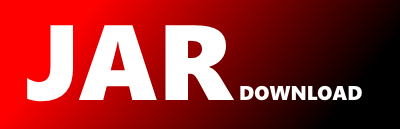
com.adobe.fontengine.font.postscript.PostscriptTokenParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: PostscriptTokenParser.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.postscript;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.adobe.fontengine.font.OrigFontType;
final public class PostscriptTokenParser {
private static final Pattern origFontTypePattern
= Pattern.compile("/OrigFontType\\s*/((?:" + OrigFontType.kTYPE1.toString() + ")|(?:"
+ OrigFontType.kTRUETYPE.toString() + ")|(?:"
+ OrigFontType.kOCF.toString() + ")|(?:"
+ OrigFontType.kCID.toString() + "))(\\s+def)", 0);
private static final Pattern fsTypePattern = Pattern.compile("/FSType\\s*(-?\\d+)(\\s+def)", 0);
public static Integer getFSType(String v)
{
if (v != null)
{
Matcher m = fsTypePattern.matcher(v);
if (m.find())
{
int startPos = m.start(1);
int endPos = m.start(2);
return new Integer(Integer.parseInt(v.substring(startPos, endPos)));
}
}
return null;
}
public static OrigFontType getOrigFontType(String v)
{
if (v != null)
{
Matcher m = origFontTypePattern.matcher(v);
if (m.find())
{
int startPos = m.start(1);
int endPos = m.start(2);
String s = v.substring(startPos, endPos);
if (s.equals(OrigFontType.kTYPE1.toString()))
return OrigFontType.kTYPE1;
else if (s.equals(OrigFontType.kTRUETYPE.toString()))
return OrigFontType.kTRUETYPE;
else if (s.equals(OrigFontType.kCID.toString()))
return OrigFontType.kCID;
else if (s.equals(OrigFontType.kOCF.toString()))
return OrigFontType.kOCF;
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy