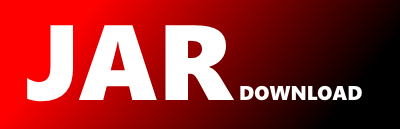
com.adobe.fontengine.fontmanagement.Base14Font Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: Base14Font.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.fontmanagement;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.lang.ref.SoftReference;
import java.util.HashMap;
import java.util.Iterator;
import com.adobe.fontengine.font.Base14;
import com.adobe.fontengine.font.FontData;
import com.adobe.fontengine.font.FontInputStream;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.font.opentype.FontFactory;
final public class Base14Font extends URLFont {
static final long serialVersionUID = 1;
private final String mBase14CSSName;
private final String mBase14PSName;
private final static HashMap> mBase14DescCache;
static {
/**
* Deserialize the cache for the builtin fonts. This MUST be allowed to fail silently because the
* static initializer is also invoked during the resource building, at which time the builtin cache
* does not exist yet.
*/
InputStream inStm = null;
ObjectInputStream objInStm = null;
HashMap> base14DescCache = null;
try {
inStm = Base14.class.getResourceAsStream("Base14Cache");
objInStm = new ObjectInputStream(inStm);
try {
base14DescCache = (HashMap>)objInStm.readObject();
} catch (ClassNotFoundException e) {
}
} catch (Exception e) {
} finally {
try {
if (objInStm != null) {
objInStm.close();
} else if (inStm != null) {
inStm.close();
}
} catch (Exception e) {
}
}
mBase14DescCache = base14DescCache;
}
public Base14Font(String resourceName, String base14CSSName, String base14PSName) {
super(Base14.class.getResource(resourceName), 0);
mBase14CSSName = base14CSSName;
mBase14PSName = base14PSName;
}
protected synchronized FontData retrieveFontData()
throws UnsupportedFontException, InvalidFontException, FontLoadingException
{
FontData font = null;
try {
if ((font = (FontData)fontRef.get()) == null) {
FontInputStream stream = new FontInputStream(outlineFileURL.openStream());
try {
FontData[] arr = FontFactory.load(stream, mBase14CSSName, mBase14PSName);
font = arr[index];
fontRef = new SoftReference(font);
} finally {
stream.close();
}
}
} catch (IOException e) {
throw new FontLoadingException(e);
}
return font;
}
/**
* Special case cache manipulation support for Base14 fonts. Since this cache is built into the
* resources it is not treated the same way as other types. Basically, everything except
* getCachedFontDescription is a noop and getCachedFontDescription simply reads an item from
* the builtin cache. Cache entries are never verified nor are new cached FontDescriptions added
* at runtime. Any missing descriptions MUST be added to the Base14CacheResourceBuilder.
*/
public String getCanonicalPath()
{
return null;
}
public long getLength()
{
return 0;
}
public long getLastModified()
{
return 0;
}
public Object getCachedFontDescription(String key)
{
if (mBase14DescCache == null)
return null;
HashMap descMap = mBase14DescCache.get(mBase14PSName);
if (descMap == null)
return null;
return descMap.get(key);
}
public Iterator getCachedFontDescriptionIterator()
{
return null;
}
public void setCachedFontDescription(String key, Object value)
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy