map, 0, this.map, 0, map.length);
}
public int cid2usv (int cid) {
// It is possible that our map do not contain entries for all CIDs, e.g.
// if a font is built for a later supplement than the supplement of
// the CMap from which we started. Another case is Adobe-GB1-4:
// the last 5 CIDs are rotated glyphs, and are not mapped by the
// Unicode CMap
if (cid >= map.length) {
return -1; }
else {
return map [cid]; }
}
//------------------------------------------------------- deserialization ---
static CIDtoUnicode get (String registry, String ordering)
throws MissingResourceException, UnsupportedFontException {
try {
InputStream is = CIDtoUnicode.class.getResourceAsStream ("cid2usv_" + registry + "_" + ordering);
if (is == null)
throw new UnsupportedFontException("Cannot convert from " + registry + "-" + ordering + " to Unicode");
ObjectInputStream ois = new ObjectInputStream (is);
try {
return (CIDtoUnicode) ois.readObject (); }
finally {
ois.close (); }}
catch (IOException e) {
MissingResourceException ee = new MissingResourceException ("", "", "");
ee.initCause (e);
throw ee; }
catch (ClassNotFoundException e) {
MissingResourceException ee = new MissingResourceException ("", "", "");
ee.initCause (e);
throw ee; }
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy