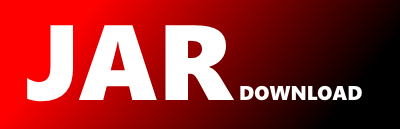
com.adobe.fontengine.inlineformatting.TypographicCase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: TypographicCase.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting;
/** Enumerated values for {@link ElementAttribute#typographicCase}.
*
* Glyphs have typographic case, which describe their shape and their spacing.
* This notion is distinct from the notion of character case (which
* is uppercase, lowercase, or caseless).
*/
final public class TypographicCase {
private final String name;
private TypographicCase (String name) {
this.name = name;
}
public String toString () {
return name;
}
/** TITLE.
* uppercase characters -> titling glyphs, close to uppercase glyphs
* lowercase characters -> lowercase glyphs
* others -> form for uppercase context
* spacing for titles
*/
public static final TypographicCase TITLE = new TypographicCase ("TITLE");
/** CAPS.
* uppercase characters -> uppercase glyphs
* lowercase characters -> lowecase glyphs
* others -> form for uppercase context
* spacing for uppercase text
*/
public static final TypographicCase CAPS = new TypographicCase ("CAPS");
/** CAPS_AND_SMALLCAPS.
* uppercase characters -> uppercase glyphs
* lowercase characters -> small cap glyphs
* others -> form for lowercase context
* spacing for lowercase text
*/
public static final TypographicCase CAPS_AND_SMALLCAPS = new TypographicCase ("CAPS_AND_SMALLCAPS");
/** SMALLCAPS.
* uppercase characters -> small cap glyphs
* lowercase characters -> small cap glyphs
* others -> form for lowercase context
* spacing for lowercase text
*/
public static final TypographicCase SMALLCAPS = new TypographicCase ("SMALLCAPS");
/** PETITECAPS.
* uppercase characters -> petite cap glyphs
* lowercase characters -> petite cap glyphs
* others -> form for lowercase text
* spacing for lowercase text
*/
public static final TypographicCase PETITECAPS = new TypographicCase ("PETITECAPS");
/** TEXT.
* uppercase characters -> uppercase glyphs
* lowercase characters -> lowercase glyphs
* others -> form for lowercase text
* spacing for lowercase text
*/
public static final TypographicCase TEXT = new TypographicCase ("TEXT");
/** UNICASE.
* uppercase characters -> unicase glyphs
* lowercase characters -> unicase glyphs
* others -> form for lowercase text
* spacing for lowercase text
*/
public static final TypographicCase UNICASE = new TypographicCase ("UNICASE");
/** NONE
* No transformation performed.
*/
public static final TypographicCase NONE = new TypographicCase ("NONE");
private static final TypographicCase[] allValues
= {TITLE, CAPS, CAPS_AND_SMALLCAPS, SMALLCAPS, PETITECAPS, TEXT, UNICASE, NONE};
public static TypographicCase parse (String s) {
for (int i = 0; i < allValues.length; i++) {
if (allValues [i].name.compareToIgnoreCase (s) == 0) {
return allValues [i]; }}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy