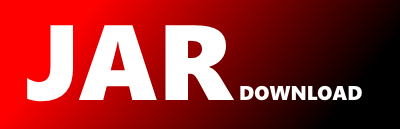
com.adobe.fontengine.fontmanagement.fxg.FXGFontDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: PostscriptFontDescription.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.fontmanagement.fxg;
import com.adobe.agl.util.ULocale;
import com.adobe.fontengine.font.FontDescription;
import com.adobe.fontengine.fontmanagement.Platform;
/**
* An FXG description of a font. This description can be used for looking up fonts.
*/
final public class FXGFontDescription extends FontDescription
{
static final long serialVersionUID = 1;
private final Platform platform;
private final ULocale locale;
private final String familyName;
private final boolean isBold;
private final boolean isItalic;
/**
* Constructor
* @param platform the platform that this description is for
* @param familyName the FXG font family name
* @param isBold true
if the font is bold; false
otherwise
* @param isItalic true
if the font is italic; false
otherwise
*/
public FXGFontDescription(Platform platform, ULocale locale,
String familyName, boolean isBold, boolean isItalic)
{
if (platform == null || locale == null || familyName == null)
{
throw new NullPointerException("Platform, Locale, and Platform Name must not be null");
}
this.platform = platform;
this.locale = locale;
this.familyName = familyName;
this.isBold = isBold;
this.isItalic = isItalic;
}
/**
* Get the platform that this description is for.
* @return the platform that this description is for
*/
public Platform getPlatform()
{
return this.platform;
}
/**
* Get the locale that this description is for.
* @return the locale that this description is for
*/
public ULocale getLocale()
{
return this.locale;
}
/**
* Get the font family name used for FXG.
* @return the font family name
*/
public String getFamilyName()
{
return familyName;
}
/**
* Tests whether the font is bold in FXG terms.
* @return true
if the font is bold; false
otherwise
*/
public boolean isBold()
{
return isBold;
}
/**
* Tests whether the font is italic in FXG terms.
* @return true
if the font is italic; false
otherwise
*/
public boolean isItalic()
{
return isItalic;
}
public int hashCode()
{
final int prime = 31;
int result = 1;
result = prime * result + (isBold ? 1231 : 1237);
result = prime * result + (isItalic ? 1231 : 1237);
result = prime * result + locale.hashCode();
result = prime * result + platform.hashCode();
result = prime * result + familyName.hashCode();
return result;
}
public boolean equals(Object obj)
{
if (this == obj)
{
return true;
}
if (obj == null)
{
return false;
}
if (!(obj instanceof FXGFontDescription))
{
return false;
}
final FXGFontDescription other = (FXGFontDescription) obj;
if (isBold != other.isBold)
{
return false;
}
if (isItalic != other.isItalic)
return false;
if (locale == null)
{
if (other.locale != null)
{
return false;
}
} else if (!locale.equals(other.locale))
return false;
if (platform == null)
{
if (other.platform != null)
{
return false;
}
}
else if (!platform.equals(other.platform))
{
return false;
}
if (familyName == null)
{
if (other.familyName != null)
{
return false;
}
} else if (!familyName.equals(other.familyName))
{
return false;
}
return true;
}
public String toString()
{
return new String("[" + this.platform + ", " + this.locale + "] - " + this.familyName +
(this.isBold ? ", bold" : "") + (this.isItalic ? ", italic" : ""));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy